Explanation of Singleton Design Pattern in C++
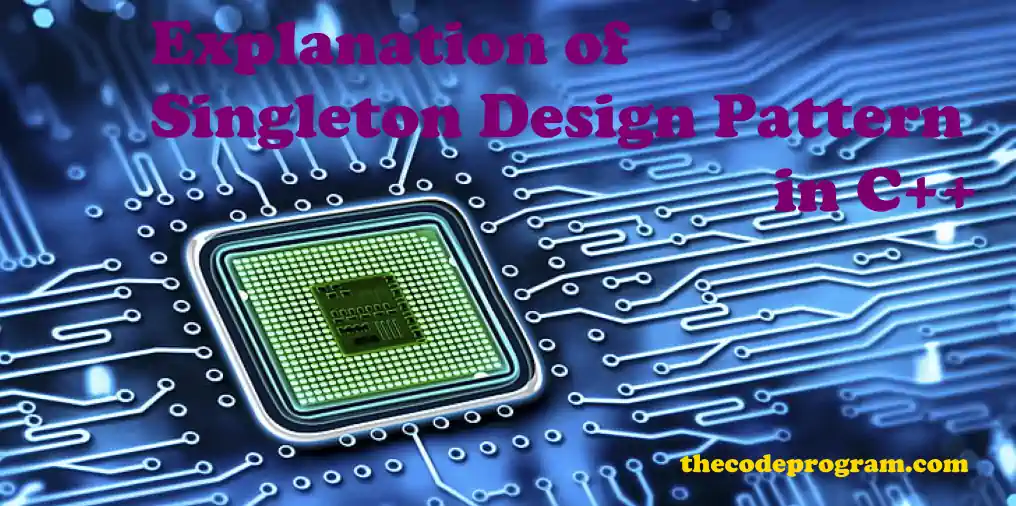
Let's get started.
Firstly, What is Singleton Design Pattern
Singleton Design Pattern provides one global object for all classes. We create a class and its own instance. Singleton class will manage its own instance. Also it provides a global access point. All classes reach this singleton classes via this access point.
#include <qcoreapplication>
#include <iostream>
#include <qstring>
using namespace std;
</qstring></iostream></qcoreapplication>
//We create a class with public property
class Configurations{
public :
int volume;
bool notification;
};
Now below we are going to create a Singleton class. Then we are going to create a public instance. We are going to access this singleton class through this instance from all around the program.
//Now create the singleton class
class Singleton {
static Singleton *instance;
Configurations *config;
// initialize the related configuration class at constructor
Singleton() {
config = new Configurations();
}
//First initialize the instance. All classes will access via this instance
public:
static Singleton *getInstance() {
if (!instance) instance = new Singleton;
return instance;
}
//Getters and setters of related functions of realted configurations
void setVolume(int vol) { this->config->volume = vol; }
int getVolume() { return this->config->volume; }
void setNotification(bool notify) { this->config->notification = notify; }
bool getNotification() { return this->config->notification; }
};
And then we are going to use getters and setters in singleton class to manage the configurations.
Singleton *Singleton::instance = 0;
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
system("title Singleton Design Pattern Example - Thecodeprogram");
cout << "Welcome Singleton Design Pattern Example in QT C++" << endl << endl;
Singleton *s = s->getInstance();
s->setVolume(50);
cout << "Volume level is : " << s->getVolume() << endl;
s->setNotification(true);
cout << "Notification sending config is : " << s->getNotification() << endl;
return a.exec();
}
You can reach the example code on Github : https://github.com/thecodeprogram/TheSingleFiles/blob/master/QSingletonPattern_Example.cpp
That is all in this article.
Burak Hamdi TUFAN