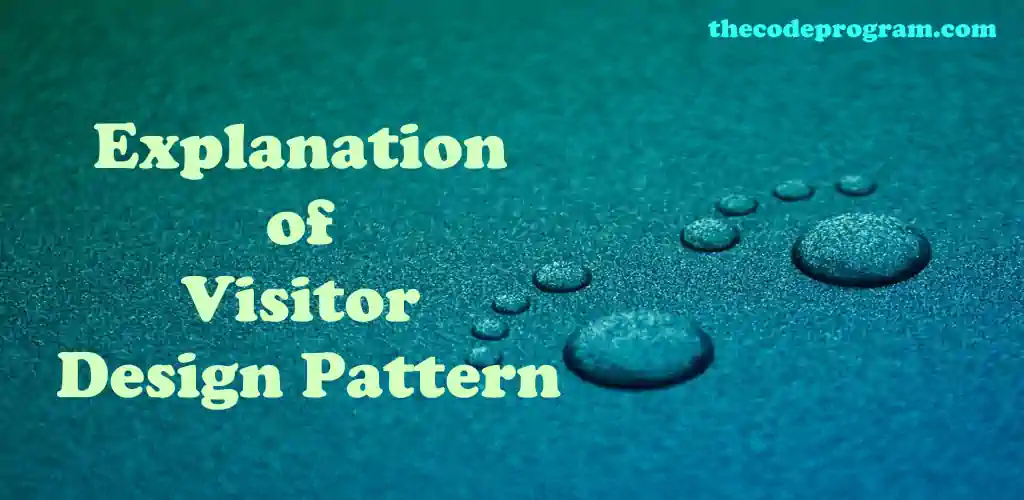
Explanation of Visitor Design Pattern
Hello everyone, in this article we are going to talk about Visitor Design Pattern within Behavioral Design Patterns. We will talk first on its description then make an example in C# and Visual Studio.Let's get started.
This design patern is complex a little bit. So let's start to talk on an example directly.
In here first I will create my Feature interface and then derive the features from this interface. Below code blocks you will see the feature interface and feature classes.
IFeature.cs
namespace VisitorPattern_Example
{
public interface IFeature
{
void EnableFeature(IAircraft aircraft);
}
}
IFE.cs
using System;
namespace VisitorPattern_Example
{
class IFE : IFeature
{
public void EnableFeature(IAircraft aircraft)
{
if (aircraft is B777)
{
Console.WriteLine("IFE is enabled");
}
else if (aircraft is B777F)
{
Console.WriteLine("IFE is not required for Cargo Aircrafts...");
}
}
}
}
CargoCooling.cs
using System;
namespace VisitorPattern_Example
{
class CargoCooling : IFeature
{
public void EnableFeature(IAircraft aircraft)
{
if (aircraft is B777)
{
Console.WriteLine("Cargo Cooling is enabled");
}
else if (aircraft is B777F)
{
Console.WriteLine("Corgo Cooling is not required for Cargo Aircrafts...");
}
}
}
}
Now I will create an interface for concrete classes and then I will derive the concrete classes from this interface. Below you will see the interface and the other concrete classes which derived from this interface.
IAircraft.cs
namespace VisitorPattern_Example
{
public interface IAircraft
{
void ActivateFeature(IFeature features);
}
}
B777.cs
using System;
namespace VisitorPattern_Example
{
class B777 : IAircraft
{
public B777()
{
Console.WriteLine("B777 is initializing...");
}
public void ActivateFeature(IFeature features)
{
features.EnableFeature(this);
}
}
}
B777F.cs
using System;
namespace VisitorPattern_Example
{
class B777F : IAircraft
{
public B777F()
{
Console.WriteLine("B777F is initializing...");
}
public void ActivateFeature(IFeature features)
{
features.EnableFeature(this);
}
}
}
All of configurations are ready. Now I will initialize the concrete classes and belonged features from our program main function.
Program.cs
using System;
namespace VisitorPattern_Example
{
class Program
{
static void Main(string[] args)
{
Console.Title = "Visitor Design Pattern Example - Thecodeprogram";
//Features Arrasy
IFeature[] listFeatures = { new IFE(), new CargoCooling() };
//Concrete classes to initialize class and features
B777 _b777 = new B777();
for (int i = 0; i < listFeatures.Length; i++)
{
_b777.ActivateFeature(listFeatures[i]);
}
Console.WriteLine("-----------------------------------------");
B777F _b777f = new B777F();
for (int i = 0; i < listFeatures.Length; i++)
{
_b777f.ActivateFeature(listFeatures[i]);
}
Console.ReadLine();
}
}
}
In this way the all concrete classes and the features classes have been loosen linked and we can add new features without any changing in any other classes.
That is all in this article.
You can reach the example application on Github via : https://github.com/thecodeprogram/VisitorPattern_Example
Have good visiting the classes
Burak Hamdi TUFAN
Comments