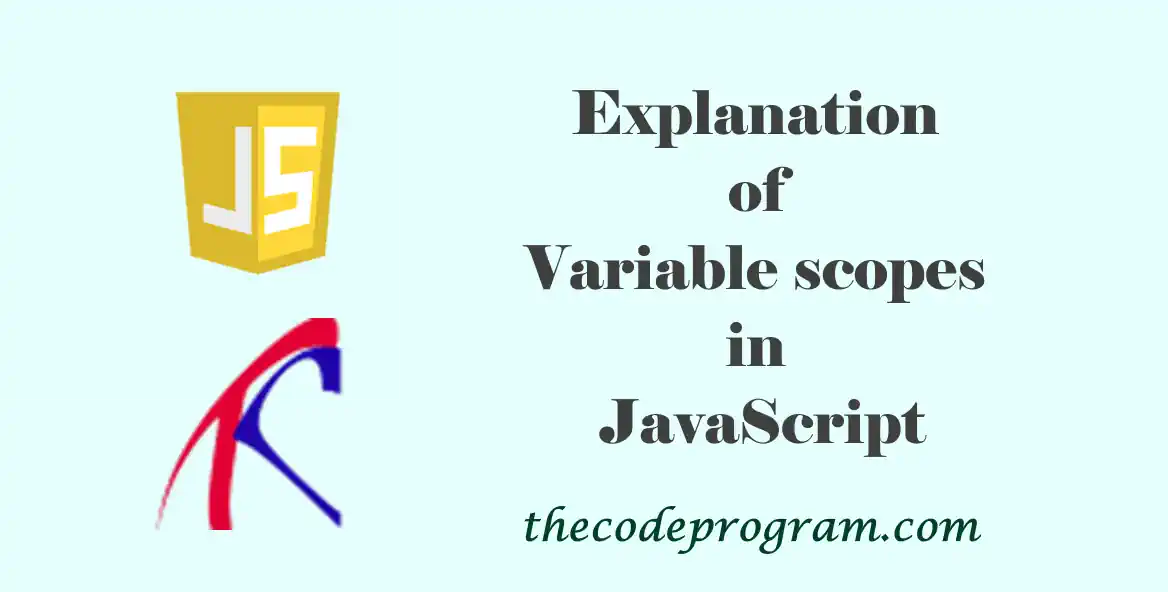
Explanation of Variable scopes in JavaScript
Hello everyone, in this article we are going to talk about scopes of variables in JavaScript and with code examples for better understanding.Let's begin.
JavaScript is one of most famous programming languages with especially its flexibility. In java script sometimes it might be a challenge the understanding variable scopes and using variables in the code. This is the reason we should learn the variable scope in Javascript for writing bug free code.
Variable scope specify the where a variable can be accessible. We can declare variables that can be accessible from all around the application or only from related class or function. In JavaScript there are two main types of Variable Scopes:
Global Scope
Variables are defining outside of any function or any block, So they are reachable globally at the application.
var globalVariable = 'This is a global variable';
function print() {
console.log(globalVariable); // Accessible from function
}
print();
console.log(globalVariable); // Accessible outside the function also and any other locations
Local Scope
Variables are defining within the function and it is accesible only within this function
function greet() {
var localVariable = 'Local variable definition';
console.log(localVariable); // Accessible within the function
}
greet();
console.log(localVariable); // Error: localVariable is not defined
Hoisting is one important feature about variable scope in JavaScrip. JavaScript hoists variable declarations to the top of their containing scope. This means that even if you declare a variable inside a block or a function, it is as if it were declared at the beginning of that block or function.
function hoistingExample() {
console.log(x); // Outputs 'undefined'
var x = 10;
console.log(x); // Outputs '10'
}
hoistingExample();
In this example, x is hoisted to the top of the hoistingExample function, but it is initialized with undefined. It's essential to be aware of hoisting to avoid unexpected behavior in your code.
Conclusion
Knowledge on variable scope is very important to write clean and maintainable code in JavaScript. By learning the concepts of variable scopes and additionally hoisting, you can avoid common pitfalls and write more efficient JavaScript code.
That is all
Burak Hamdi TUFAN
Comments