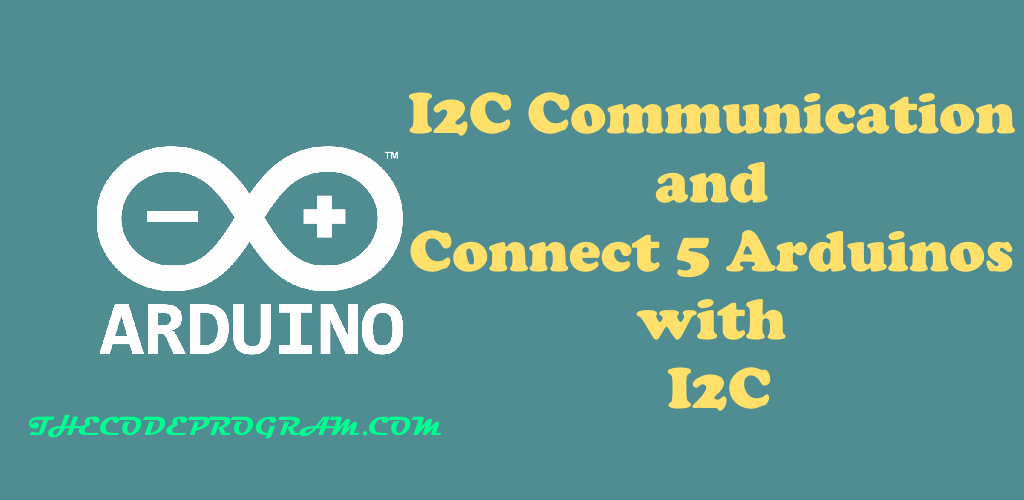
I2C Communication and Connect 5 Arduinos with I2C
Hello everyone, in thi article we are going to talk about I2C communication in arduino. First, we will talk about I2c and later we are going to make an example in arduino. In here we are going to communicate between multiple arduinos.Let's get started.
Firstly, What is I2C?
I2C stands for Inter Integrated Circuits. I2C allows the devices to communicate with two wires and multiple slaves. There is no need for selection pins for every device, all devices connect to the same communication bus and let them being communicated. I2C has less speed than SPI.At first it communicates at maximum 100kHz and with Fast Mode I2C 400kHz with High Speed 3.4 MHZ and with ultra Fast mode 5MHz. All devices on the bus must have an adress to prevent confusing the target.
Today mostly devices have I2C communication protocol. So many environmental sensors are using the I2C communication. All devices must have pull-up resistors at the bus connections. Every device start to communication with a start signal; and stop the communication with a stop signal on the bus. The I2C communication supports almost unlimited device to communication but the adress value can be 7-bit value. So the protocol spuurts 127 device at the same time. Master device do not need to an adress.
There are Two line on the I2C communication bus. These are SCL(Serial Clock) and SDA(Serial Data).
- SDA : Transfer the data between masters devices and slave devices. This transferring goes on both sides.
- SCL : This line oscilate a clock pulse to synchronise the communication for all devices.
Our explanation is over here, Now We will make an example about I2C with Arduino.
In this example we will connect five arduinos together. Below Image you can see the connections of arduinos.
