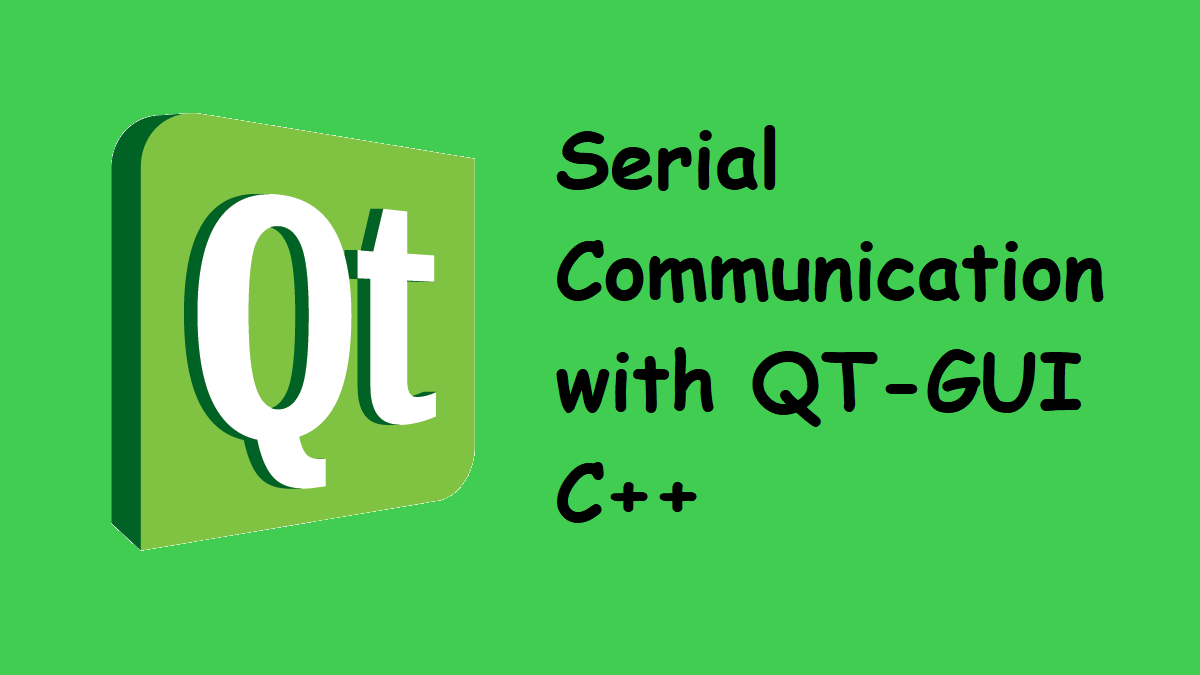
Serial Communication with QT-GUI C++
Hello everyone, in this article we are going to talk about how can we communicate with embedded systems via UART Serial Port using QT-GUI C++. You can use this example on your own test programs.Now let's get started.
First we have to add below code in .pro file of project to use QSerialPort Class in our project.
QT += serialport
Now we are ready to use QSerialPort Class in QT C++.
We shall add following class in head of header file of our class.
#include <QtSerialPort/QSerialPort>
and the below code block in private section:
private:
QSerialPort *serial;
Now we can use the serial port in our program. Lets write some more codes. Open the .cpp file write below functions.
void MainWindow::fnc_openSerialPort()
{
serial->setPortName("COM1");
serial->setBaudRate(9600);
serial->setDataBits(QSerialPort::DataBits);
//We will chose the parity bits
serial->setParity(QSerialPort::Parity);
//We will chose the stop bits
serial->setStopBits(QSerialPort::StopBits);
//We will chose the Flow controls
serial->setFlowControl(QSerialPort::FlowControl);
if (serial->open(QIODevice::ReadWrite)) {
} else {
QMessageBox::critical(this, tr("Error"), serial->errorString());
}
}
This is the code block to open COM1 serial Port with 9600 BaudRate and the other values.
And below function to close opened serial port.
void MainWindow::fnc_closeSerialPort()
{
if (serial->isOpen())
serial->close();
}
To write data on Serial Port via opened COM port first we need to check the port is opened and avail to write some data. we have to write the data with ASCII values.
void MainWindow::writeData()
{
if(serial->isOpen())
serial->write(ui->txtSerialSendData->text().toLatin1() );
else
QMessageBox::critical(this, tr("Write Error"), serial->errorString());
}
If we want to write data with the writeline we can change the code block like below:
if(serial->isOpen())
serial->write(ui->txtSerialSendData->text().toLatin1() + char(13) );
else
serial->write(ui->txtSerialSendData->text().toLatin1() );
And also we need to read datas on Serial Port. We can do it with below code block.
void MainWindow::readData()
{
bool _ok;
//This code is to read all data on serial port
QByteArray data = serial->readAll();
if (ui->chkGetHexadecimalValue->isChecked() == true)
qDebug() << data.toHex(); //Here is the read data as Hex values
else
qDebug() << data; // Here is the code block to read normal latin words.
}
Now we can use the serial port with our QT GUI C++ program.
That is all for this article.
Have a good communication.
Burak hamdi TUFAN
Tags
Share this Post
05/05/2015
Dev-C ile Bir Sayının Katlarını Bulma
25/04/2023
Explanation of Inheritance in C++
04/10/2023
Comments
Hocam merhabalar, daha önce birçok kaynaktan faydalanmaya çalıştım, sizin kaynağınızdaki kodları da denedim fakat sonuç alamadım. Acaba veriyi gönderip göndermediğimi nasıl anlayabilirim ? Sorunun hangi tarafta olduğunu merak ediyorum da. Kart: Raspberry Pi 4 İşletim Sistemi : Raspbian
2020/04/16 15:33:33Merhaba, Öncelikle problemin nerede olduğunu anlayabilmek adına farklı bir seri haberleşme cihazı veya programı deneyebilirsiniz. Bu sayede hata cihazınızda ise cihaz kodlarını kontrol edebilir veya hata programda ise programdaki kodları kontrol edebilirsiniz.
2020/04/16 17:06:26