Explanation of Template Design Pattern
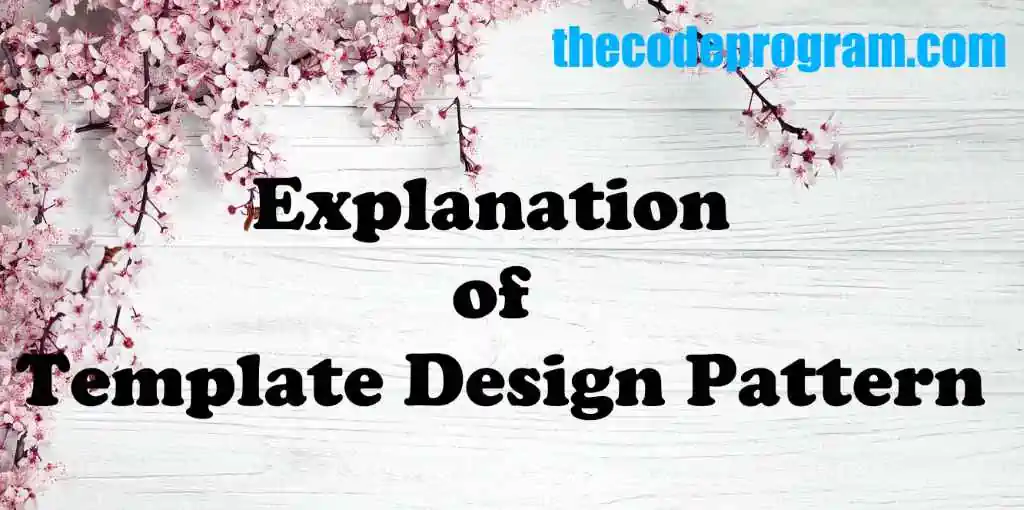
Let's begin.
Before we start the example you should take a look at below article. I used somethings within this article : Type Class and typeof Keyword and GetType in C#
In our example we are going to make different types of soups. Almosts every soups have same mixtures : Tomatoes, paste, onions and water. The other elements are changing according to kind of soups.
First I have to create my abstract class. In this class I will have my abstract and virtual methods to get a soup ready. After I will derive soup classes from this abstract class.
And last I have a method which perform all required tasks.
Soup.cs
using System;
namespace TemplatePattern_Example
{
public abstract class Soup
{
//My abstract methods
public abstract void AddMaterials();
public abstract void CookSoup();
//My virtual method
public virtual void AddSpecialMaterial(string specialMaterial)
{
Console.WriteLine("The Special material " + specialMaterial + " for " + GetType().Name + " has been added");
}
//My get Ready method.
public void GetSoupReady()
{
AddMaterials();
CookSoup();
Console.WriteLine(GetType().Name + " is ready... Enjoooy :)");
Console.WriteLine("-----------------------------" + Environment.NewLine);
}
}
}
Now I need to derive the sub classes which perform the specific operations. Below code blocks you will see my sub classes.
TomatoSoup.cs
using System;
namespace TemplatePattern_Example
{
class TomatoSoup : Soup
{
public override void AddMaterials()
{
Console.WriteLine("Adding Essential Materials for Tomato Soup...");
}
public override void CookSoup()
{
Console.WriteLine("Tomato Soup is cooking...");
}
}
}
ChickenSoup.cs
using System;
namespace TemplatePattern_Example
{
class ChickenSoup : Soup
{
public override void AddMaterials()
{
Console.WriteLine("Adding Essential Materials for Chicken Soup...");
//I also add some other materials except the main materials
AddSpecialMaterial("Delicious Chicken meat");
}
public override void CookSoup()
{
Console.WriteLine("Chicken Soup is cooking...");
}
}
}
EzoGelinSoup.cs
using System;
namespace TemplatePattern_Example
{
class EzoGelinSoup : Soup
{
public override void AddMaterials()
{
Console.WriteLine("Adding Materials for EzoGelin Soup...");
//I also add some other materials except the main materials
AddSpecialMaterial("Lentils");
}
public override void CookSoup()
{
Console.WriteLine("EzoGelin Soup is cooking...");
}
}
}
OnionSoup.cs
using System;
namespace TemplatePattern_Example
{
class OnionSoup : Soup
{
public override void AddMaterials()
{
Console.WriteLine("Adding Materials for Onion Soup...");
}
public override void CookSoup()
{
Console.WriteLine("Onion Soup is cooking...");
}
}
}
Program.cs
using System;
namespace TemplatePattern_Example
{
class Program
{
static void Main(string[] args)
{
TomatoSoup tomSoup = new TomatoSoup();
tomSoup.GetSoupReady();
ChickenSoup chickSoup = new ChickenSoup();
chickSoup.GetSoupReady();
EzoGelinSoup ezoSoup = new EzoGelinSoup();
ezoSoup.GetSoupReady();
OnionSoup onionSoup = new OnionSoup();
onionSoup.GetSoupReady();
Console.ReadLine();
}
}
}
That is all in this article.
You can reach the example on Github : https://github.com/thecodeprogram/TemplatePattern_Example
Now you are good to use the Template Design pattern in your projects.
I wish you healthy days.
Burak Hamdi TUFAN.