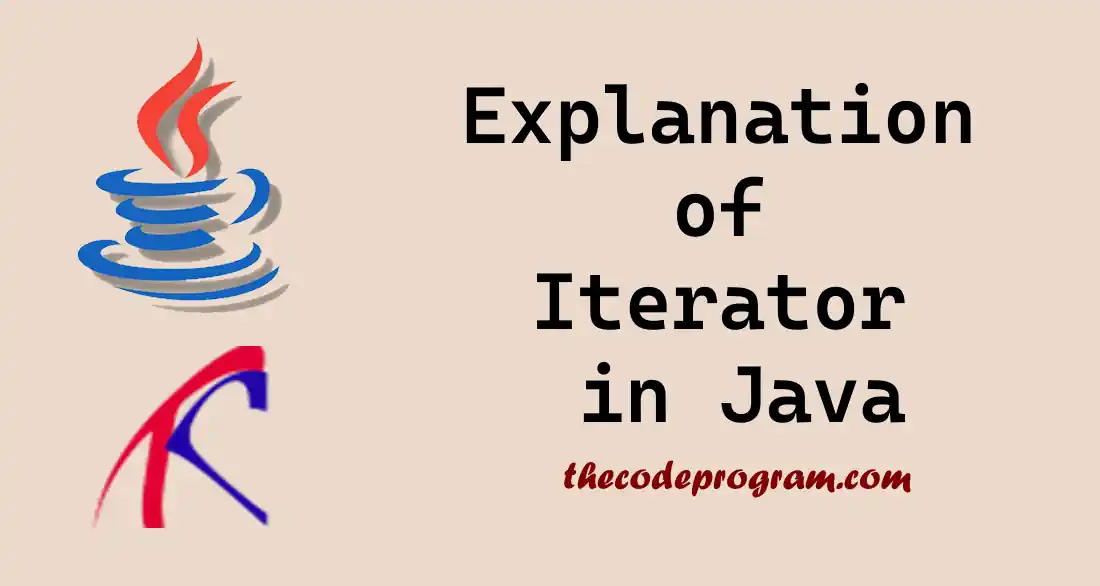
Explanation of Iterator in Java
Hello everyone, in this article we are going to talk about Iterator Interface which is one of most important concept for traversing containers is in Java help of examples.Let's get started...
Iterators are one of most important interfaces for Java containers. Iterator is basically support us to traverse the collection and help us to organise the data in it. We can iterate all collections with same logic and no needs to expose what type of collection that we are using. We can iterate ArrayList, LinkedList, HashSet, Vector and all the other collections are able to be used by Iterator.
We can traverse the list with an iterator. We can check if the next element is exist with hasNext(), and we can get it with next() methods. Below you can see an example for this.
import java.util.*;
public class IteratorExample {
public static void main(String[] args) {
// Initialize a list
List<String> list = Arrays.asList("the", "code", "program");
// Initialise Iterator for the above List Collection
Iterator<String> it = list.iterator();
// Traverse the list via Iterator
while (it.hasNext()) {
String val = it.next();
System.out.println(val);
}
}
}
Program output will be like below
the
code
program
We can also check and remove unwanted values from a Collection via Iterator remove method. Below you can see an example about how to use.
import java.util.*;
public class IteratorExample {
public static void main(String[] args) {
// Initialize a list
List<Integer> list = new ArrayList(Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9));
// Initialise Iterator for the above List Collection
Iterator<Integer> it = list.iterator();
// Iterate the list and remove even numbers
while (it.hasNext()) {
Integer i = it.next();
if(i % 2 == 0){
it.remove();
}
}
// print the collection
list.stream().forEach(number -> System.out.println(number));
}
}
Program output will be like below
1
3
5
7
9
When using remove method of iterator there is an important point about initialising of the List that you will iterate. If you initialise the list like below you will get java.lang.UnsupportedOperationException: remove error.
// Problematic way for remove method
List<String> list = Arrays.asList("the", "code", "program");
The reason is Arrays.asList returns an array that wrapped by a List interface. remove method of iterator directly tries to remove the element from an array as they do to ArrayList but it is not possible since we can not remove element from an Array directly. Also we can not remove an item from a List since it is an interface, so it will throw the java.lang.UnsupportedOperationException: remove error. To fix this obstacle we can initialize it within an ArrayList, or any other required collection as we did in above example
// Better way to initialise
List<Integer> list = new ArrayList(Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9));
And lastly we can also invoke a method for all the elements within collection through an iterator with forEachRemaining(...) method. Now lets see an example about how to use it.
import java.util.*;
public class IteratorExample {
public static void main(String[] args) {
// Initialize a list with an ArrayList wrapper
List<String> list = new ArrayList(Arrays.asList("the", "code", "program"));
// Initialise Iterator for the above List Collection
Iterator<String> it = list.iterator();
// invoke a method for all elements
it.forEachRemaining((val) -> {
System.out.println(val);
});
}
}
When using forEachRemaining you should be carefull for traversing. Because when you iterated the collection, it will not re-iterate the collection again since they are already iterated. So if you need to traverse and use these values for an event you may need to think about of changing the implementation.
Conclusion
Iterators one of very important interface for traversing and organising the data of collections in Java. With Iterator we can directly access the data within collection without think what class is the collection. It makes easier to work with different type of collection types.
That is all for this article.
Have a good iterating.
Burak Hamdi TUFAN
Comments