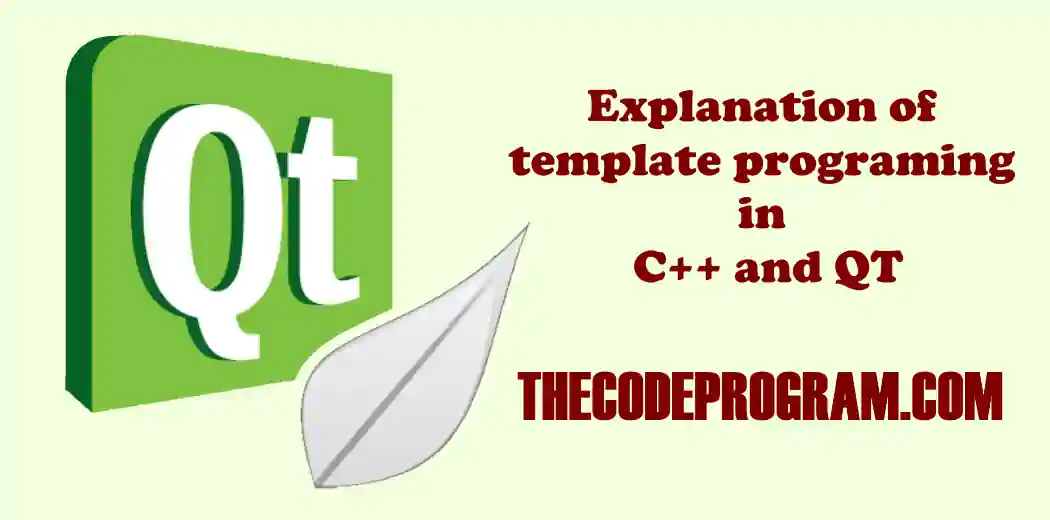
Explanation of template programing in C++ and QT
Hello everyone, in this article we are going to talk about template programming in C++. With template programming we are going to see how to declare generics.Let's Begin
Firstly we need to know a couple of definitions. These definitions are base of usage of template programming.
What is Generic Programming
Generic programming is developing a program as parametric based. It means we can use same class, method or any type of algorithm for different type of data types. For example we can use vectors with integer values and string values. These vectors are designed for generic using purposes.
What is Template Programming
Template programming is a efficient way to write generic blocks. Templates let us to write same code for different type of data types. We can create generic classes and methods in C++. Temlate programming is a way of compile time polymorphism
How Function Templates work in C++
Function template is a way of writing same method for different data types. We write a template method and use it in our program as usual. When the program is compiling, the compiler rewrite our template method according to our writing time parameter types. So we are gaining time with metaprogramming feature of our C++ compiler.When we write a template method and call it with different type of data types it will be like below:
template <typename T> void TemplateExample::myTemplateFunc(T p1, T p2){
//...
}
void TemplateExample::perform(){
this->myTemplateFunc(2.3, 5.6);
this->myTemplateFunc('a', 'v');
}
Above code block will be turned into below code block during compiling time :
void TemplateExample::myTemplateFunc(double p1, double p2){
//...
}
void TemplateExample::myTemplateFunc(char p1, char p2){
//...
}
void TemplateExample::perform(){
this->myTemplateFunc(2.3, 5.6);
this->myTemplateFunc('a', 'v');
}
As we can see metaprogramming is happening here and our template method is rewriting by compiler for overloading for all used data types .
What is Template Class in C++ ?
Template class is a way to write a class to use it with different data types at one time declerations. So we can write one class to use it for every data types. We can create template classes on same way with template functions.
template <typename T1, typename T2> class TheClass {
private:
//Define the variables inside
T1 key;
T2 value;
public:
};
And call it from another location like below:
TheClass <int, QString> *dict = new TheClass <int, QString>();
IMPORTANT
IN QT YOU CAN NOT USE SIGNAL-SLOT MECHANISM DIRECTLY IN TEMPLATES.
template<typename T1, typename T2> class TheClass {
//First we define our variables inside
T1 p1;
T2 p2;
public:
A(T1 p1, T2 p2) {
cout<<"Class initialized with parameters : "<< p1 << " and " << p2 <<endl;
}
};
At code definition initialization will be:
TheClass <int, int> the1 = new TheClass <int, int>(22, 33);
TheClass <QString, int> the2 = new TheClass <QString, int>("Burak Hamdi TUFAN", 33);
TheClass <int, QString> the3 = new TheClass <int, QString>(33, "The Code Program");
Program output will be like below:
Class initialized with parameters : 22 and 33
Class initialized with parameters : Burak Hamdi TUFAN and 33
Class initialized with parameters : 33 and The Code Program
Now we know how can we declare and use template classes in C++ programming language.
That is all in this article.
Burak Hamdi TUFAN
Comments