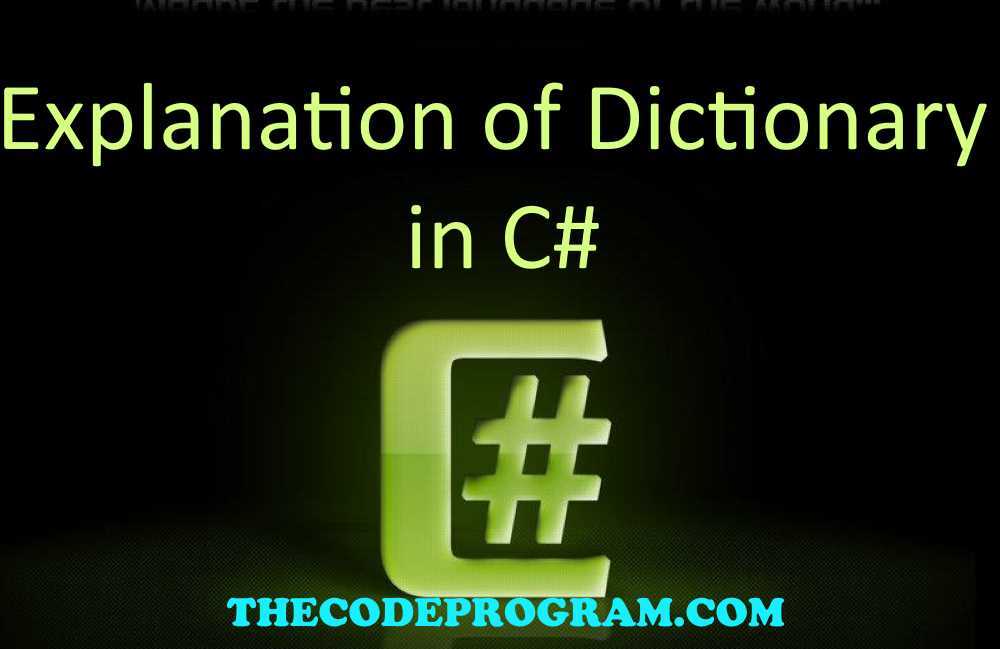
Explanation of Dictionary in C#
Hello everyone, in this article we are going to talk about Dictionary in C#. We will talk what Dictionary is and we will make an example about Dictionary in C# with Visual Studio.Let's get started.
Dictionary is the collection of the key-value pairs. ıt holds the datas with keys and we use them as this. We add the datas with keys and we get the datas with keys. ıt is under the Collection Generic namespace.
When we use the standart arrays we have to set the dimensions of the arrays. The developer can not change it during the normal operation. Sometimes we may need the arrays with dynamic dimensions. In these case we use the Dictionaries. Dictionary has a dynamic dimension and we do not specify the array dimension.
When a value added into the Dictionary, it assigns an index value to the added data automatcally. So the data has both key and index values. This situation allows us to reach with both keys and index.
Now Let's write some code to make it understand.
Firstly we have to insert below namespaces.
using System.Collections.Generic;
using System.Linq;
First we have to declare and initialize a dictionary. In here we will declare five different Dictionaries to show how to initialize the differant types of dictionaries.
Initialization of the differant type of Dictionaries As you can see we declared the dictionary with keys and values. Below four initializations we used four combinations of string and int variable types. Also you can initialize it with interface. You can also declare and initialize the dictionary with the values. Here we initialized the dictionary with values.
//Initialization of the differant type of Dictionaries
//As you can see we declared the dictionary with keys and values.
//Below four initializations we used four combinations of string and int variable types.
Dictionary<string, string> dic = new Dictionary<string, string>();
Dictionary<string, int> dic_int = new Dictionary<string, int>();
Dictionary<int, string> dic_string = new Dictionary<int, string>();
Dictionary<int, int> dic_both_int = new Dictionary<int, int>();
//Also you can initialize it with interface.
IDictionary<string, string> idic = new Dictionary<string, string>();
//You can also declare and initialize the dictionary with the values.
//Here we initialized the dictionary with values.
Dictionary<string, string> dic_with_values = new Dictionary<string, string> {
{ "key1", "value1" }, { "key2", "value2" }, { "key3", "value3" }
};
To add some data we use Add method which declared in Dictionary. We can add datas directly or with KeyValuePairs. Directly adding is simple. Adding the datas with KeyValuePairs is more simple :) But you can use this with interface declared. You can not use this specification with directly declared with Dictionary.
//To add some data we use Add method which declared in Dictionary
//We can add datas directly or with KeyValuePairs.
//Directly adding is simple
dic.Add("key", "value");
dic.Add("web", "https://thecodeprogram.com");
dic_int.Add("int_value", 33);
//Adding the datas with KeyValuePairs is more simple :)
//But you can use this with interface declared.
//You can not use this specification with directly declared with Dictionary.
KeyValuePair<string, string> key_valuePair = new KeyValuePair<string, string>("string_key", "string_value");
idic.Add(key_valuePair);
After we can remove the datas from the Dictionaries. We can remove data with keys. Also we can remove datas with KeyValue Pairs through indexes.
//We can remove data with keys.
dic.Remove("key");
//Also we can remove datas with KeyValue Pairs through indexes.
idic.Remove(new KeyValuePair<string, string>("string_key", "string_value"));
Also we can search some datas from the Dictionary. We use the contains. We can search between the keys and between the values.
We can also make the searchment with the keys and datas. To search some key in the keys we will use the ContainsKey method and we use theContainsValue to search in the values.
//We can also make the searchment with the keys and datas.
//To search some key in the keys we will use the ContainsKey method
//and we use theContainsValue to search in the values.
if (dic_with_values.ContainsKey("key1")) Console.WriteLine("Key1 is exist");
if (dic_with_values.ContainsKey("thekey")) Console.WriteLine("thekey is not exist.");
if (dic_with_values.ContainsValue("value1")) Console.WriteLine("value1 is exist");
if (dic_with_values.ContainsValue("thevalue")) Console.WriteLine("thevalue is not exist.");
You can find the example application on Github via : https://github.com/thecodeprogram/Dictionary_Example
That is all in this article.
I wish you all healthy days.
Have a good using dictionary.
Burak Hamdi TUFAN
Comments