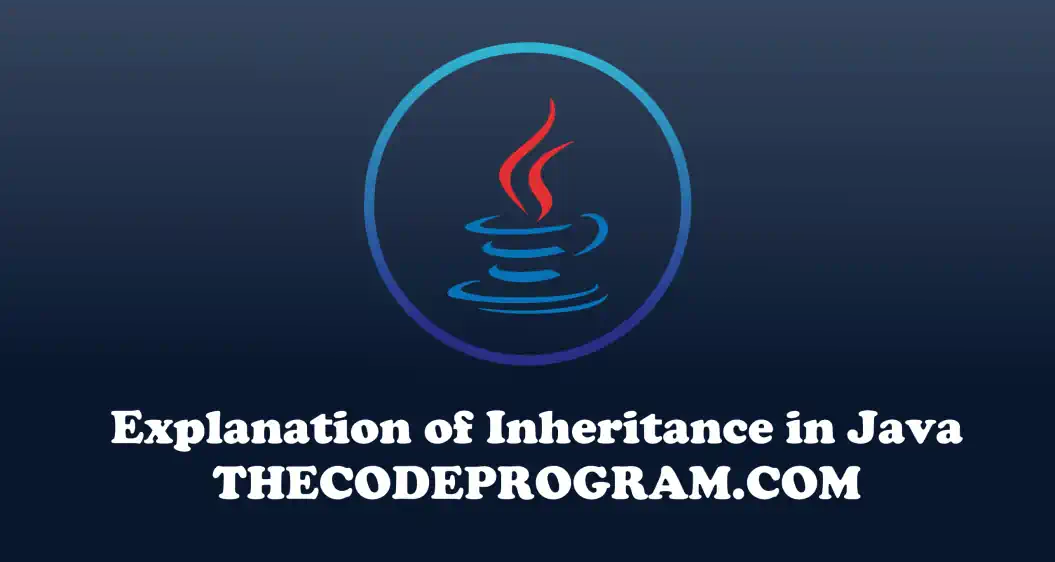
Explanation of Inheritance in Java
Hello everyone, in this article we are going to talk about Inheritence feature of Object Oriented Programming in Java and we will make some examples for better understanding.Let's get started.
Inheritence is one of the key concepts of object oriented programming. It allows us to create new classes using an existing class. We can derive new classes and these child classes can use properties of parent class. We can directly invoke the functions within parent class throgh child class.
Inheritance is increasing the code reusability. Same codes are being using in different classes with only one time declaring.
There are five types of inheritance:
We use extends keyword for inheritng an existing class.
Below you can see an example of inheritance
class Vehicle{
public void start(){ ... }
}
class Car extends Vehicle {
public static void main() { start(); }
}
class Motocycle extends Vehicle {
public static void main() { start(); }
}
In above code block we created a Vehicle class. This class is our base class. We created Car and Motocycle classes and we derived them from Vehicle base class. We used start function which declared in base class without redeclaring in child classes.
Working with inheritance we need to pay attention access modifiers in base class.
import java.util.*;
public class Main {
public static void main(String[] args) {
Airbus a = new Airbus();
Boeing b = new Boeing();
a.initialize();
b.initialize();
}
}
public class Aircraft{
public void start(){
System.out.println("Started");
}
}
public class Airbus extends Aircraft{
public void initialize(){
System.out.println("Airbus initializing");
start();
}
}
public class Boeing extends Aircraft{
public void initialize(){
System.out.println("Boeing initializing");
start();
}
}
Program output will be like below:
Airbus initializing
Started
Boeing initializing
Started
In above program we created a Airvraft class. This class is our base class and we derived two child classes from this base class. We called the start function which declared in base class without redeclaring them. This is the basic usage of inheritance feature of object oriented programming.
Sometimes we may need to access to base class directly. In these cases we need to use super keyword. With super keyword are able to access the inherited class. Below example you will see the how to use super keyword and accessing to the super class.
import java.util.*;
public class Main {
public static void main(String[] args) {
Boeing b = new Boeing();
b.initialize();
}
}
public class Aircraft{
public void start(){
System.out.println("Started");
}
public void greetAircraft(){
System.out.println("Aircrafts are made for flying. This method called from base class");
}
}
public class Boeing extends Aircraft{
public void initialize(){
System.out.println("Boeing initializing");
start();
System.out.println("Super class invoking");
super.greetAircraft();
}
}
Program output will be like below
Boeing initializing
Started
Super class invoking
Aircrafts are made for flying. This method called from base class
That is all in this article.
Have a good class extending.
Burak Hamdi TUFAN
Comments