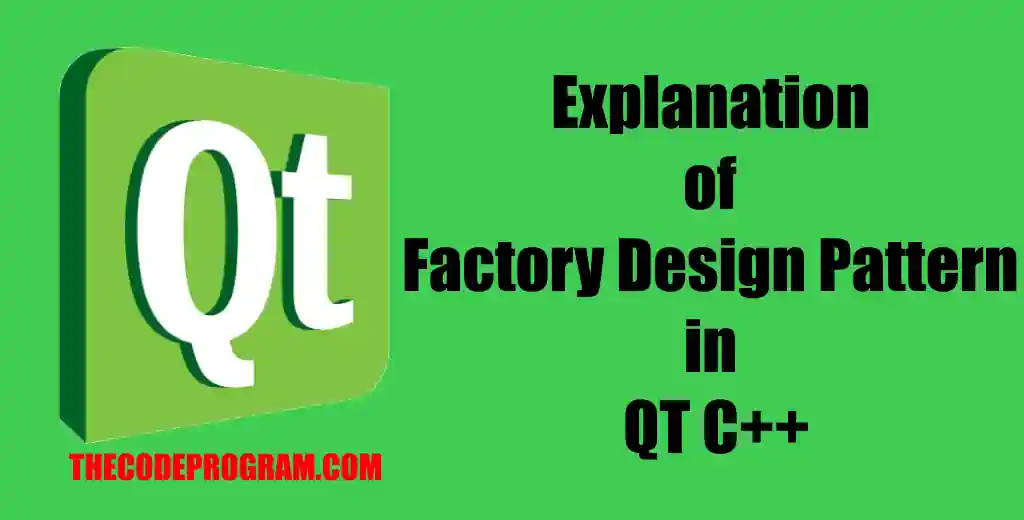
Explanation of Factory Design Pattern in QT C++
Hello everyone, in this article we are going to talk about Factory Design Pattern. We are going to make a simple example on factory design pattern with C++ and QT.Let's begin.
Firstly You can check this article out the understand Creational Design Patterns. In that article I explained all of them with C# .Net examples. But In here we are going to make almost same example with QT C++.
https://thecodeprogram.com/explanation-of-creational-design-patterns
https://thecodeprogram.com/explanation-of-creational-design-patterns
Now Let's start to talk on Factory Design Pattern.
According to above article we are not going to talk different thing and I will the same thing before I said :)
What is Factory Design Pattern
Factory type of creational design patterns provide us to create objects or components on a central location. Thanks to factory type creating objects is seperating from the main thread and it use the interfaces to create objects. We use the same interfaces for all similar types objects. For example we will create some kind of vehicles like car, truck, bicycle, aircraft... These are all vehicles as you can see.
First we create our classes which derived from same interface. And then we make callbacks from main thread to related class via interface.
Due to we derived all similar types of classes from one interface we use if/else if blocks so much to decide which method be calledback.
Due to we derived all similar types of classes from one interface we use if/else if blocks so much to decide which method be calledback.
Below code block you will see the interface class. In this class I have created the functions which will be called from derived classes.
ivehicle.h
#ifndef IVEHICLE_H
#define IVEHICLE_H
#include "iostream"
//First we need to create our interface class to derive other classes.
class IVehicle
{
public:
IVehicle() { }
~IVehicle(){ }
bool GetVehicleReady();
void StartVehicle();
void StopVehicle();
};
#endif // IVEHICLE_H
ivehicle.cpp
#include "ivehicle.h"
Now we will create the other classes which derived from above interface class.
car.h
#ifndef CAR_H
#define CAR_H
#include "ivehicle.h"
#include "iostream"
class Car : public IVehicle
{
public:
Car();
virtual ~Car(){ std::cout << "Destructor of Car" << std::endl << "--------------------------" << std::endl; }
bool GetVehicleReady(){std::cout << "Car is ready" << std::endl; return true; }
void StartVehicle(){ std::cout << "Car has been started" << std::endl; }
void StopVehicle(){ std::cout << "Car has been stopped" << std::endl; }
static IVehicle * __stdcall Initialize() { return new Car(); }
};
#endif // CAR_H
car.cpp
#include "car.h"
Car::Car()
{
StartVehicle();
GetVehicleReady();
StopVehicle();
}
truck.h
#ifndef TRUCK_H
#define TRUCK_H
#include "ivehicle.h"
#include "iostream"
class Truck : public IVehicle
{
public:
Truck();
virtual ~Truck(){ std::cout << "Destructor of Truck" << std::endl << "--------------------------" << std::endl; }
bool GetVehicleReady(){std::cout << "Truck is ready" << std::endl; return true; }
void StartVehicle(){ std::cout << "Truck has been started" << std::endl; }
void StopVehicle(){ std::cout << "Truck has been stopped" << std::endl; }
static IVehicle * __stdcall Initialize() { return new Truck(); }
};
#endif // TRUCK_H
truck.cpp
#include "truck.h"
Truck::Truck()
{
StartVehicle();
GetVehicleReady();
StopVehicle();
}
Above our classes are ready. We will call them in our main function and let them to print somethings on the screen.
main.cpp
#include <QCoreApplication>
#include "ivehicle.h"
#include "car.h"
#include "truck.h"
#include "iostream"
using namespace std;
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
system("title Factory Design Pattern Example - Thecodeprogram");
cout << "Welcome Factory Design Pattern Example in QT C++" << endl << endl;
IVehicle vehicle;
vehicle = Car();
vehicle = Truck();
return a.exec();
}
That is all in this article.
You can reach example project on Github via : https://github.com/thecodeprogram/FactoryDesignPattern_QT_CPP
I wish all of you healthy days.
Burak Hamdi TUFAN.
Tags
Share this Post
25/01/2016
Linux Mint Üzerine Android Studio Kurulumu
09/05/2021
C++ ile PIMPL IDIOM Nedir?
20/10/2021
Comments