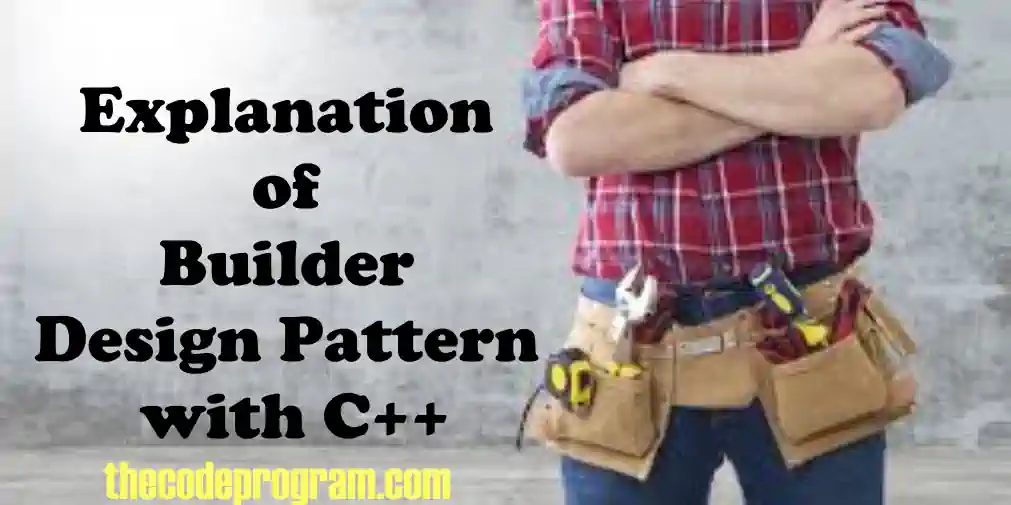
Explanation of Builder Design Pattern with C++
Hello everyone, in this article we are going to talk about one of very famous Design Pattern called as Builder Design Pattern within Creational Design Patters. We are going to make an example with QT Console application.Let's get started.
Firstly, what is Builder Design Pattern
If we have an object which has multiple subobject, a builder class create all of them and prepare the main class with all subclasses. This design pattern is useful when we are working more complex components and its subcomponents. Our Builder class will build the requested component with subparts according to user request and get it ready.Now let's write some code :) We can not live without code :)
I have written all of codes inside the main.c file. So you do not need to create other header files.
First of all we have to include our libraries these are essential.
#include <QCoreApplication>
#include <iostream>
using namespace std;
//Some QT libraries
#include <QString>
#include <QTextStream>
#include <QStringList>
Here first we are in need of a product which may contain many different types of sub components like an aircraft
class Aircraft{
public:
QStringList partlist;
void ListAircraftParts() const{
for(int i = 0; i < partlist.size(); i++){
cout << partlist.at(i).toStdString() << endl;
}
}
};
Then we need a builder class interface. This interface will derive the class to produce the products sub components according to user requests.
class Builder{
public:
virtual ~Builder(){}
virtual void ProduceEngines() const =0;
virtual void ProduceSeats() const =0;
virtual void ProduceIFE() const =0;
};
This class also known as ConcreteBuilder class. Product implementations are going to be realised through this Productor class. I will use only one Productor class but if you want you can use several types of Productor classes.
class AircratProductor : public Builder{
private:
Aircraft* aircraft;
public:
AircratProductor(){
this->aircraft= new Aircraft();
}
~AircratProductor(){
delete aircraft;
}
void ProduceEngines()const override{
this->aircraft->partlist.append("Engines");
}
void ProduceSeats()const override{
this->aircraft->partlist.append("Passenger Seats");
}
void ProduceIFE()const override{
this->aircraft->partlist.append("In Flight Entertainment System");
}
Aircraft* GetAircraftBuiltAircraft() {
Aircraft* res= this->aircraft;
this->aircraft= new Aircraft();
return res;
}
};
NOTE
We are initializing the product at constructorAll producing steps will be defined here and they are all will be called according to user request.
Returns the built producted aircraft and here reset the productor for a new production with initial configurations
Here our manager class. This class is responsible for managing the production. This class is capable of working with different builders, As I mentioned above there can be more builder classes and we should manage them through just one manager class.
class Manager{
private:
Builder* builder;
public:
Manager(Builder* _builder)
{
this->builder=_builder;
}
~Manager()
{
delete builder;
}
void BuildLowCost(){
this->builder->ProduceEngines();
}
void BuildMediumCost(){
this->builder->ProduceEngines();
this->builder->ProduceSeats();
}
void BuildFullandFull(){
this->builder->ProduceEngines();
this->builder->ProduceSeats();
this->builder->ProduceIFE();
}
};
IN HERE
We can work with different type of builders So we have to set it before we start to production. I decided to set it at constructorWe are going to set the production configuration through Manager class. We will tell the manager what we need and it will produce.
Now last part of the article.
First we have to initialize the productor and our manager with this productor
Then Program will ask the user the requested type of aircraft and produce according to user input
Lastly Show the selected aircraft properties and and delete the garbage component when we do not need to prevent memory leaks.
Then Program will ask the user the requested type of aircraft and produce according to user input
Lastly Show the selected aircraft properties and and delete the garbage component when we do not need to prevent memory leaks.
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
system("title Factory Design Pattern Example - Thecodeprogram");
cout << "Welcome Builder Design Pattern Example in QT C++" << endl << endl;
program_init:
AircratProductor *productor = new AircratProductor();
Manager *manager = new Manager(productor);
std::cout << "Enter your Requested Aircraft type : ";
QString Request;
QTextStream cin(stdin);
Request = cin.readLine();
if(Request == "low")
{
manager->BuildLowCost();
}
else if(Request == "medium")
{
manager->BuildMediumCost();
}
else if(Request == "full")
{
manager->BuildFullandFull();
}
else if(Request == "exit")
{
return 0;
}
cout << "Part list of Selected Aircraft type" << endl;
Aircraft *producedAircraft = productor->GetAircraftBuiltAircraft();
producedAircraft->ListAircraftParts();
delete producedAircraft;
cout << "-------------------------" << endl;
goto program_init;
return a.exec();
}
That is all in this article.
You can reach the example on Github via : https://github.com/thecodeprogram/TheSingleFiles/blob/master/QBuilderPattern_Example.cpp
Burak Hamdi TUFAN.
Tags
Share this Post
03/04/2020
Explanation of Fuzzy Logic
05/04/2020
Explanation of Facade Design Pattern
20/10/2021
Comments