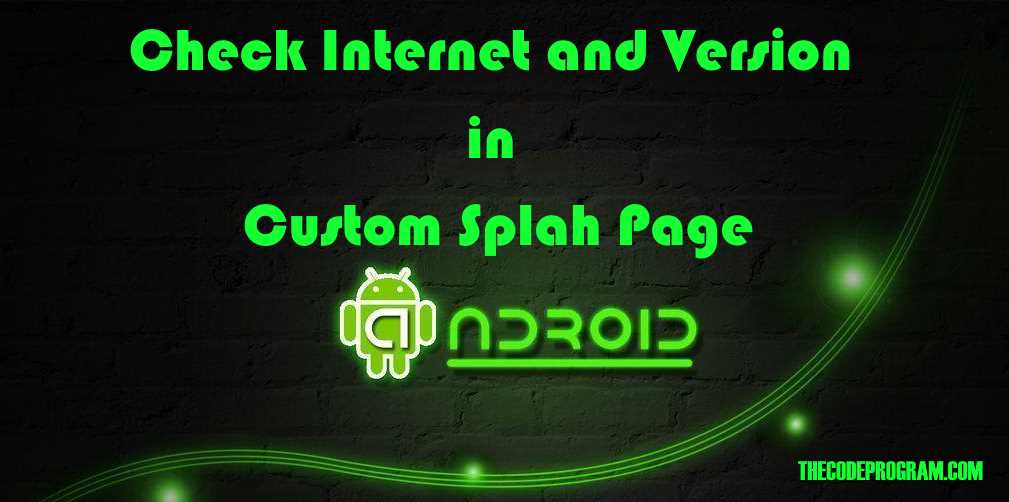
Check Internet and Version in Custom Splah Page in Android
Hello everyone, in this article we are going to talk about building a Splash Screen and load the required datas for the next activy in android and we are going to make an example on it.Let's begin.
Fist create a project with empty activity and this activty name will be MainActivity and it will be default activty. After it we will add another empty activty and wil are going to name it as splash_page. After it we are going to make it as default activity via AndroidManifest file. Below image you can see it:
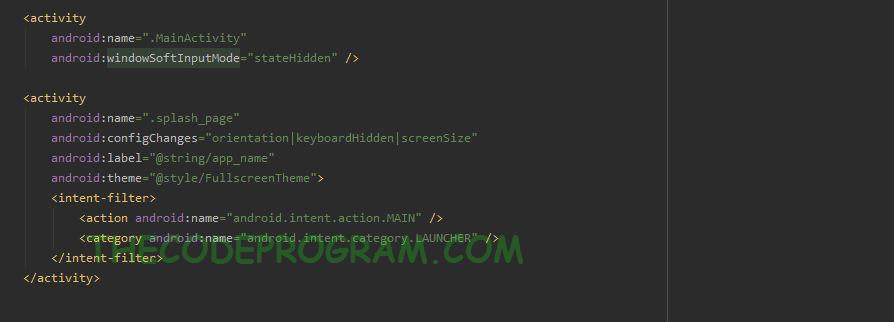
As you can see the above image I also set the theme of the activity as full screen. Below code block you can see the my FullScreen theme. I do not want any action bar so I removed it.
<style name="FullscreenTheme" parent="AppTheme.NoActionBar">
<item name="android:actionBarStyle">@style/FullscreenActionBarStyle</item>
<item name="android:windowActionBarOverlay">true</item>
<item name="android:windowBackground">@null</item>
<item name="metaButtonBarStyle">?android:attr/buttonBarStyle</item>
<item name="metaButtonBarButtonStyle">?android:attr/buttonBarButtonStyle</item>
</style>
And below you can see the my splash_page xml design.
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#0099cc" >
<FrameLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/splash_Back"
android:foregroundGravity="center_vertical|center_horizontal"
android:gravity="center_vertical|center|center_horizontal"
android:orientation="vertical"
android:weightSum="1">
<ImageView
android:layout_width="150dp"
android:layout_height="150dp"
android:src="@drawable/tcp" />
<TextView
style="?metaButtonBarButtonStyle"
android:textColor="@color/colorPrimary"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:text="@string/author_name"
android:layout_weight="0.01" />
</LinearLayout>
<LinearLayout
android:id="@+id/fullscreen_content_controls"
style="?metaButtonBarStyle"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="bottom|center_horizontal"
android:background="@color/black_overlay"
android:orientation="vertical"
tools:ignore="UselessParent">
<TextView
android:id="@+id/textView2"
android:layout_width="match_parent"
android:layout_height="40dp"
android:background="@color/colorPrimary"
android:text="@string/author_creator_name"
android:textStyle="bold"
android:textSize="20sp"
android:foregroundGravity="center_vertical|center_horizontal"
android:gravity="center_vertical|center|center_horizontal"
android:fontFamily="cursive"
android:textColor="@color/white" />
<TextView
android:background="@color/colorPrimary"
android:textColor="@color/white"
android:foregroundGravity="center_vertical|center_horizontal"
android:gravity="center_vertical|center|center_horizontal"
android:layout_width="match_parent"
android:layout_height="25dp"
android:text="@string/author_site"
android:fontFamily="sans-serif-smallcaps"/>
</LinearLayout>
</FrameLayout>
</FrameLayout>
Below function will check the connection state and returns connection acquired or not.
