Angular Signals: A Game-Changer for State Management
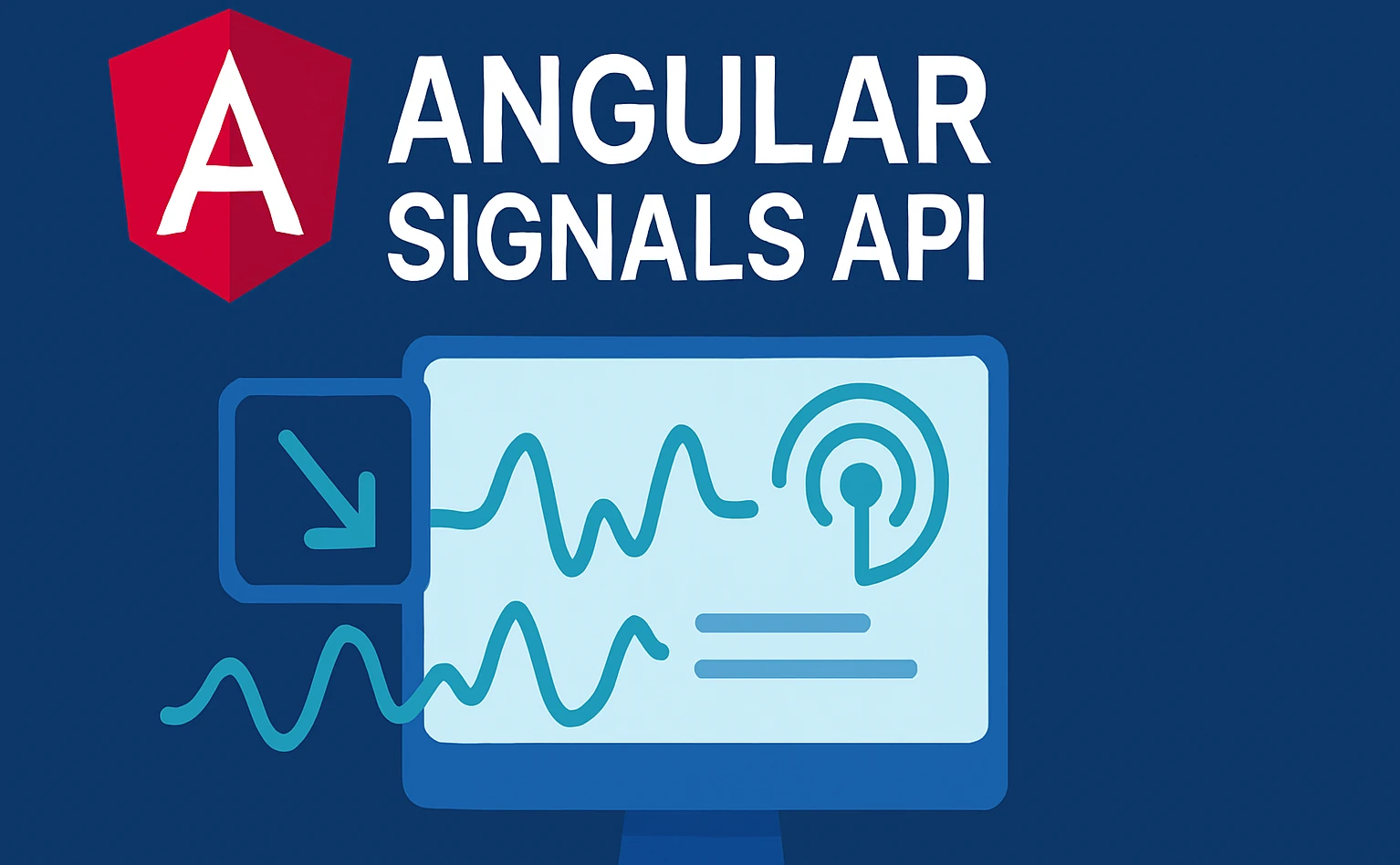
Lets get started.
As we all know angular has a great place at all amoung of web development technologies. Angular owes this to its constant evolution and improvements on the experience and performance for web applications. One of the latest important improvement of Angulat is Signals for state management. With Signals Angular provides a new reactive model for state management to improve the performance of Angular based applications.
What Are Angular Signals?
Angular Signals provide a new way to manage reactivity in Angular applications. They are lightweight, dependency-free, and improve performance by making state updates more predictable. Signals act as reactive variables that track dependencies and trigger updates only when necessary, reducing unnecessary computations and improving application efficiency.
Why to Use Angular Signals?
There are plenty of reasons to use Angular SignalsHow Angular Signals Work?
Lets create a signal first
To create a signal we can use the signal function which is provided by Angular, Here is an example:
import { signal } from '@angular/core';
export class CounterComponent {
count = signal(0);
increment() : void {
this.count.set(this.count() + 1);
}
}
In the above example, the count signal holds the reactive state as the given initial data. When the increment method is called, it will trigger a set of the signal and it will automatically update the UI where the above signal has been used.
We can read the signal with the calling it as a function in the required places.
<p>Count: {{ count() }}</p>
<button (click)="increment()">Increment</button>
Signals also can be derived with the compute function of the Angular. With the compute function we can set another signal which is depending on the existing one.
import { computed } from '@angular/core';
export class CounterComponent {
count = signal(0);
doubleCount = computed(() => this.count() * 2);
}
In this code block, computed function will be invoked everytime the dependent signals are updated automatically. So keep this in mind to avoid unnecessary computations.
Effects in Signals
Effect function needs to be defined in constructor.
import { effect } from '@angular/core';
export class LoggerComponent {
count = signal(0);
constructor() {
effect(() => console.log('Count changed:', this.count()));
}
}
In above code block, Whenever the count signal is update, the function inside the effect will be invoked. It is a bit different from compute function. Compute function is calculates and create a new signal. Effect is not creating a new signal. It invoke a function.
Signals vs RxJS basic comparison
Angular is providing a simplier built-in way for state management, Signals are an alternate for RXJS and also will be much stronger alternate in the future. But for now, definitelly it is not a replacement for RXJS. RxJS is remaining to perform more complex operations still. But we need to keep in mind, Signals are more performant for small operations.Summary
Angular Signals a great improvement for the state management for reactive applications with Angular. It offers unnecessary computation easy debugging and less memory usages at the first look. This makes the development simplfy and provides more maintainable code. As Angular continue to be improved, it also promise the improvement of Signals for the development ecperience work flow. I strongly recomend you to take a look at it if you did not yet.
Happy signals
Burak Hamdi TUFAN