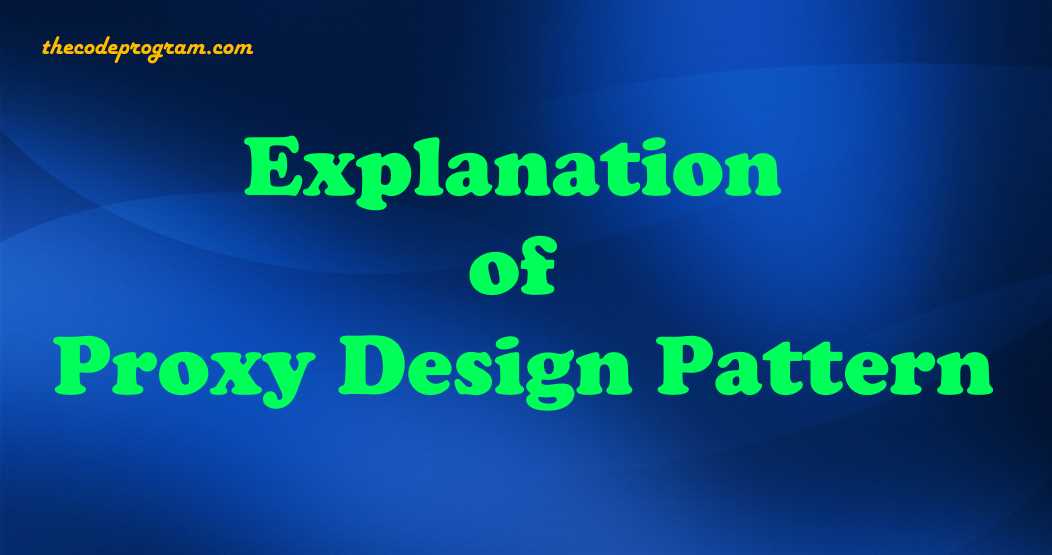
Explanation of Proxy Design Pattern
Hello everyone, in this article we are going to talk about Proxy design pattern within Structural Design Patterns. We will see what it is and then we are going to make an example on about Proxy Design Pattern.Let's Begin.
Proxy design pattern is one of the Structural design patterns. We can assign a purpose or the process of a component or a class to another object or component. This sub component can accomodate the process of this main component. So we can assign related sub component as a delegate for the main component. Generally using in remote systems.
Generally the developers use proxy design pattern at three situations :
- Remote Proxy : When we need to use a remote object the developer provide a local component to use it. For example we sometimes login to a web server via a mobile device. and remote login services.
- Protection Proxy : It checks the remote clients premissions and priviliges. For example if we need to login the service checks our permissions and allows or not to be logged in.
- Virtual Proxy : When our program doing big processes it shows a message that specify the loading instead of empty screen. Because real component is being preperad and we show a cache information or a message.
In proxy design pattern, it is good to know some terms which we will in need.
- Client : This term stands for a user or another api to use the proxy class to make some operations.
- Real Subject : Main class or object that represent the process.
- Subject : A derived class or interfaced class from RealSubject class which will represent the main purpose from everywhere.
- Proxy : It is a delegate class. It will contain the subject or Real subject class to make the operation according to clients requests.
Now let's make an example :
In this example we will have a client program and a class which is accomodate the real Subject of the program inside the main. Then I will create a real subject class named Server and i will create a proxy class. Both of these two classes are derived from same interface..IServer.cs
namespace ProxyPattern_Example
{
//This interface will derie the realsubject class and proxy class.
//I want both of them to be able to login to server.
interface IServer
{
bool login(string _username, string _password);
}
}
This class will be our RealSubject class. This related RealSubject class derived from our subject class interface In this class we are going to make the real operations
Server.cs
using System;
namespace ProxyPattern_Example
{
//This class will be our RealSubject class.
//This related RealSubject class derived from our subject class interface
//In this class we are going to make the real operations
class Server : IServer
{
private string username = "";
private string password = "";
private bool isLoggedIn = false;
public bool login(string _username, string _password)
{
username = _username;
password = _password;
if (username != "" && password != "")
{
if (username == "burak" && password == "12345")
{
Console.WriteLine("Logging in successful");
isLoggedIn = true;
return true;
}
else
{
Console.WriteLine("Wrong user parameters");
isLoggedIn = false;
return false;
}
}
else
{
Console.WriteLine("Can not send empty user parameters..");
isLoggedIn = false;
return false;
}
}
public bool check_isLoggedIn()
{
return isLoggedIn;
}
public void some_operations()
{
if (this.isLoggedIn)
{
Console.WriteLine("Operation representing...");
}
else
{
Console.WriteLine("Failed. You need to log in first...");
}
}
}
}
This class is our proxy class. We will make some operations via this class. This proxy class will make the operations on Server and we will able to use server class with here from everywhere of the program. As you can see both proxy and server classes derived from same interface to be able to accomodate same operations.
ProxyClass.cs
using System;
namespace ProxyPattern_Example
{
//This class is our proxy class. We will make some operations
//via this class. This proxy class will make the operations on
//Server and we will able to use server class with here from everywhere of the program.
//As you can see both proxy and server classes derived from same interface
//to be able to accomodate same operations
class ProxyClass : IServer
{
Server srv = new Server();
bool loggedin = false;
public void access_server()
{
if (srv.check_isLoggedIn() == false)
Console.WriteLine("You have to be loggedin first...");
else
Console.WriteLine("Accessing is successfull to the server...");
}
public void read_some_data()
{
srv.some_operations();
}
public bool login(string _username, string _password)
{
loggedin = srv.login(_username, _password);
return true;
}
}
}
Here is our subject. In the subject we directly access the server and make some operations from here. if you are working on the server it will be good to access directly.Lets see it.
Program.cs
using System;
namespace ProxyPattern_Example
{
class Program
{
static void Main(string[] args)
{
Console.Title = "Proxy Design Pattern Example - Thecodeprogram";
//Here is our subject. In the subject we directly access the server and make some operations from here
//if you are working on the server it will be good to access directly.Lets see it.
Console.WriteLine("Operations directly inside the server : ");
Server _server = new Server();
if (_server.check_isLoggedIn() == false)
Console.WriteLine("-You have to login first");
_server.login("burak", "12345");
if (_server.check_isLoggedIn())
Console.WriteLine("-You have logged in");
_server.some_operations();
Console.WriteLine("-----------------------------------------------");
Console.WriteLine("-----------------------------------------------");
Console.WriteLine("Operations Via Proxy Class : ");
ProxyClass proxy = new ProxyClass();
//first check is logged in and show the message to the client.
proxy.access_server();
proxy.login("burak", "12345");
//try to access to server again
proxy.access_server();
//after accessing to the server make some operations that only server can make.
//via our proxy class. Not to access directly server class via client
proxy.read_some_data();
Console.ReadLine();
}
}
}
That is all in this article.
Have nice Designing programs.
Burak Hamdi TUFAN
Comments