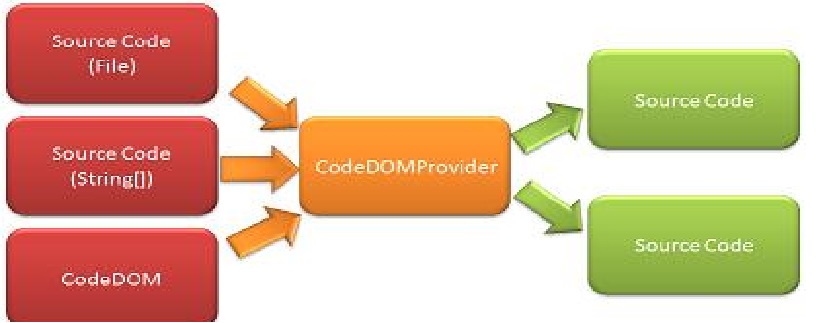
Codedom Library in C# - Code entegration into Executable
In this article, I will tell you about C# language and CodeDom library. With this library, I will explain how to change the code structure within the software or to change a field we want.I'd like to say this. This process is completely legal and not a hacking process. This library allows you to change the code in your programs by windows via the execurable file.
First of all, what is Codedom and how it works:
It is a library used to change and rewrite a code field that we know in the program. By means of a program written with this library, we find and modify the variables or functions we want in the source code through the exe file of another program, and create a modified program by rewriting.
We need to add the following library to the program we will use this library.
Using System.CodeDom;
There are several articles available with this library, but they are articles based on re-code generation. In this article we will replace the existing code and reprint the program.
We will first search the code block for which we will perform our operations, and then make changes to them.
Now let's start writing. First, let's write a simple console application and then change the codes of this console application.
class TheCode
{
public void print()
{
System.Console.WriteLine("The Code Program");
}
}
static void Main( string[] args )
{
TheCode the= new TheCode();
the.print();
Console.ReadLine();
}
//we have created a simple class and function
//this program will prints The Code Program on screen
Now that we've written the simple program above, let's move on to the program we're going to change.
Here we will create the code that we write in the program as if it were a string expression, and when it is found we will replace it with a string expression.
- CodeCompiler object: Is the object we use to recompile the newly created code. We will create and compile new code with child objects of this object. Then you'll get new exe.
- With the CSharpCodeProvider object, we will return the assembly code in C # language and print the new code as Assembly.
- With the CompilerParameters object, we will create and send the parameters that will work with the new codes that we print to our program.
- With the CompilerResults object, which is the child object of the CompilerSource object, we will recompile the parameters that we have recently sent into the program and the assembley code that we created with the Provider object above. In this way, we will return our newly created program.
With the following code block, we have written a function to compile the program and generate assembly codes. This function will return the code expressions entered in the form of assembly.
private static Assembly CompileSource( string kaynak_kod )
{
CodeDomProvider cpd = new CSharpCodeProvider();//Created code provider
CompilerParameters cp = new CompilerParameters(); //created object to send parameters
cp.ReferencedAssemblies.Add("System.dll"); //created referance library
cp.ReferencedAssemblies.Add("benim_class.dll"); //added a class that I have created
cp.GenerateExecutable = true; //setted to compile program
CompilerResults cr = cpd.CompileAssemblyFromSource(cp, kaynak_kod);
// created a programs assembly
return cr.CompiledAssembly;//returned created assembly
}
Since we write the function that creates the assembly codes above, we need to use this function in order. We will call and use the above function via the function below.
namespace create_assembly_code
{
class Program
{
static void Main( string[] args )
{
create();
}
private static void create()
{
string my_source_code= @"
class TheCode
{
public void print()
{
System.Console.WriteLine("The Code Program");
}
}";
Assembly assembly_code = CompileSource(my_source_code);
object new_code = assembly_code .CreateInstance("the code written here will be changed");
}
}
}
When we run the above code, we replace the function we wrote at the top with the field we wrote at the bottom. So we change the code to string.
Here the above program will no longer work. Because there will be compilation error. Because calls the print function in TheCode class when the program runs. But there is no longer a function called print in the program. however, it does not exist in a class called TheCode. Because we deleted that data and we put something else in its place..
Our newly created assembly code is an object. Because of that, we called object variable type.
So how do we change the executable program. Let's make an example about this topic and finish our article.
With the following example, we will make a Codedom Application and make changes to the executable entered.
public static bool compile_program(string exe_path, string will_changed_code)
{
CodeDomProvider compiler= CodeDomProvider.CreateProvider("CSharp");
//created compiler and specified programming language
CompilerParameters parameters= new CompilerParameters();
//created parameters
CompilerResults output = default(CompilerResults);
//created output object
parameters.GenerateExecutable = true;
parameters.OutputAssembly = exe_path;
parameters.ReferencedAssemblies.Add("System.dll");
parameters.CompilerOptions = " /target:winexe";
parameters.TreatWarningsAsErrors = false;
// compiling parameters specified
output = compiler.CompileAssemblyFromSource(parameters, will_changed_code);
//sended to compiling
if (output .Errors.Count > 0) //any errors with compiling
{
foreach (var item in output .Errors)
{
MessageBox.Show(item.ToString()); //print error if exist
}
return false;
// and return false
}
else if (output .Errors.Count == 0)
{
return true; if there is no error return true and finish the process
}
return true; //this one has no problem :)
}
We will call the function above and convert the exe we selected and create an exe file again.
Let's call the function as follows. Let's get a form application and do this.
OpenFileDialog dialog = new OpenFileDialog();
dialog.FileName = "";
dialog.Filter = "Files|*.exe|DLL Files|*.dll";
if (dialog.ShowDialog() == DialogResult.OK)
{
programi_derle(dialog.FileName.ToString(), "the_code_will_be_hanged");
}
In this way, we re-created our exe file with the codes we prepared and rewrote our program. If you do not receive any compilation errors, the program will compile successfully. And our exe file will re-occur with the code we write.
Let me tell you again, this process is definitely not a hack process. We modify and rebuild the contents of exe files through the libraries provided by Microsoft
So much friends in this article - Stay tuned
Have a nice coding...
Burak Hamdi Tufan
Comments