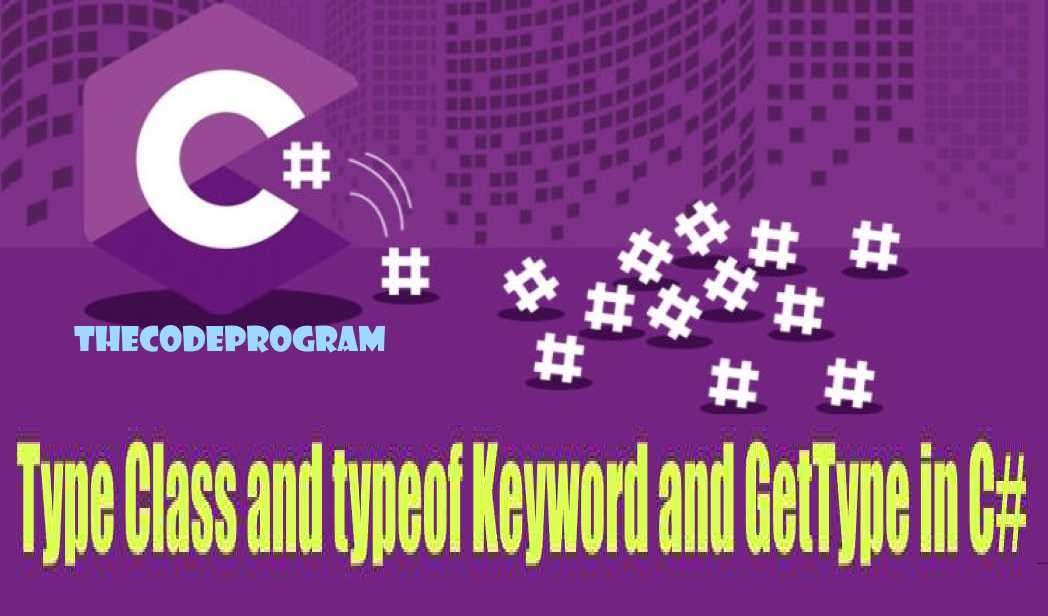
Type Class and typeof Keyword and GetType in C#
Hello everyone, in this article we are going to talk about typeof and GetType() in C# programming language. We are going to make an example in C# and Visual Studio.Let's begin.
We can access the variable and class Type's with this class. Also we can reach the properties of the user defined class and its members.
To make example first we create a Class like below.
Declare the properties of the user defined class and Declare the constructor and lastly declare an example free method.
ın this class I declared the properties string, int and bool type variables. And then I will create the constructor to set the properties and lastly we declare an empty method.
namespace typeOf_Example
{
class Aircraft
{
//Declare the properties of the user defined class
public string Brand { get; set; }
public string Model { get; set; }
public int releaseYear { get; set; }
public bool stillInService { get; set; }
//Declare the constructor
public Aircraft(string _brand, string _model, int _releaseYear, bool _stillInService)
{
this.Brand = _brand;
this.Model = _model;
this.releaseYear = _releaseYear;
this.stillInService = _stillInService;
}
//declare an example free method.
public void exampleMethod()
{
}
}
}
And then will make the required operations in main program. You can see it below:
Fist I will declare the below namespaces: The first one is essential for the program operations. System namespace is required. The reflection namespace is To use MemberInfo data type we should declare the Reflection namespace.
using System;
using System.Reflection;
In the Main class First I will declare the required variables and the user defined class to use these methods.
string brand = "Boeing";
string model = "777-200";
int releaseYear = 1999;
bool stillInService = true;
Aircraft ac = new Aircraft(brand, model, releaseYear, stillInService);
And then we check the user defined class type and its single property datas and print it on the console.
First let's a take a look at user defined class properties and its members type properties. Here we declared a class named Aircraft and we are going to make some controls.
//First let's a take a look at user defined class properties
//and its members type properties.
//Here we declared a class named Aircraft and we are going to make some controls
Console.WriteLine("Aircraft class and members properties: ");
Console.WriteLine("Aircraft is a " + ac.GetType());
Console.WriteLine("Aircraft Member Example : ");
Console.WriteLine("-Brand variable is a " + ac.Brand.GetType());
Console.WriteLine("-Brand variables FullName is " + ac.Brand.GetType().FullName);
Console.WriteLine("-Brand variables Namespace is " + ac.Brand.GetType().Namespace);
We use the member info array and we use the class properties. Then we will print all of the properties via for loop.
First we need to declare a MemberInfo array and load this array with class properties.Then we print all members with a for loop.
Console.WriteLine(Environment.NewLine + "User Defined class Methods");
//First we need to declare a MemberInfo array and load this array with class properties.
MemberInfo[] ac_methods = ac.GetType().GetMethods();
//Then we print all members with a for loop.
for (int i = 0; i < ac_methods.Length; i++)
{
Console.WriteLine("--" + ac_methods[i].Name + " is a "+ ac_methods[i].MemberType);
}
And then we are going to print all properties of the user defined class.
Here we see the usage of typeof with typeof we can check the type of a variable and we can make some operations if the related type is specified. Here first we declare a Type variable and we set it as type properties. Then we use a foreach to print all of them first.
//Here we see the usage of typeof with typeof we can check the type of a variable and
//we can make some operations if the related type is specified.
Console.WriteLine(Environment.NewLine + "User Defined class properties");
//Here first we declare a Type variable and we set it as type properties.
//Then we use a foreach to print all of them first.
Type ac_type = ac.GetType();
foreach (var s in ac_type.GetProperties())
{
Console.WriteLine(s.Name + " " + s.PropertyType );
}
Also we can filter the types. If you want to select the one type you can select it. In here I will print only string values. Here we use typeof method to make it.
Also we can filter the types of the class. And we will write here only strings type of the properties.
//Also we can filter the types of the class.
Console.WriteLine(Environment.NewLine + "only string type Defined class properties");
//And we will write here only strings type of the properties.
foreach (var item in ac_type.GetProperties())
{
if (item.PropertyType == typeof(string))
{
Console.WriteLine(item.Name + " " + item.PropertyType);
}
}
You can find the example application on github via https://github.com/thecodeprogram/typeof_example
That is all in this article.
Have a good typing of :).
I wish you all healty days.
Burak Hamdi TUFAN.
Comments