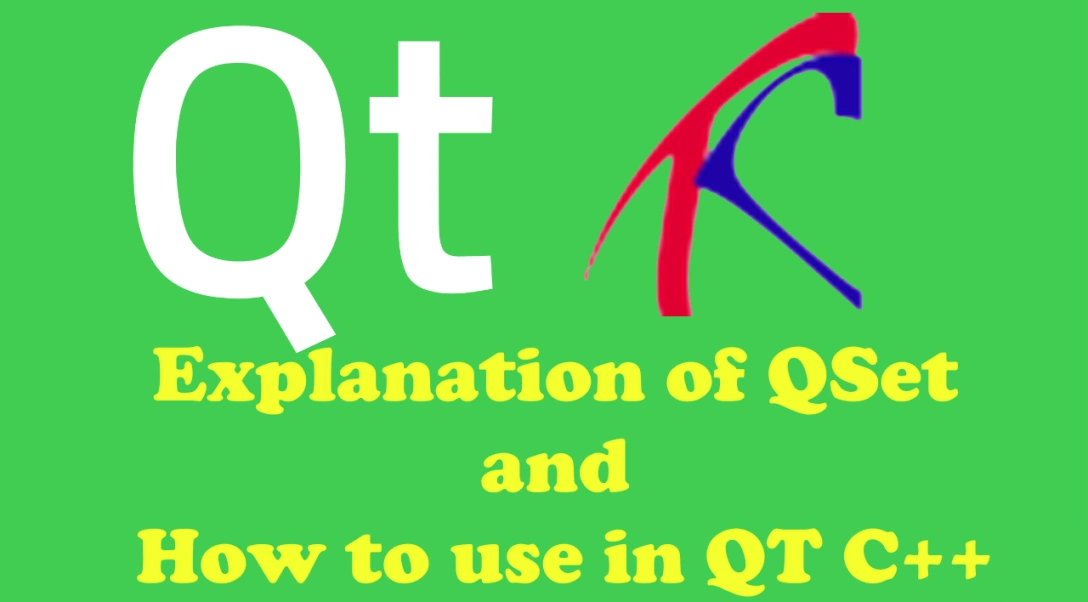
Explanation of QSet and How to use in QT C++
Hello everyone, in this article we are going to talk about Set Data structure and QSet implementation in QT Framework and C++. We are going to talk about structure and make some examples.Let' begin.
First of all we need to know why we need to use Set. To understand better of set container class we need to know why we are in need to use Set class.
Set is a one of most used generic container class which store unique datas inside of array. We can discover the intersection of two dataset during the trying to add some datas in a Set structure. If related data is exist in Set structure, it will ot allow us to insert data. Set structure is an unordered container type.
Now we are good to go for QSet class in Qt.
QT += core
In the class which we are going to use Set Container we need to include QSet library:
#include <QSet>
#include <QSetIterator>
#include <QList>
We need to use insert(T data) method to add adata in container. We can also use << operator.
QSet<QString> set;
set.insert("The");
set.insert("Code");
set.insert("Program");
foreach (QString el, set)
cout << el.toLatin1().constData() << endl;
cout << endl;
QSet<QString> set1;
set1 << "Burak" << "Hamdi" << "TUFAN";
foreach (QString el, set1)
cout << el.toLatin1().constData() << endl;
Program output will be like below
The
Program
Code
Burak
Hamdi
TUFAN
We can also convert our set container to QList by toList() and values() methods. Below code block you can see the example.
QList<QString> setList = set.toList();
for(int i=0; i<setList.size();i++)
cout << setList.at(i).toLatin1().constData() << endl;
QList<QString> setListVals = set.values();
for(int i=0; i<setListVals.size();i++)
cout << setListVals.at(i).toLatin1().constData() << endl;
Program çıktısı aşağıdaki gibi olacaktır
Program
The
Code
Program
The
Code
We are need to use size() method to return element count of related set container. size() method returns an integer value.
int size = set.size();
int size1 = set1.size();
cout << "Size : " << size << endl;
cout << "Size1 : " << size1 << endl;
Output will be like below
Size : 3
Size1 : 3
We need to use empty() method to get the container is empty or nt. It will return a boolean value, I mean true or false.
cout << "Is container empty : " << set.empty() << endl;
set.clear();
cout << "Is container empty : " << set.empty() << endl;
Program Output will be like below. Normally true equals 1 and false equals 0. Results are according to this definition.
Is container empty : 0
Is container empty : 1
Sometimes we may need to know do two containers contains same values. To control this we need to use intersects( QSet<T> ) method. As you can see below we are controlling the sets are intersecting and we are printing the results.
QSet<int> numbers1, numbers2;
numbers1 << 1 << 2 << 3;
numbers2 << 4 << 5 << 6;
cout << "Numbers intersects : " << numbers1.intersects(numbers2) << endl;
numbers1 << 4 << 5;
cout << "Numbers intersects : " << numbers1.intersects(numbers2) << endl;
Output:
Numbers intersects : 0
Numbers intersects : 1
Also if we need the intersecting elements we need to use intersect( QSet<T> ) method. It will return another QSet container which consist of intersecting elements.
QSet<int> intersectList = numbers1.intersect(numbers2);
foreach (int el, intersectList)
cout << "Intersecting element : " << el << endl;
Output:
Intersecting element : 5
Intersecting element : 4
So what is we want to remove intersecting elements from one of QSet container. In this case we are going to use subtract( QSet<T> ) method
QSet<int> n1, n2;
n1 << 1 << 2 << 3 << 4 << 5;
n2 << 4 << 5 << 6;
QSet<int> subtracktedSet = n2.subtract(n1);
foreach (int el, subtracktedSet)
cout << "Rest element : " << el << endl;
Output
Rest element : 6
Now Take a look at the iterating of QSet structure. In here we are going to use begin() and end() methods which return element iterators. We will start to print from begin until the end of the container
QSet<QString> sets;
sets << "The" << "Code" << "Program" << "Burak" << "Hamdi" << "TUFAN";
QSet<QString>::iterator i;
for (i = sets.begin(); i != sets.end(); ++i){
cout << (*i).toLatin1().constData() << " ";
}
Output
TUFAN Hamdi The Program Code Burak
As you can see above we talked about almost all of functionality of the Set containers in the Qt framework.
You can reach the example codes on Github via : https://github.com/thecodeprogram/QSetExample_Thecodeprogram
Burak Hamdi TUFAN
Comments