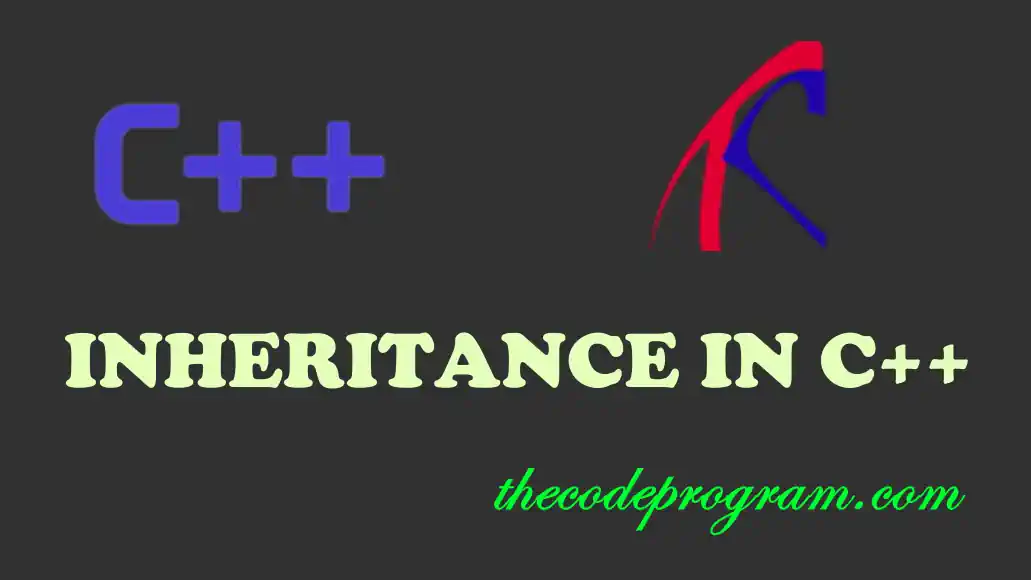
Explanation of Inheritance in C++
Hello everyone, in this article we are going to talk about Inheritance concept of Object Oriented Programming Languages within C++ with examples.Let's get started.
Inheritance is one of most important concept in object-oriented programming (OOP) that allows us to create new classes based on existing ones. Within C++, Inheritance is a powerful feature that allows us to reuse the code and create a class hierarchy. Also we are able to improve the code quality. With this article you will see the basics of inheritance in C++ and code examples for beginners.
What is Inheritance?
Inheritance is a relationship between two classes where one class, known as the base class or parent class, provides some of its attributes and methods to another class, known as the derived class or child class. The derived class can then use these attributes and methods, as well as add its own.
Below you can see how to inherit a class in C++
class ParentClass {
// ...
// Definitions
// ...
};
class DerivedClass : public ParentClass {
// ...
// Definitions and usages of parent class
// ...
};
In C++, there are 3 access types of inheritance:
Below you can see access modes of inheritance in C++
class ParentClass {
// ...
// Definitions
// ...
};
class DerivedClass : private ParentClass {
// Can Access private members of parent class
};
class DerivedClass1 : public ParentClass {
// Can Access public members of parent class
};
class DerivedClass2 : protected ParentClass {
// Can Access protected members of parent class
};
Important Note: Please do not confuse Access Modes and Inheritance Types in C++.
Now lets talk about inheritance types of C++. There are five types of inheritance:
Now, Let's make more examples for better understanding of Inheritance in C++
class BaseClass {
public:
void printBase() {
cout << "This class is base." << endl;
}
};
class DerivedClass1 {
public:
void printDerived1() {
cout << "This class is first derived class" << endl;
}
};
class DerivedClass2 : public DerivedClass1 {
public:
void printDerived2() {
cout << "This class is second derived class" << endl;
}
};
int main() {
//Class initializations
DerivedClass1 obj1;
DerivedClass2 obj2;
// Single Inheritance printing
obj1.printBase();
obj1.printDerived1();
//Multi level Inheritance printing
obj2.printBase();
obj2.printDerived1();
obj2.printDerived2();
return 0;
}
In above example we can see two types of inheritance types:
Now, Let's make another example
#include <iostream>
using namespace std;
// Base Classes
class Animal {
public:
void speak() {
cout << "This is base Animal class" << endl;
}
};
class Plant {
public:
void speak() {
cout << "This is base Plant class" << endl;
}
};
//Derived Classes
class Cat : public Animal {
public:
void speak() {
cout << "Meow" << endl;
}
};
class Dog : public Animal {
public:
void speak() {
cout << "Woof" << endl;
}
};
class Fungi : public Animal, public Plant {
public:
void speak() {
cout << "Whuuu" << endl;
}
};
int main() {
// Definitions and usages
return 0;
}
In above example we can see two types of inheritance types:
As a result we can use inheritance concept to increase the reusability and we can improve the grouping of the code.
That is basically all.
Burak Hamdi TUFAN
Comments