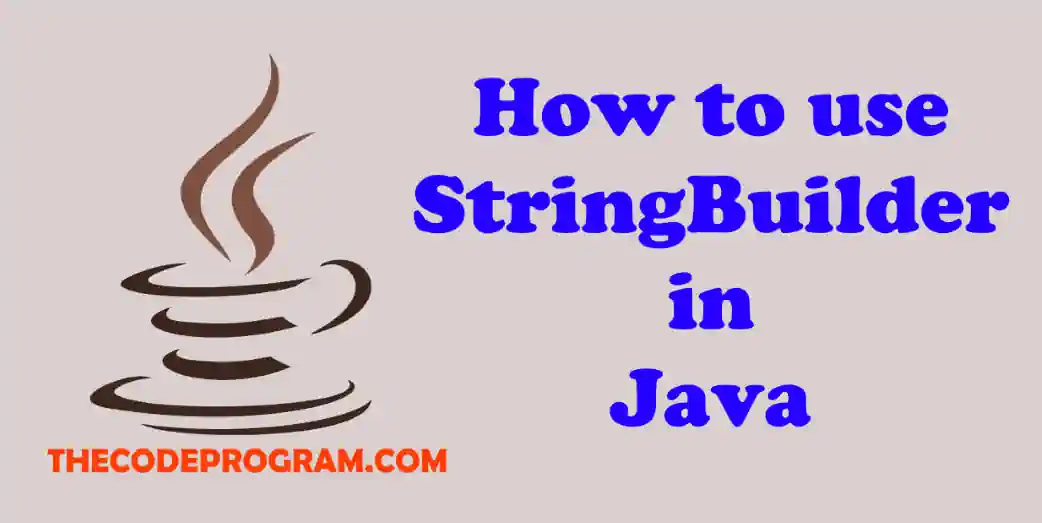
How to use StringBuilder in Java
Hello everyone, in this article we are going to talk about StringBuilder classes in Java language. We are going to talk about the specifications one by one on examples.Let's get started.
public void stringBuilderConstructors(){
StringBuilder sb = new StringBuilder();
StringBuilder sb1 = new StringBuilder("Thecodeprogram String");
StringBuilder sb2 = new StringBuilder(10);
StringBuilder sb3 = new StringBuilder(cs);
}
CharSequence cs = new CharSequence() {
//...
// DO NOT FORGET THE OVERRIDE METHODS...
//...
};
After initialization we are going to use the StringBuilder methods. Below you can find the most commonly methods of StringBuilder class.
HERE SOME MOST COMMON METHODS OF STRINGBUILDER
StringBuilder append(X x)
This Method inserts a text to ending of specified string. This method also overloaded with String, char, boolean int... parameter types. X is the identifier, x parameter.
StringBuilder sb= new StringBuilder("TheCodeProgram");
sb.append(" is the best :). Since : ");
sb.append(2015);
sb.append(" Yes it is ");
sb.append(true);
System.out.println(sb.toString());
Example Output :
TheCodeProgram is the best :). Since : 2015 Yes it is true
StringBuilder insert(int offset, String s)
This method is inserts a text at specified location. We specify the location with offset parameter for this method. This method also averloaded with different types of text parameters like insert(int, char), insert(int, boolean), insert(int, int), insert(int, float), insert(int, double).
StringBuilder sb= new StringBuilder("TheCodeProgram");
sb.insert(3, " great ");
System.out.println(sb.toString());
sb.insert(0, "Number ");
sb.insert(7, " one, ");
System.out.println(sb.toString());
Example Output
The great CodeProgram
Number one, The great CodeProgram
StringBuilder reverse()
This method reverse the given string within StringBuilder.
StringBuilder sb= new StringBuilder("TheCodeProgram");
sb.reverse();
System.out.println(sb.toString());
Example Output:
margorPedoCehT
int capacity() This method return capacity of the StringBuilder. If you did not specialized the capacity during initialisation it will return the current capacity value .
StringBuilder sb= new StringBuilder("TheCodeProgram"); // It return current capacity not 14.
System.out.println("First Text Capacity : " + sb.capacity() );
sb.append(" - Burak Hamdi TUFAN");
System.out.println("Second Text Capacity : " + sb.capacity() );
Example Output
First Text Capacity : 30
Second Text Capacity : 62
int length() This method returns the character count of text within StringBuilder.
StringBuilder sb= new StringBuilder("TheCodeProgram");
System.out.println("First Text Lenght : " + sb.length());
sb.append(" - Burak Hamdi TUFAN");
System.out.println("Second Text Lenght : " + sb.length() );
Example Output
First Text Lenght : 14
Second Text Lenght : 34
String substring(int beginIndex) This method return the substring text from specified index. This method also overloaded with endindex value. If we send parameter with end index it will return between them.
StringBuilder sb= new StringBuilder("TheCodeProgram");
System.out.println("First Substring : " + sb.substring(3));
System.out.println("Second Substring : " + sb.substring(3, 7));
Example Output:
First Substring : CodeProgram
Second Substring : Code
char charAt(int index) This method returns the character at the index which we specified.
StringBuilder sb= new StringBuilder("TheCodeProgram");
System.out.println("Forth Char : " + sb.charAt(3));
System.out.println("Eighth Char : " + sb.charAt(7));
Example Output:
Third Char : C
Seventh Char : P
StringBuilder delete(int startIndex, int endIndex) and deleteCharAt(int index) This methods accomodate the deleting something from text. With delete method: we delete a text between two indexes and also if we want to delete just a character we use deleteCharAt method.
//Delete specified character
StringBuilder sb= new StringBuilder("TheCodeProgram");
sb.deleteCharAt(0);
System.out.println(sb.toString());
//Delete characters between two indexes
sb= new StringBuilder("TheCodeProgram");
sb.delete(3, 7);
System.out.println(sb.toString());
//Delete Last n characters
sb= new StringBuilder("TheCodeProgram");
sb.delete(sb.toString().length() - 7, sb.toString().length());
System.out.println(sb.toString());
Example Output :
heCodeProgram
TheProgram
TheCode
StringBuilder replace(int startIndex, int endIndex, String str) This method replaces the string with given string between two indexes. If we want to apply this replacement we can give indexes as 0 and StringBulder.lenght.
//Replace text between indexes
StringBuilder sb= new StringBuilder("TheCodeProgram");
sb.replace(3, 7, "XXXX");
System.out.println(sb.toString());
//Replace the entire text
sb= new StringBuilder("TheCodeProgram");
sb.replace(0, sb.length(), "BurakHamdiTUFAN");
System.out.println(sb.toString());
Example Output:
TheXXXXProgram
BurakHamdiTUFAN
public void replaceSpecifiedString(StringBuilder sb, String findString, String replaceString){
while(sb.indexOf(findString) > 0){
sb.replace(sb.indexOf(findString), sb.indexOf(findString) + findString.length(), replaceString);
}
System.out.println(sb.toString());
}
All we need to call above method like below:
String findString = "Code";
String replaceString = "Burak";
StringBuilder sb= new StringBuilder("TheCodeProgramCodeCodeProgram");
//Calling the method
replaceSpecifiedString(sb, findString,replaceString);
Example Output:
TheBurakProgramBurakBurakProgram
That is all in this article.
Have a good String Building.
I wish you all healthy days.
Burak Hamdi TUFAN.
Comments