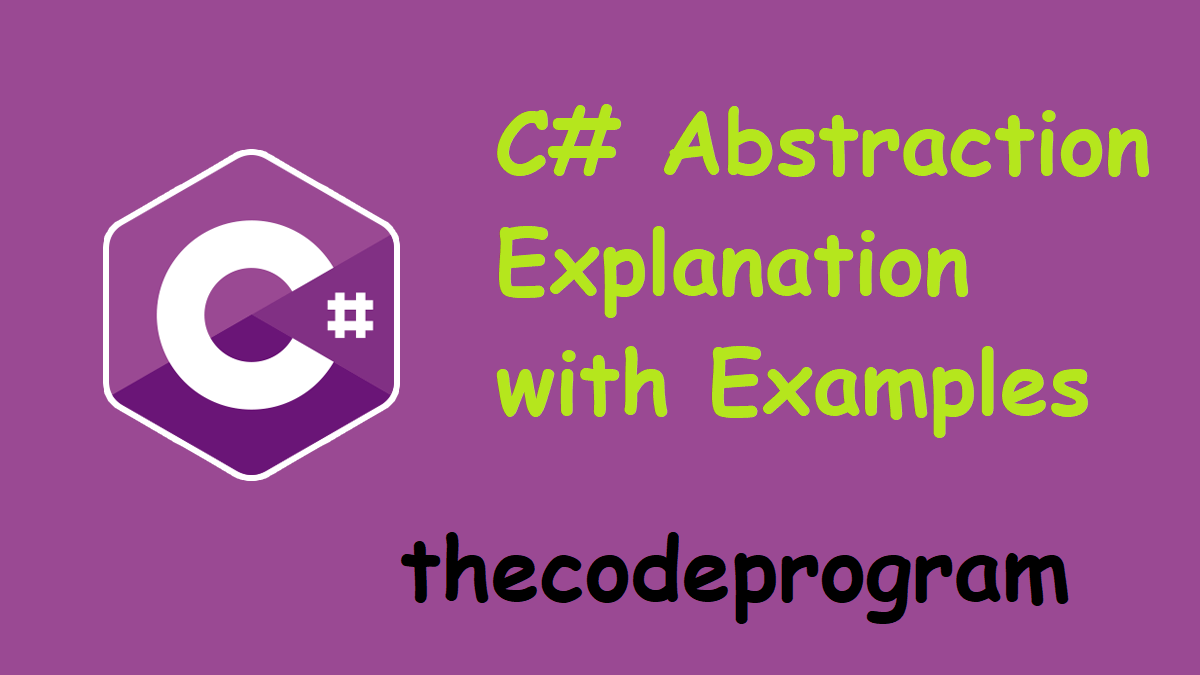
C# Abstraction Explanation with Examples
Hello everyone, in this article we are going to talk about Object oriented programming Abstraction specification. ı will use C# programming language to make example. Here we are going to create classes to use it on our form and we are going to make some calculations on these classes.Let's get started then.
What is Abstract Class?
Abstract classes are the base of other classes. Abstract Class contains common functions which used at other classes. Purpose of abstract classes is being base for the other classes not for deriving new classes. So you can not derive objects from abstract class.
What is abstract method?
When derive new classes from these abstract classes, we have to override functions which defined as abstract method in class. We just define the function in the class body as abstract and we will not write the function body. Abstract functions have to be overrided at the derived classes.
After project creation first I recommend you to create a folder. Clean code methodology requires to be tidied up. And so I use the folders If there are so many class files to keep them as groupped.
First Add a folder in your project and right click on the folder then add a class inside this folder. Below images you can follow how can you do this.
abstract class Vehicle
{
//Properties of the all vehicles
public string Owner = "";
public string Brand = "";
public Color Color;
public int maxSpeed = 0;
}
abstract class Vehicle
{
//Properties of the all vehicles
public string Owner = "";
public string Brand = "";
public Color Color;
public int maxSpeed = 0;
//Construction Class
public Vehicle(string _Owner, string _Brand, Color _Color, int _maxSpeed)
{
//I will set all required values when initialized
Owner = _Owner;
Brand = _Brand;
Color = _Color;
maxSpeed = _maxSpeed;
}
}
And also if you want to update these values after initialization, you can do it just making equalization from the class where it used. Because we defined it as public and other class can reach and update them.
Also we will use the abstract methods and override methods. At the below code block we will define an abstract method, later we will call that function at our derived class. At that derived class we create a new function as protected override and the same name with the abstract function.
abstract class Vehicle
{
//Properties of the all vehicles
public string Owner = "";
public string Brand = "";
public Color Color;
public int maxSpeed = 0;
//Abstract methods
//Abstract methods will have no body here
public abstract void setYear();
//Construction Class
public Vehicle(string _Owner, string _Brand, Color _Color, int _maxSpeed)
{
//I will set all required values when initialized
Owner = _Owner;
Brand = _Brand;
Color = _Color;
maxSpeed = _maxSpeed;
}
}
Our abstract class is ready. Now we will make our Car class is derived from our abstract class and we will use it at our program.
//I have created a folder named Vehicles and I have a class named Vehicle
//I derived this class from that class
class Car : Vehicles.Vehicle
{
public int tyreCount = 4;
//We need to implement construction of he class
public Car(string _Owner, string _Brand, Color _Color, int _maxSpeed) : base(_Owner, _Brand, _Color, _maxSpeed)
{
}
//We have to override below abstract method
public override void setYear()
{
throw new NotImplementedException();
}
public void setColor()
{
}
}
We will use these classes from main windows' class. Below code block will do it.
using CSharp_OOP.Vehicles;
private void Form1_Load(object sender, EventArgs e)
{
//Here we initialized the with the required values of the class
Car c = new Car("Burak Hamdi TUFAN", "Mercedes", Color.Black, 280);
//Called a function that defined at Car class
c.setColor(Color.Yellow);
//Bring a value of class
MessageBox.Show("The owner of the car is : " + c.Owner);
}
That is all in this article.
Have a good abstraction.
Burak Hamdi TUFAN
Comments