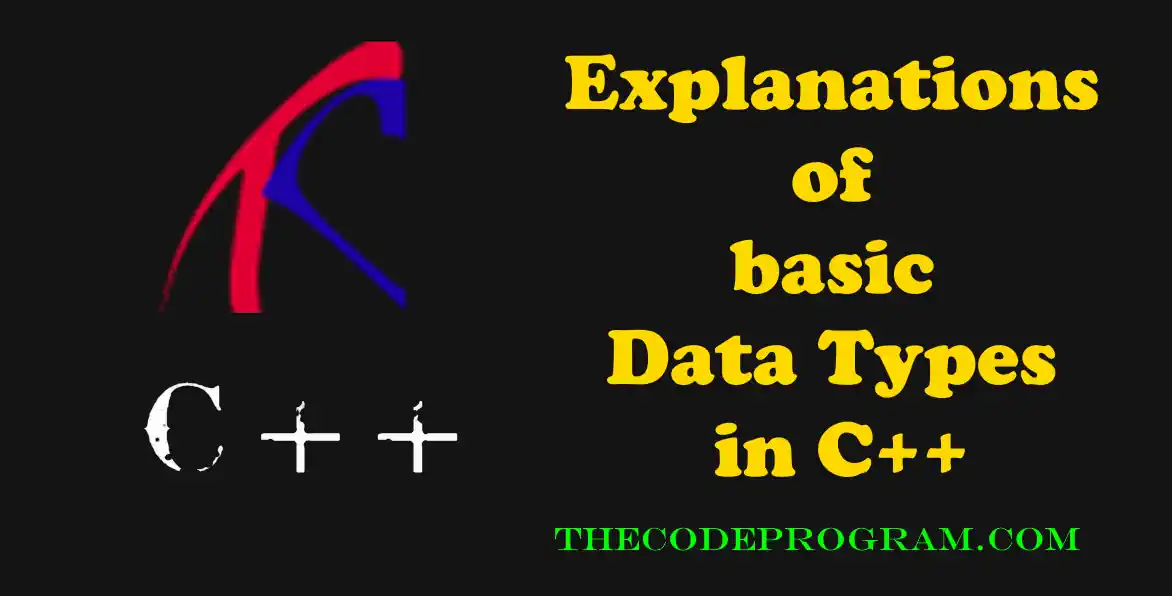
Explanations of basic Data Types in C++
Hello everyone, in this article we are going to talk about Data Types from basics to advanced in C++ and we will make some examples for better understanding.Let's begin
Understanding the data types are very important for all of the programming languages. They help us to store the data in memory and process them when needed in convenient way. With data types we tell to compiler the size and type of the data and the compiler allocate it on memory according to data type definition.
In C++ we have various types of data types from Basic data types to user defined and more... In this article we are going to deep dive into these data types in C++.
We can store and process integer values with int data type. int can be positive and negative numbers. We can also check uint only for positive numbers.
int myInteger = 42;
We can store and process the numbers which has floating point. Double data type has more precision then float.
float myFloat = 3.14159;
double myDouble = 2.71828;
We can store and process the true false values with bool data types. bool data type some times also represents 0 and 1 values to describe false and true.
bool bTrue= true;
bool bFalse = false;
We can store and process single characters with char data type. We are showing them within single quotes not like strings.
char ch= 'A';
We can store multiple same type elements in an array. We can access them with one data and iterate and process them with arrays.
int arr[5] = {1, 2, 3, 4, 5};
We can group some data with different types. We can group them and use with strcuts
struct Student{
std::string name;
char grade;
int age;
double average;
};
Student student;
student.name = "Burak";
student.grade= 'A';
student.age= 30;
student.average= 4.76;
Classes are similar tu structs but with more capabilities. CLasses allows us to encapsulate the data and also provide more capability for object oriented programming. We can also use access modifiers with classes.
class Square {
private:
double edge;
public:
double area() {
returnedge * edge;
}
};
Square square;
square.edge = 100;
With enums we can create symbolic names collection of for some specifiers. enums are declaring with integers internally.
enum Color { RED, GREEN, BLUE };
Color selectedColor= GREEN;
With string data type we can store and process the char sequences. It is one of built-in data type of C++ Standart library.
std::string text = "Hello Thecodeprogram";
std::cout << text << endl;
Vector class is an array class to store and process same types of data. Vector arrays can grow or shrink as the size needed by the elements are trying to be added or removed. To use vector class we need to include the vector library first.
//Inclide the library
#include <vector>
// initialise the vector
std::vector<int> nums = {1, 2, 3, 4, 5};
A map is the data structure which allow us to store and process data by the key-value pairs. Keys should be uniqu in a map and we are allowed to reach the values by the keys of the map. Map data structure is coming with the standart library of C++. To use map we need to include the map library first, and then we are good to use the data structure.
//Inclide the library
#include <map>
// initialise the map
std::map<int, std::string> words;
// Set the key value pairs
words[0] = "The";
words[1] = "Code";
words[2] = "Program";
In C++ we can also define our own data types. C++ is allowing us to create our own classes and we can define our own data types with classes and we can define the behaviours as we required. We can encapsulate some internal fields or manipulate them specifically as we needed,
class Rectangle{
public:
int width, height;
Rectangle(int width, int height) : width(width), height(height) {
// Initialisation implementation of the custom class
}
double area(){
return width * height;
}
};
Rectangle rect(10, 20);
std::cout << rect .area() << endl;
Conclusion
Data types are the building blocks of any program. Without data types we can not organise the data. With variables we are managing the data and processing them. For efficient memory usages we need to be sure that we are using appropraite data type for the related operation. So understanding data types is very important in any programming language.
That is all for this article.
Burak Hamdi TUFAN
Comments