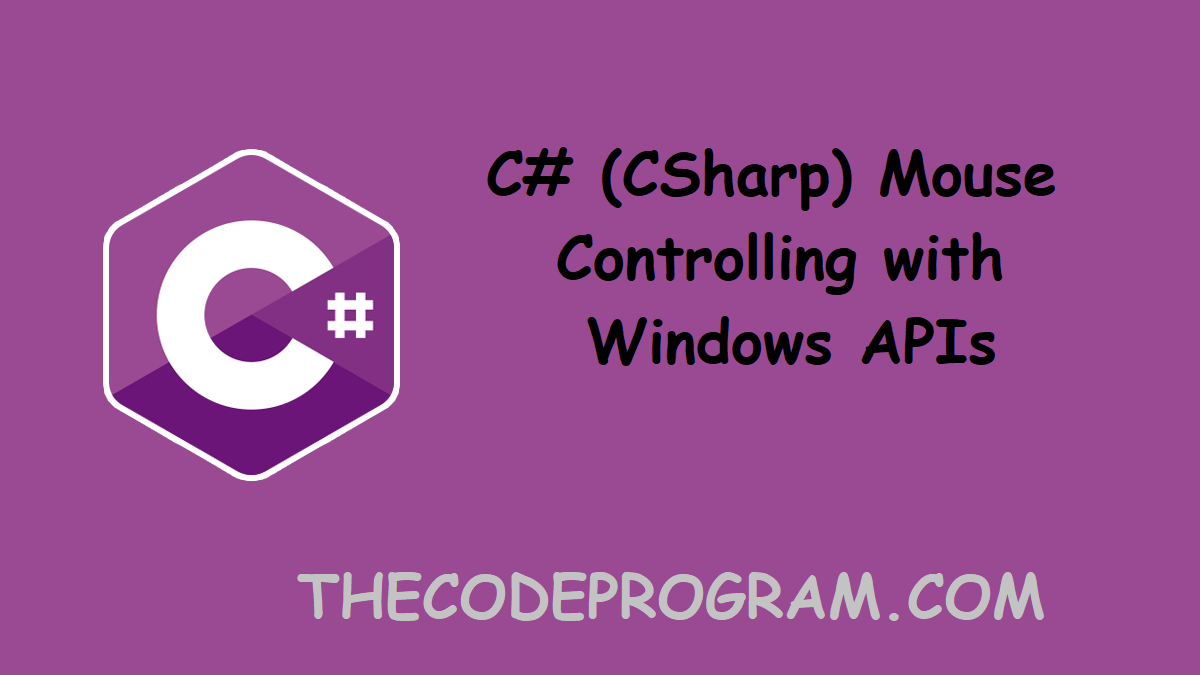
C# Mouse Controlling with Windows APIs
Hello everyone I will explain how to control the mouse from our program in this article with C#. After that we will bring the mouse to the point we want and click as many times as we want.We will use the Windows APIs for this process and send the ASCII Hex code to the system. Let's start now.
First we will use the System DLL files.
using System.Runtime.InteropServices;
We need to add the above library to the namespace.
And then we need to introduce the variables and DLL to the program.
[DllImport("user32.dll", CharSet = CharSet.Auto, CallingConvention = CallingConvention.StdCall)]
//We have integrated Windows API to use in our program
public static extern void mouse_control(Int32 dwFlags, Int32 dx, Int32 dy, Int32 cButtons, Int32 dwExtraInfo);
//We are going to move cursor with above method
private const int click_left= 0x02;
private const int unclick_left= 0x04;
private const int click_right= 0x08;
private const int unclick_right= 0x10;
//These are variables that we will send them into move function
//Purpose of these variables is prevent confusing due to hex codes. We have equalized the hex values to the variables.
Please note that we have identified the commands we will send from HexaDecimal. This is because the DLL understands ASCII code and is essentially ASCII code.
Now let's move our mouse and right-click ...
Below are the right-click mouse codes
private void SagTikla()
{
//X position of the cursor on screen
int mouse_x_location= Cursor.Position.X;
//Y position of the cursor on screen
int mouse_y_location= Cursor.Position.Y;
mouse_control(click_right| unclick_right, mouse_x_location, mouse_y_location, 0, 0);
}
Yes, as you can see from the above function, we give the necessary coordinates and the keys to be clicked.
Now let's look at the left click process ...
private void SolTikla()
{
//X position of the cursor on screen
int mouse_x_location= Cursor.Position.X;
//Y position of the cursor on screen
int mouse_y_location= Cursor.Position.Y;
mouse_control(click_left| unclick_left, mouse_x_location, mouse_y_location, 0, 0);
}
In this way, we can do some time-click operations while we are not at the computer. We can move the mouse on the screen and make transactions.
Have a Nice coding...
Burak Hamdi TUFAN
Comments