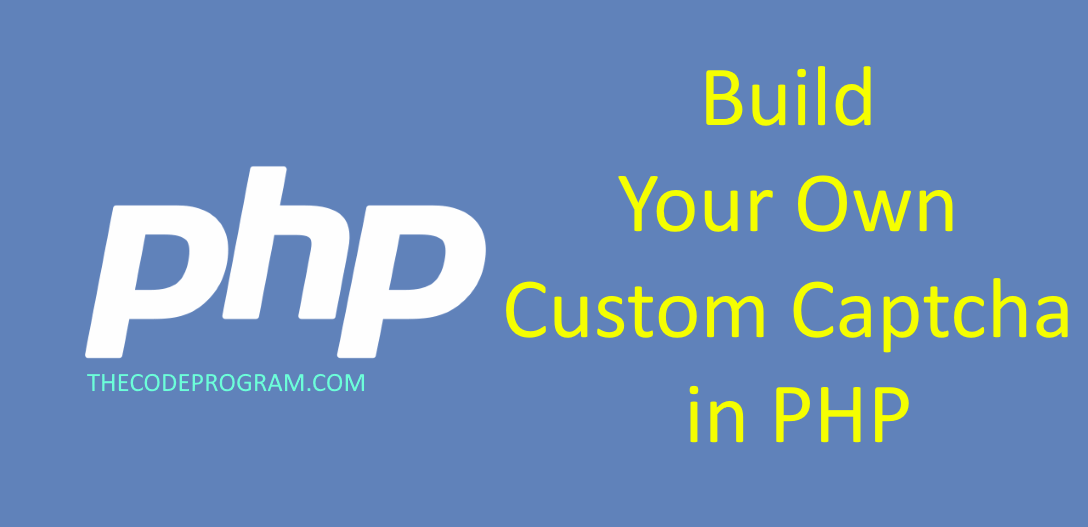
Build Your Own Custom Captcha in PHP
Hello everyone, in this article we are going to build a captcha in PHP language. With these captcha, we are going to protect our websites from the autobots. So many bots developing nowadays, we will stop them when they try to make a flooding to our website.Now we are ready to begin.
In here firstly we have create a captcha text to show to the user and after it we have to create an image to show created text.
Below code block will Create the captcha word. I want it 5 charactered captcha text with only numbers and capital letters. After it I will load this captcha word on SESSION. We will check it with SESSION value.
function create_captcha_word() {
$captcha_text = ""; //initialize the required variable to keep the data.
for($i=0; $i<5; $i++) //I want to create 5 charactered captcha word
{
//Make a random selection
switch(rand(1,2))
{
case 1: $captcha_text .=chr(rand(48,57)); break; //0-9
case 2: $captcha_text .=chr(rand(65,90)); break; //A-Z
//case 3: $captcha_text .=chr(rand(97,122)); break; //a-z
}
}
//load the created word to the SESSION
$_SESSION['captcha_string'] = $captcha_text ;
return $captcha_text;
}
Now our captcha word is ready. We should create an image and we have to write our word to the image. After we will return this related the image and create an img object then we will put this image in this. I want to create some confussion about the image to make solving difficult for the bot programs. So I will add some background lines and dots to the image with different colors.
Below code block you will see how to create image and write the text in it.
function create_captcha_image()
{
//Define an image to build.
$captcha_image= imagecreatetruecolor(250, 100) or die("GD could not initialized");
//Here we define the background, captcha, line and dot colors.
$back_color = imagecolorallocate($image, 255, 255, 255);
$dot_color = imagecolorallocate($image, 0, 0, 255);
$line_color = imagecolorallocate($image, 64, 64, 64);
//We fill our created image with the defined background color.
imagefilledrectangle($captcha_image, 0, 0, 250, 100, $back_color );
//Here we add some dots to the image
for ($i = 0; $i < 200; $i++) {
imagesetpixel($captcha_image, rand() % 250, rand() % 100, $dot_color);
}
//Here we add some lines to the image
for ($i = 0; $i < 1; $i++) {
imageline($captcha_image, 0, rand() % 100, 250, rand() % 100, $line_color);
}
return $captcha_image;
}
Our captcha word and captcha image are ready now. All we have to do now to load our word on the image. We will write char by char to the image.
Below code block you can see how to do it.
function load_text_to_image(){
$word = create_captcha_word();
global $captcha_image = create_captcha_image();
//We wrote all of letters inside the image
$text_color = imagecolorallocate($captcha_image , 0, 0, 0);
for ($i = 0; $i < 5; $i++) {
imagestring($captcha_image , 7, 55 + ($i * 30), 20, $word[$i], $text_color);
}
//clean the global image for refreshing
$images = glob("*.png");
foreach ($images as $image_to_delete) {
@unlink($image_to_delete);
}
imagepng($captcha_image , "image" . $_SESSION['time'] . ".png");
}
Our functions are ready now, here we have to show our enter captcha screen. Below code bloc will do it.
<?php
session_start();
$_SESSION['time'] = time();
?>
<body>
<?php
if ($_SESSION['captcha_word'] == ) {
if ($input == $_SESSION['captcha_word']) {
?>
<div>
<h2>correct</h2>
<form action=" <?php echo $_SERVER['PHP_SELF']; ?>" method="POST">
<input type="submit" value="refresh the page">
</form>
</div>
<?php
} else {
?>
<div style="text-align:center;">
<h1>incorrect!</h1>
</div>
<?php
load_text_to_image();
enter_captcha_screen();
}
} else {
load_text_to_image();
enter_captcha_screen();
}
function enter_captcha_screen()
{
?>
<div style="text-align:center;">
<h4>What do you see in the image</h4>
<div>
<img src="image<?php echo $_SESSION['time'] ?>.png">
</div>
<form action=" <?php echo $_SERVER['PHP_SELF']; ?>" method="POST" / >
<input name="captcha_word" type="text" />
<input type="submit" value="submit" />
</form>
<a href="<?php echo $_SERVER['PHP_SELF']; ?>"></a>
</div>
<?php
}
?>
Also you can reach the example PHP file from here: https://github.com/thecodeprogram/PHP_Captcha .
That is all in this article.
Have good protection you website.
Burak hamdi TUFAN
Comments