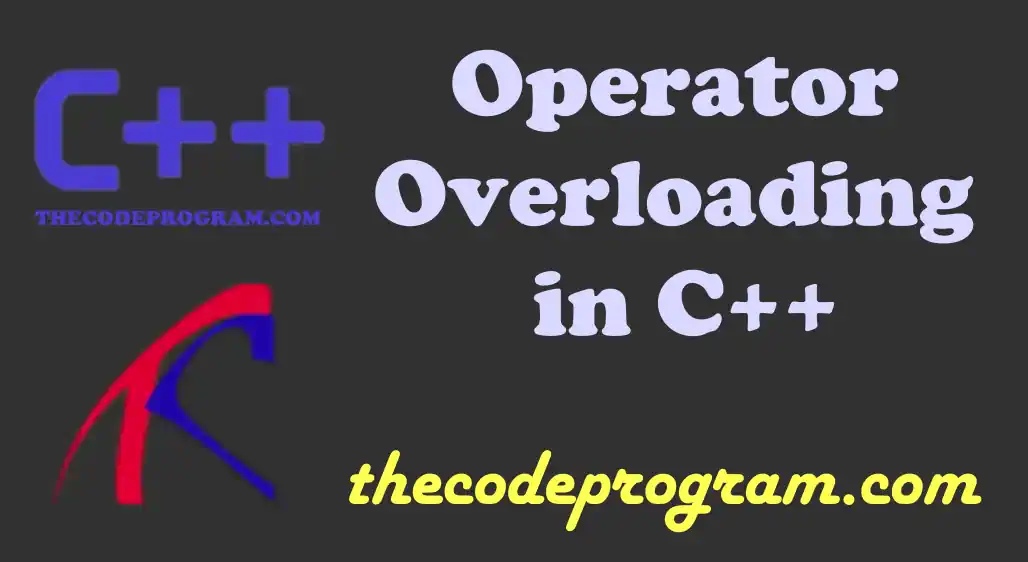
Operator Overloading in C++
Hello everyone, in this article we are going to talk about operator overloading in C++ programming language. We will see how to overload an operator with examples.Let's begin
What is Operator Overloading
Operator overloading is adding more user-defined functionalities to operators and letting them to work as user defined functions.
We can overload the assigned function and redefine the functionality of related operator. For example we can combine two strings with plus operator. We can redefine this function and redefine according to our requirement.
returningType operator symbolOfOperator(parameter) {
//overloaded operation
}
Now let's make an operator overloading example for better understanding. In this example I created two classes Square and Circle. I will make somre operations with overloaded operators.
class Square {
private:
int area;
public:
Square(double _area = 0) {area = _area; }
void printArea() { cout << area << endl; }
Square operator+(const Square& _s) {
Square s;
return s.area = this->area + _s.area;
}
};
We will initialzie this class with a specified area value. In this class we overloaded (+) operator for summing areas of two different squares.
Square sq1(50), sq2(90);
cout << "Area of Square 1 is ";
sq1.printArea();
cout << "Area of Square 2 is ";
sq2.printArea();
Program output will be:
Area of Square 1 is 50
Area of Square 2 is 90
Summarized area is 140
Another example class have more overloadings. In here we overloaded three operators. We have much more operations in this class.
class Circle {
private:
const double PI = 3.14;
double radius;
double area;
void calculateArea(){
area = PI * radius * radius;
}
public:
Circle(double _radius = 0) {
radius = _radius;
this->calculateArea();
}
void printData() {
cout << "Radius is " << radius << " and Area is :" << area << endl;
}
void operator |=(const double _rad) {
this->radius = _rad;
this->calculateArea();
}
void operator +=(const double val) {
this->radius = this->radius + val;
this->calculateArea();
}
void operator %=(const double _area) {
this->area = _area;
this->radius = sqrt(this->area / this->PI);
this->calculateArea();
}
};
In this class we overloaded operators:
In all overloaded operators we re calculated the area of circle.
Circle circle(10);
circle.printData();
circle |= 25;
circle.printData();
circle += 33.965;
circle.printData();
circle %= 314000;
circle.printData();
Example Output will be
Radius is 10 and Area is :314
Radius is 25 and Area is :1962.5
Radius is 58.965 and Area is :10917.4
Radius is 316.228 and Area is :314000
As you can see above example we overloaded |= , += and %= operators. We set some values and calculated the related datas according to these values.
That is all in this article.
You can reach the source code file on Github via https://github.com/thecodeprogram/TheSingleFiles/blob/master/OperatorOverloadingInCPlusPlus.cpp
Have a good operator overloading
Burak Hamdi TUFAN
Comments