How to Create Custom Widget in QT C++
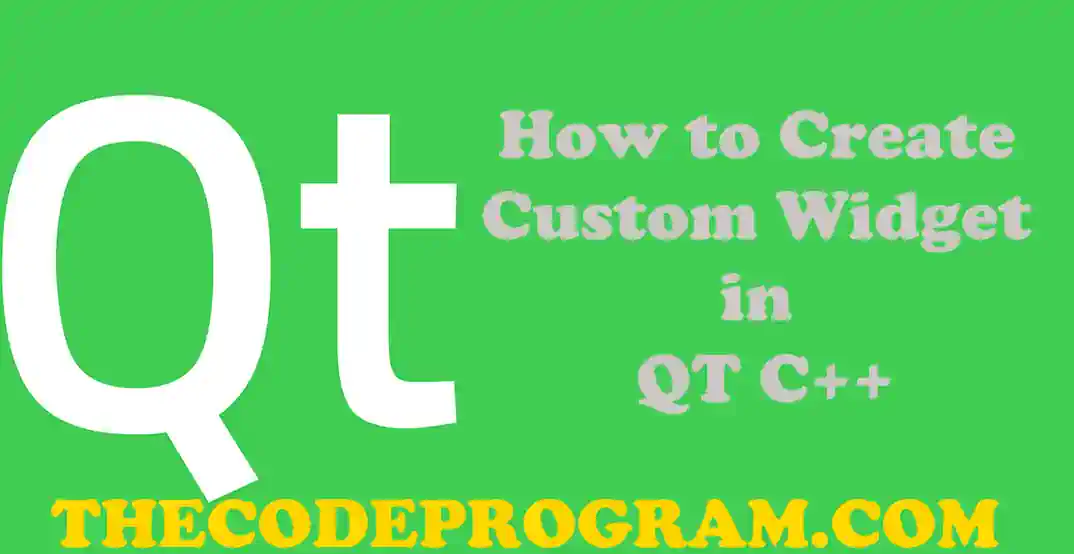
Let's get started...
Lets create our class which derived from QWidget class. This is the basic header file of my class.
thewatch.h
class TheWatch : public QWidget
{
Q_OBJECT
public:
TheWatch(QWidget *parent = nullptr);
};
Now let's add our required element, signals and slots in it. In here I will add QLabel to show the current time. Also I will write a slot to update the value of QLabel.
thewatch.h
class TheWatch : public QWidget
{
Q_OBJECT
Q_ENUMS(Priority)
public:
TheWatch(QWidget *parent = nullptr);
private :
QLabel *lblWatch;
private slots:
void writeUpdatedTime();
signals:
void timeUpdated(QString timetext);
};
Here I added Q_OBJECT macro to use QObject features. In here I will use signal and slots so I need to add Q_OBJECT macro inside the private area of my custom widget class. Q_OBJECT macro is required.
I defined the QLabel here and I will initialized it in implementation side.
Now let's make our custom class implementation. In here I class constructor and update slot method.
thewatch.cpp
TheWatch::TheWatch(QWidget *parent)
{
QHBoxLayout *layout = new QHBoxLayout(this); // this keyword is iimportant, we tell the widget the parent withn be showed
layout->setMargin(0);
lblWatch = new QLabel(QString("00:00:00"));
QString strFont = "font: 75 108pt 'Comic Sans MS',";
lblWatch->setStyleSheet(strFont);
lblWatch->setAlignment(Qt::AlignCenter);
lblWatch->setMargin(0); // to stretch the widget
qDebug() << "TheWatch Constructor";
layout->addWidget(lblWatch);
}
void TheWatch::writeUpdatedTime()
{
lblWatch->setText( QDateTime::currentDateTime().toString("hh:mm:ss") );
}
In constructor I have created a HorizontialLayout and added my QLabel in it. Then I configured QLabel's visual properties. And astly added it in my HorizontialLayout. In writeUpdateTime slot we will set the time which is coming with timetext parameter.
Important : You can create only one Layout while creating a custom widget. So we can not create another Horizontial or Vertical etc. layouts.
maiinwindow.cpp
CustomDigitalWatch::CustomDigitalWatch(QWidget *parent) :
QWidget(parent),
ui(new Ui::CustomDigitalWatch)
{
ui->setupUi(this);
theWatch = new TheWatch();
QGridLayout *layout = new QGridLayout;
layout->setMargin(0);
layout->addWidget(theWatch);
ui->widgetWatch->setLayout(layout);
QTimer *timer = new QTimer(this);
connect( timer , SIGNAL(timeout()), theWatch, SLOT(writeUpdatedTime()) );
timer->start(1000);
}
As you can see above our custom widget is ready and we are good to adapt it for our requirements.
That is all in this article.
Burak Hamdi TUFAN