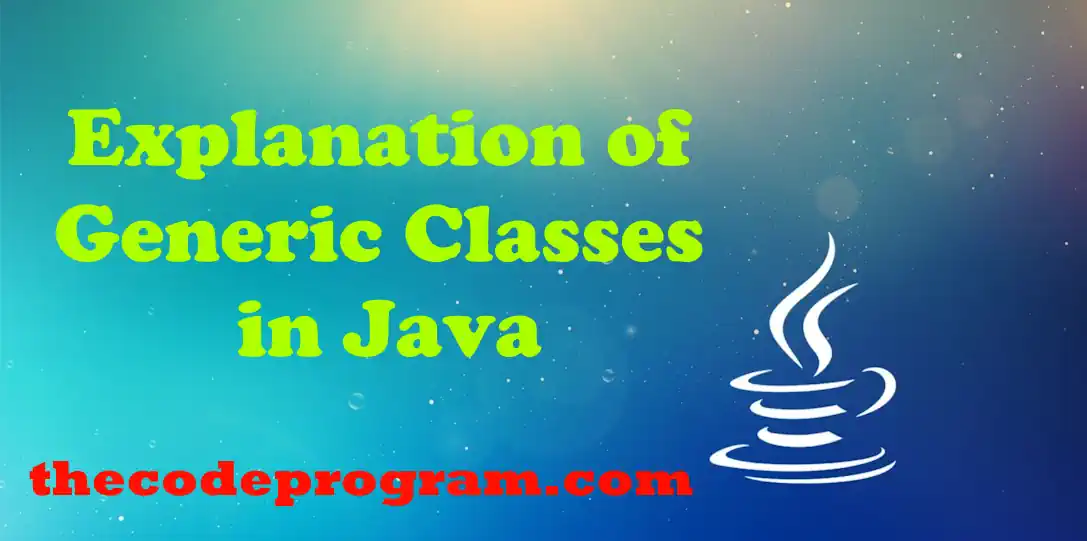
Explanation of Generic Classes in Java
Hello everyone, in this article we are going to talk about Generic classes in Java programming language with code examples for better understanding.Lets get started.
Generic classes are the one of the efficient way to create structures to work with different types of data with safe development. It is an efficient way to create flexible and reusable classes and interfaces in Java.
Understanding Generic Classes
Generic classes allow us to create interfaces, classes and some other structures which is able to take objects as a parameter to work with different types of object with same implementation with keeping type safe implementation. Generic classes are improving the reusable code implementation with more clean and maintainable way. Generally we can see the generic classes usages with Collections (List, Vector, Set, Map...) in Java.
Now lets dive in how to implement a Generic Class in Java.
class GenericClassExample {
public static class TheGeneric<T> {
private T value;
public TheGeneric(T value) {
this.value = value;
}
public T getValue() {
return value;
}
public void setValue(T value) {
this.value = value;
}
}
public static void main(String[] args) {
TheGeneric<Integer> generic1 = new TheGeneric<>(12345);
TheGeneric<String> generic2 = new TheGeneric<>("Thecodeprogram");
TheGeneric<Boolean> generic3 = new TheGeneric<>(false);
System.out.println(generic1.getValue());
System.out.println(generic2.getValue());
System.out.println(generic3.getValue());
}
}
Program Output will be like below
12345
Thecodeprogram
false
As you can see above we have defined our class with generic identifier in angled brackets after the class definition. Inside the class we added some functions to handle the generic object which is represented by the generic identifier instance. In the main function we have just initialised the Generic Class and used same method for all of data types to get the value which is wrapping by the Generic class.
Now, we know how to create a generic class, Now lets create some generic methods.
class GenericClassExample {
public static <T> void printIndividual(T data){
System.out.print(data + " ");
}
public static <T> void printGenericArray(T[] array) {
for (T el : array) {
printIndividual(el);
}
System.out.println();
}
public static void main(String[] args) {
Integer[] numbers = { 10, 20, 30, 40, 50 };
String[] strings = { "the", "code", "program" };
printGenericArray(numbers);
printGenericArray(strings);
}
}
As you can above code block we have declared two methods which are working with Generic data types and we have called them with the different types of data to print the datas.
Conclusion
Generics are efficient feature of Java that allows us to create more flexible and clean classes to work with different data types. Generics are allowing us to create more reusable and clean codes with type safety when working with different types of data. Usage of the Generics will improve the performance of the program and the maintainability of the codebase.
That is all
Burak Hamdi TUFAN
Comments