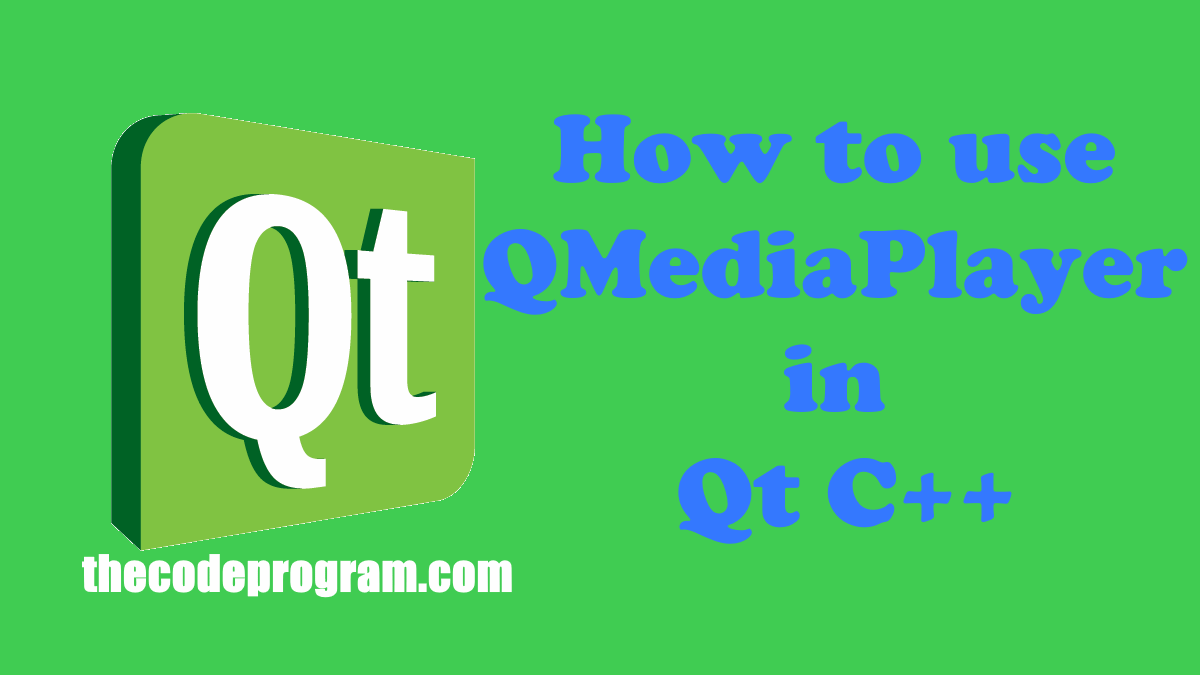
How to use QMediaPlayer in Qt C++
Hello everyone, in this article we are going to see QMediaPlayer in QT with C++. How can we use enable and use QMediaPlayer in QT C++ and we are going to make a simple example about it.Let's Begin.
To use media player widgets in our qt program, first we have to enable it via pro file.
Add below code block to your .pro file:
QT += multimedia
We are ready to use multimedia,
First I will import the QMediaPlayer and then I will declare a MediaPlayer variable in header file
#include <QMediaPlayer>
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = 0);
~MainWindow();
private:
Ui::MainWindow *ui;
QMediaPlayer *player;
};
After that we are going to initialize the QMediaPlayer at constructor. Here we initialize the QMediaPlayer with related Window And we set the volume of the player, not the device.
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
//here we initialize the QMediaPlayer with related Window
player = new QMediaPlayer(this);
//And we set the volume of the player, not the device.
player->setVolume(75);
}
Now I recommend you to declare some slots to set the media durations to the required variables.
on_positionChanged function is dedicated to get players current position. When the player keep playing it will update a progressbar and a label to show the player current position. on_durationChanged function will be work when media changed. Below media will be fired one times for every media changed. mediaStatuChngd method will get the media status. This method will be triggered when the media status changed of the QMediaPlayer. I declared second_to_minutes method to convert the current media duration to minutes and seconds.
//on_positionChanged function is dedicated to get players current position.
//When the player keep playing it will update a progressbar and a label to
//show the player current position.
void MainWindow::on_positionChanged(qint64 position)
{
ui->pbMusic->setValue(position);
ui->lblCurrentMusicDuration->setText( second_to_minutes(position / 1000).append("/").append( second_to_minutes( (ui->pbMusic->maximum())/1000 ) ) );
}
//on_durationChanged function will be work when media changed.
//Below media will be fired one times for every media changed.
void MainWindow::on_durationChanged(qint64 position)
{
ui->pbMusic->setMaximum(position);
}
//mediaStatuChngd method will get the media status. This method will be triggered
//when the media status changed of the QMediaPlayer.
void MainWindow::mediaStatuChngd(QMediaPlayer::MediaStatus state)
{
if(state == QMediaPlayer::EndOfMedia)
{
QMessageBox.inform(this, "Ended", "Music Ended");
}
}
//I declared second_to_minutes method to convert the current media duration
//to minutes and seconds.
QString MainWindow::second_to_minutes(int seconds)
{
int sec = seconds;
QString mn = QString::number( (sec ) / 60);
int _tmp_mn = mn.toInt() * 60;
QString sc= QString::number( (seconds- _tmp_mn ) % 60 );
return (mn.length() == 1 ? "0" + mn : mn ) + ":" + (sc.length() == 1 ? "0" + sc : sc);
}
So I am hearing the questions where did you setted these functions at to related methods. I wrote some signal-slot connections at constructor to do it. Below you can see it.
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
//here we initialize the QMediaPlayer with related Window
player = new QMediaPlayer(this);
//And we set the volume of the player, not the device.
player->setVolume(75);
connect(player,SIGNAL(positionChanged(qint64)) ,this,SLOT(on_positionChanged(qint64)));
connect(player,SIGNAL(durationChanged(qint64)) ,this,SLOT(on_durationChanged(qint64)));
connect(player,SIGNAL(mediaStatusChanged(QMediaPlayer::MediaStatus)),this,SLOT(mediaStatuChngd(QMediaPlayer::MediaStatus)));
player->setMedia(QUrl::fromLocalFile("your_music_path"));
player->play();
}
That is all in this article.
Have a good Media Playing.
Burak Hamdi TUFAN
Comments