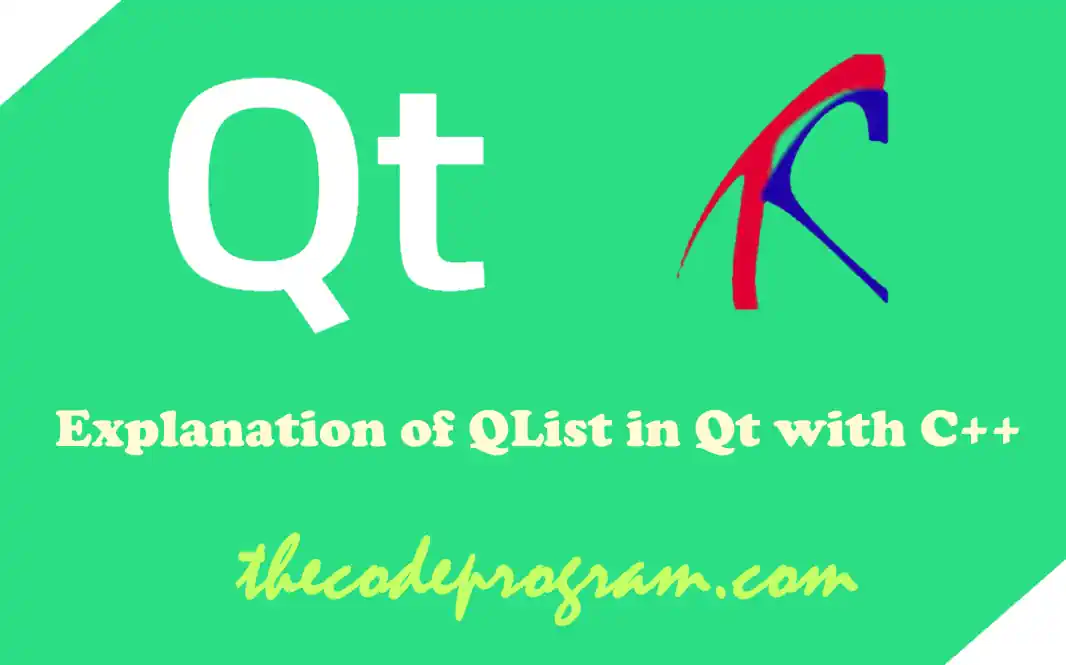
Explanation of QList in Qt with C++
Hello everyone, in this article we are going to talk about QList container in Qt with C++ programming language with the help of examples for better understanding.Let's get started
QList is one of the most important data storing structure in Qt Framework. QList helps to manage and store data in efficient way as a data sequence with a dynamic array template class in it.
Qlist is coming with Qt Core modules and QList store data like std::vector and std::list containers in C++ but QList are optimised for Qt applications and offers more additional features than standard lists in C++.
To use QList we need to import QList library and we should initialise the list.
// import the library
#include <QList>
int main() {
// initialise the QLists with template classes, so we can store in these data types
QList<double> numbers;
QList<QString> colors;
return 0;
}
Now lets see how to organise information within QLists.
Below lets make an example with above methods. For more details please check official documentation of Qt.
#include <QList>
int main() {
QList<QString> colors;
colors.append("red"); // Add value to end
colors.prepend("blue"); // Add value to beginning
colors.insert("green", 1); // Inserts value to index 1 and slide the rest
colors << "yellow"; // Add value to end
colors.removeAt(0); // Removes the element at specific index
colors.removeOne("yellow"); // Removes the first occurrence of value
QString str = colors.takeAt(0); // Removes element at zero position from list and returns removed element
QString val = colors.at(0); // Gets element at specified index
QString val2 = colors[0]; // Gets element at specified index
colors.clear(); // Removes all elements of list.
return 0;
}
We can iterate a QList in very easy and efficient way. Below you can see the example for this.
#include <QList>
int main() {
QList<QString> colors = {"red", "green", "blue"};
for (const QString& color : colors) {
qDebug() << colors;
}
return 0;
}
We also have some some algorithms like sort, filter, contains, join.... Qt framework provides some algorithms for QList also to use it easier and optimised way. Below you can see some examples for these algorithms.
#include <QList>
int main() {
// sort methods sorts the list in ascending order
QList<QString> colors = {"red", "green", "blue"};
colors.sort();
// filter returns a QStringList which fits the condition string
QStringList res;
res = colors.filter("r"); // red, green
bool exist = colors.contains("red"); // true
bool exist2 = colors.contains("RED", Qt::CaseInsensitive); //true
bool exist3 = colors.contains("RED", Qt::CaseSensitive); //false
// combines the elements with separator
QString colorsStr = colors.join(", "); //red, green, blue
return 0;
}
Conclusion
QList is very important container in Qt framework which makes easier to work with data collections. In Qt we can use QList directly instead of std::list to also have the optimisations for Qt Framework. In this article we have seen some basic operations of QList to manage the data collections in Qt.
That is all.
Burak Hamdi TUFAN
Comments