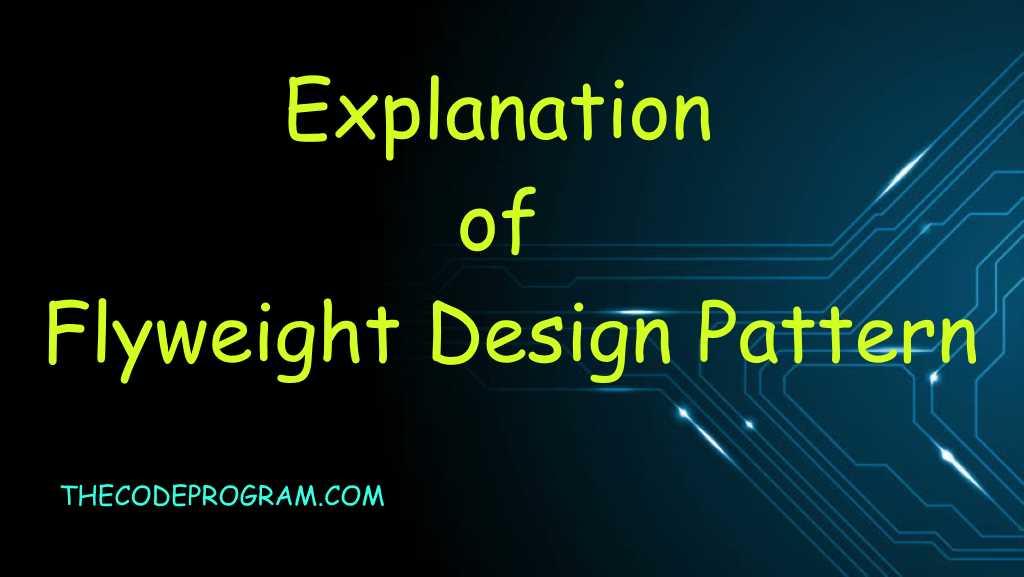
Explanation of Flyweight Design Pattern
Hello everyone, in this article we are going to talk about Flyweight Design pattern within Structural Design Patterns. We will talk on where can be used and how can we use it, after we will make an example about it.Let's begin now.
We use Flyweight design pattern for saving system resources and increasing the performance of the application. We will use the declared variable again and again instead of redeclaring so many variables or objects. We will use the same interface or abstract class for all same type classes to derive them and we will use these related classes through the same instrict specifications.
First We should know two specification about Flyweight:
- Intrinsic state: The specifications which belong to the class and this class keep it.
- Extrinsic state:The specifications which add and share by external classes.
Here I will make an example about File and folder selector application. Here we will create an abstract class to derive file and folder with the same specifications and we will show them.
FileFolder.cs
namespace FlyweightPattern_Example
{
abstract class FileFolder
{
//These are the extrinct states of the model
//These states will be configured from out of related class
protected string Name;
protected int lenght;
protected string modifiedDate;
//This is instrict state of the model
//This variable will be setted from inside the model
protected string type;
//I want this function to show to user every model which derived from here
public abstract void show();
}
}
FileModel .cs
using System;
namespace FlyweightPattern_Example
{
class FileModel : FileFolder
{
public FileModel(string _name, int _lenght, string _modifiedDate)
{
//extrict states
Name = _name;
lenght = _lenght;
modifiedDate = _modifiedDate;
//instrict state
type = "File";
}
public override void show()
{
Console.WriteLine("##########################");
Console.WriteLine("This is a File : ");
Console.WriteLine("Name : " + Name);
Console.WriteLine("lenght : " + lenght + " bytes");
Console.WriteLine("modifiedDate : " + modifiedDate);
Console.WriteLine("type : " + type);
}
}
}
FolderModel.cs
using System;
namespace FlyweightPattern_Example
{
class FolderModel : FileFolder
{
//These are also instrict states of the model
int contentCount;
bool isHidden;
public FolderModel(string _name, string _modifiedDate, int _contentCount, bool _isHidden)
{
//extrict states
Name = _name;
modifiedDate = _modifiedDate;
//instrict state
type = "folder";
this.contentCount = _contentCount;
this.isHidden = _isHidden;
//this will instrict here but in other model will be extrict
//because the folders are always 4096 bytes
lenght = 4096;
}
public override void show()
{
Console.WriteLine("##########################");
Console.WriteLine("This is a folder : ");
Console.WriteLine("Name : " + Name);
Console.WriteLine("lenght : " + lenght + " bytes");
Console.WriteLine("modifiedDate : " + modifiedDate);
Console.WriteLine("type : " + type);
Console.WriteLine("contentCount : " + this.contentCount);
Console.WriteLine("isHidden ? : " + this.isHidden.ToString());
}
}
}
Program.cs
using System;
namespace FlyweightPattern_Example
{
class Program
{
static void Main(string[] args)
{
FileModel file = new FileModel("Thecodeprogram.cs", 774422, DateTime.Now.ToShortDateString());
FolderModel folder = new FolderModel("TheCodeprogram", DateTime.Now.AddDays(-3).ToShortDateString(),9, false);
file.show();
Console.WriteLine("------------------------------");
folder.show();
Console.ReadLine();
}
}
}
That is all in this article.
Have great Flyweight developing.
Burak Hamdi TUFAN
Tags
Share this Post
06/05/2020
Explanation of Interpreter Design Pattern
31/07/2021
Comments