public boolean has_internet_connection() {
final ConnectivityManager conMgr = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
final NetworkInfo activeNetwork = conMgr.getActiveNetworkInfo();
if (activeNetwork != null && activeNetwork.isConnected()) {
return true;
} else {
return false;
}
}
And also I will get the version of the installed program and i will make a comparisation according to the value which located at server. And if the installed program is under the required program version we will show a message to update the program.
Below function will get the programcurrent version number.
public int getProgramVersion() {
int version = 0;
try {
version = getPackageManager().getPackageInfo(getPackageName(), 0).versionCode;
} catch (PackageManager.NameNotFoundException e) {
}
return version ;
}
Now our codes are ready, At onCreate of the we are going to wait a little bit to show the splash screen and then we are going to make internet connection and program version check from the server. If there is no problem after all we are going to start the MainActivity.
Below code block first we declared a Timer and we started that timer with 200miliseconds delay. Purpose of this to show our beatiful splash screen of the author of the program. In this timer we called programStart function. This function will check the internet connection status of the device and if there is no connection a message box will appeared which specify the connection state. Else if the internet connection acquired it will connect to the server to get the program version from server. At all if the user have to update the application redirec to the market, if there is no need to update it will start the MainActivity.
Below code block will do it.
public class splash_page extends AppCompatActivity {
private Timer myTimer;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_splash_page);
getWindow().setFlags(WindowManager.LayoutParams.FLAG_FULLSCREEN, WindowManager.LayoutParams.FLAG_FULLSCREEN);
//Start a timer with 2 seconds delay. This timer will work after 2 seconds
myTimer = new Timer();
myTimer.schedule(new TimerTask() {
@Override
public void run() {
programStart();
}
}, 2000);
}
private void programStart(){
if (has_internet_connection() == false) {
AlertDialog.Builder alertDialog = new AlertDialog.Builder(splash_page.this);
alertDialog.setTitle("There is no internet connection");
alertDialog.setMessage("To use this program you have to connect internet first!");
alertDialog.setPositiveButton("Cancel"), new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,int which) {
finish();
}
});
alertDialog.setNegativeButton("Try Again"), new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
finish();
startActivity(getIntent());
}
});
alertDialog.setCancelable(false);
alertDialog.show();
}
else {
isCorrectVersion();
}
}
private void isCorrectVersion(){
String url = String.format("the service url to get program version");
String program_version = "0";
StringRequest postRequest = new StringRequest(Request.Method.GET, url,
new Response.Listener<String>() {
@Override
public void onResponse(String response){
try {
object = new JSONObject(response);
JSONArray client_inf = object.getJSONArray("client");
program_version = client_inf.getJSONObject(0).getString("version");
if(program_version == String.valueOf(getProgramVersion())){
Intent intent = new Intent(splash_page.this,MainActivity.class);
startActivity(intent);
}
else{
final AlertDialog.Builder alertDialog = new AlertDialog.Builder(splash_page.this);
alertDialog.setTitle("Update Required");
alertDialog.setMessage("You have to update the program first");
alertDialog.setPositiveButton("Go update page"), new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,int which) {
final String appPackageName = getPackageName();
startActivity(
new Intent(Intent.ACTION_VIEW,
Uri.parse("market://details?id=" + appPackageName))
);
}
});
alertDialog.setNegativeButton(getResources().getString("Cancel the updating"),
new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
}
});
alertDialog.setCancelable(false);
alertDialog.show();
}
} catch (JSONException e) {
e.printStackTrace();
}
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
error.printStackTrace();
}
});
Volley.newRequestQueue(this).add(postRequest);
}
}
That is all in this article.
Have a good building your custom splash screens.
I wish You all healthy days.
Burak Hamdi TUFAN
Comments