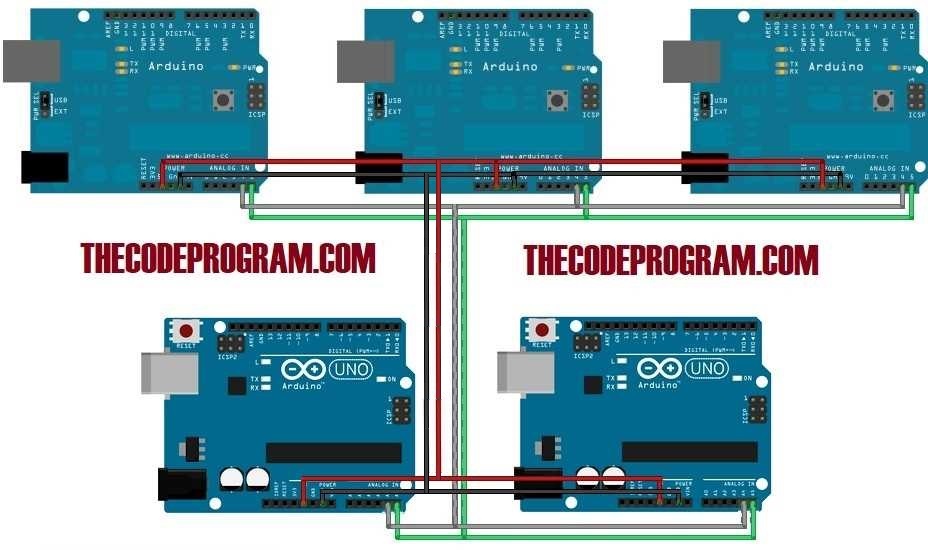
Master Device Code
master.ino
//I2C Communication Master Device
#include <Wire.h>
//Define slave devices Adress ID's
#define DEVICE_ID_1 1
#define DEVICE_ID_2 2
#define DEVICE_ID_3 3
#define DEVICE_ID_4 4
#define DEVICE_ID_5 5
//Define the values that will be read from slave devices
#define DEVICE_1_SIZE 5
#define DEVICE_2_SIZE 15
#define DEVICE_3_SIZE 32
#define DEVICE_4_SIZE 20
#define DEVICE_5_SIZE 5
//Output variables
String output_1 = "";
String output_2 = "";
String output_3 = "";
String output_4 = "";
String output_5 = "";
String all_outputs = "";
void setup()
{
Serial.begin(9600);
output_1 = "";
output_2 = "";
output_3 = "";
output_4 = "";
output_5 = "";
all_outputs = "";
//Activate the I2C communication
Wire.begin();
}
void loop()
{
read_I2C_bus();
delay(100);
}
void read_I2C_bus(){
output_1 = "";
output_2 = "";
output_3 = "";
output_4 = "";
output_5 = "";
all_outputs = "";
//We requesting data from slave device with data size
Wire.requestFrom(DEVICE_ID_1, DEVICE_1_SIZE);
while (Wire.available()) {
char c = Wire.read();
output_1 += c;
}
Wire.requestFrom(DEVICE_ID_2, DEVICE_2_SIZE);
while (Wire.available()) {
char c = Wire.read();
output_2 += c;
}
Wire.requestFrom(DEVICE_ID_3, DEVICE_3_SIZE);
while (Wire.available()) {
char c = Wire.read();
output_3 += c;
}
Wire.requestFrom(DEVICE_ID_4, DEVICE_4_SIZE);
while (Wire.available()) {
char c = Wire.read();
output_4 += c;
}
Wire.requestFrom(DEVICE_ID_5, DEVICE_5_SIZE);
while (Wire.available()) {
char c = Wire.read();
output_5 += c;
}
all_outputs = output_1 + ":" + output_2 + ":" + output_3 + ":" + output_4 + ":" + output_5;
Serial.println(all_outputs);
}
Now we need to setup our slave devices. All of the codes will be on same logic. I will write slave device code one time and you can apply them for all slave devices. First I imcluded the required Wire.h library and I defined DEVICE_ID. I defined as 1 and you can defined it for all devices. Make sure the defined device exist in the master device. Else you will not connect them
Below code blocks you will see the code:
slave_device_1.ino
//I2C communication libary
#include <Wire.h>
//Slave Device ID
//This value has to be exist in the master.
//And you have to define it for all device differantly.
#define DEVICE_ID 1
void setup() {
//Start the serial usb communication to send data via usb serial port
Serial.begin(9600);
//We started I2C communication with the slave device ID
Wire.begin(DEVICE_ID);
//The method which be fired when I2C request occured
Wire.onRequest(requestEvent);
}
void loop() {
//I do not need any code here, because I will make the operations on the requestEvent function
}
void requestEvent()
{
//Five charactered data for device 1 to send on the bus
String data = "xxxxx";
Serial.println(data );
//Write data with I2C
Wire.write(data .c_str());
}
That is all in this article.
Have a good I2C communication.
Burak Hamdi TUFAN
Comments