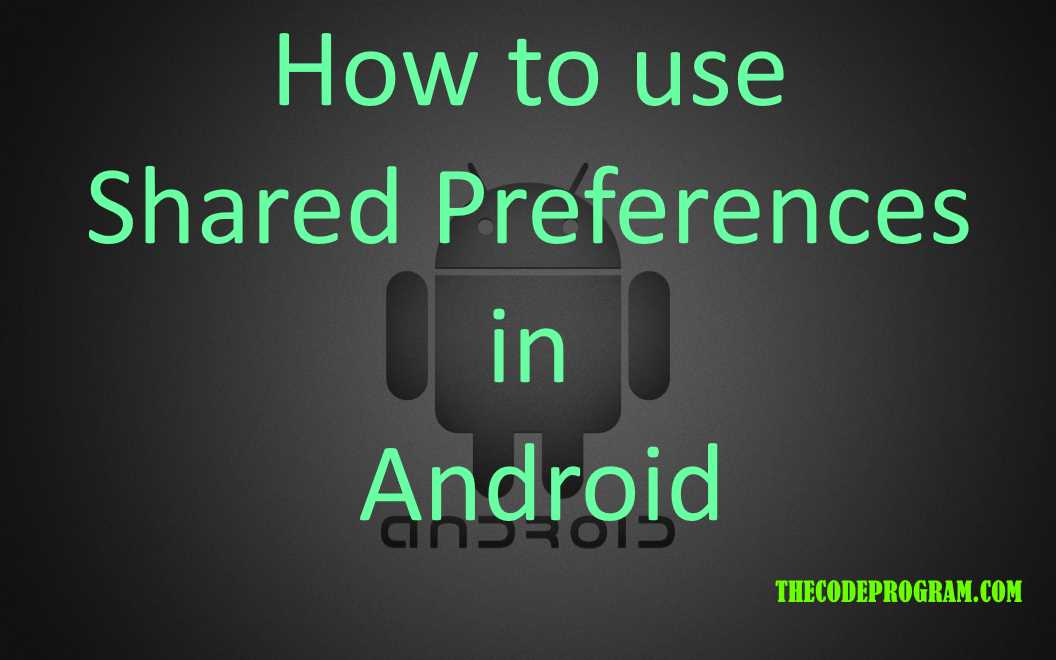
How to use Shared Preferences in Android
Hello everyone, in this article we are going to talk about Shared Preferances in android. We are going to use android studio and Java language and we will make an example.Let's begin.
Sometimes we to keep spme data even the application is closed. We can use databases like sqlite and realm or something like them. Using the databases for big value is good but if we are going to keep a few values it is not a good idea to use databases. So in these cases it is recommended to use Shared Preferances in android.
We can keep the datas which are string, int, float, long and boolean. The Datas are holding as key-value pairs in an XML file. Android writes the shared preferances files in an XML file into application package folder under DATA folder. When the user removed the application or clean the application the shared preferances will e removed.
There are some kind of method to start shared preferances. Below you can see:
- getPreferences() : We use this method at our activity to access activity-levelshared preferances.
- getSharedPreferences() : We use this method at our activity to access application-level shared preferances.
- getDefaultSharedPreferences() : This method work with PreferenceManager and it will work on all around the application.
In this article we are going to use getDefaultSharedPreferences() with PreferanceManager to use it everywhere of application.
First I will initialize the SharedPreferances and SharedPreferances editor. And then I will put my values with keys via the editor. And last we have to commit our changes on the editor.
//First I will initialize the SharedPreferances and SharedPreferances editor.
SharedPreferences preferences = PreferenceManager.getDefaultSharedPreferences(MainActivity.this);
SharedPreferences.Editor editor = preferences.edit();
//And then I will put my values with keys via the editor.
editor.putString("web", "https://thecodeprogram.com");
editor.putInt("int_value", 33);
editor.putLong("long_value", 99999999999999);
editor.putFloat("float_value", 5555555555);
editor.putBoolean("boolean_value", true);
//And last we have to commit our changes on the editor.
editor.commit();
Now our datas are saved. Here we read the saved datas.
First we declare and initialize the SharedPreferances. This will get the saved value and if this value is not exis it will get empty value or whatever you want as default.
//First we declare and initialize the SharedPreferances.
SharedPreferences shared_preferences;
shared_preferences = PreferenceManager.getDefaultSharedPreferences(MainActivity.this);
//This will get the saved value and if this value is not exis it will get empty value or whatever you want as default.
String web = shared_preferences.getString(web, "");
int int_value = shared_preferences.getInt("int_value", 33);
long long_value = shared_preferences.getLong("long_value", 99999999999999);
float float_value = shared_preferences.getFloat("float_value", 5555555555);
boolean boolean_value = shared_preferences.getBoolean("boolean_value", true);
Also if you want to remove some values or clear entire values from shared preferances. Below you can see it:
First I will initialize the SharedPreferances and SharedPreferances editor. To remove the specified datas with keys you can use remove method with specified keys And commit to changes. If you want to clear entire all values you can use the clear method.
//First I will initialize the SharedPreferances and SharedPreferances editor.
SharedPreferences preferences = PreferenceManager.getDefaultSharedPreferences(MainActivity.this);
SharedPreferences.Editor editor = preferences.edit();
//To remove the specified datas with keys you can use remove method with specified keys.
editor.remove("web");
editor.remove("int_value");
//And commit to changes.
editor.commit(); // commit changes
//If you want to clear entire all values you can use the clear method.
editor.clear();
editor.commit(); // commit changes
That is all in this article.
Have a good shared preferance using.
I wish you all very healthy days.
Burak Hamdi TUFAN.
Comments