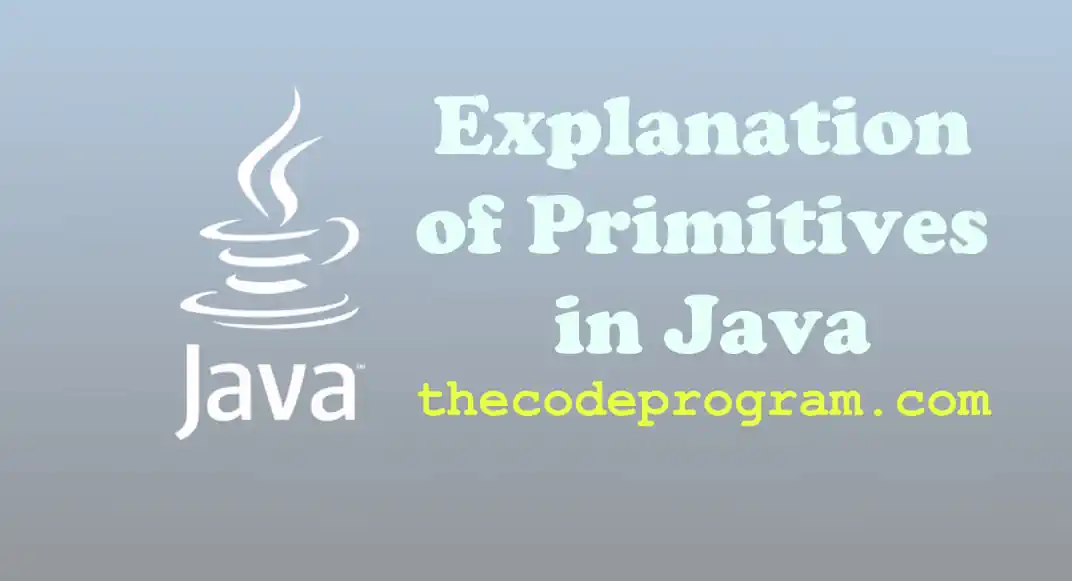
Explanation of Primitives in Java
Hello everyone, in this article we are going to talk about primitive data types in Java programming language with the help of the examples for better understanding.Let's get started
Java is widely using programming language which is known with its simplicity and versatility. In Java, we can see wide range data types and also including primitive types. In this article we will deep dive in primitive types. Primitive types are efficient when storing simple values instead of class definitions. So understanding primitive types will improve the efficiency because they are base of more complex data types.
What are primitive types?
Primitive types are using for storing values directly without creating instances of objects like numbers, chars, booleans. As they are not classes they have no methods internal properties and methods. They are just to store the data. In java primitive types are very important to optimise the performance and memory usage of the program by not storing unnecessary members of Wrapper classes of primitives.
-
Integer Types:
byte
: 8-bit signed integer (-128 to 127)short
: 16-bit signed integer (-32,768 to 32,767)int
: 32-bit signed integer (-2^31 to 2^31-1)long
: 64-bit signed integer (-2^63 to 2^63-1)
Floating-Point Types:
float
: 32-bit single-precision floating-point (approx. 7 decimal places)double
: 64-bit double-precision floating-point (approx. 15 decimal places)
Boolean Type:
boolean
: Represents true or false values
Character Type:
char
: 16-bit Unicode character (0 to 65,535)
Now let's see these types one by one for better understanding.
Integer Types
Below you can see the definitions of Integer types.
byte myByte = 100;
short myShort = 1000;
int myInt = 10000;
long myLong = 100000L; // The 'L' indicates it is a long number literally
System.out.println(myByte);
System.out.println(myShort);
System.out.println(myInt);
System.out.println(myLong);
Floating-Point Types
Below you can see the definitions of Floating point types in Java
float myFloat = 3.14F; // The 'F' indicates a float number literal
double myDouble = 3.14159265359;
System.out.println(myFloat);
System.out.println(myDouble);
Boolean Type
Below you can see the definitions of boolean primitives in Java
boolean isExist = true;
boolean isEasy = false;
System.out.println(isExist);
System.out.println(isEasy);
Character Type
Below you can see the definitions of char types in Java
char myChar = 'A';
char myUnicodeChar = '\u0194'; // Represents Gamma symbol (Ɣ)
System.out.println(myChar);
System.out.println(myUnicodeChar);
Type Conversion
When working with data types we need to be careful for type transforming. During the transforming data loss is a possibility if we do not consider the sizes of types. For example when we transform an integer to long we will not loss data but if we transform from double to integer, it might be a possibility to loss data.
// Below definition is fine,
int intValue = 42;
long longValue = intValue; integer variable size will be widen
// Below definition is not OK, data loss will be happen
float floatValue = 3.14F;
int intFromFloat = (int) floatValue; // Narrowing will be happen and data loss will be happen
Characteristics of Primitive Types
Let's talk about the Characteristics of primitive types in Java:Conclusion
Primitive types are very efficient way to store and process values directly. Primitive types are also consistant way to store the values in all platforms since they have default occuoancy sizes in memory. Understanding the primitive types in Java will improve efficiency of the program
That is all for now.
Burak Hamdi TUFAN
Comments