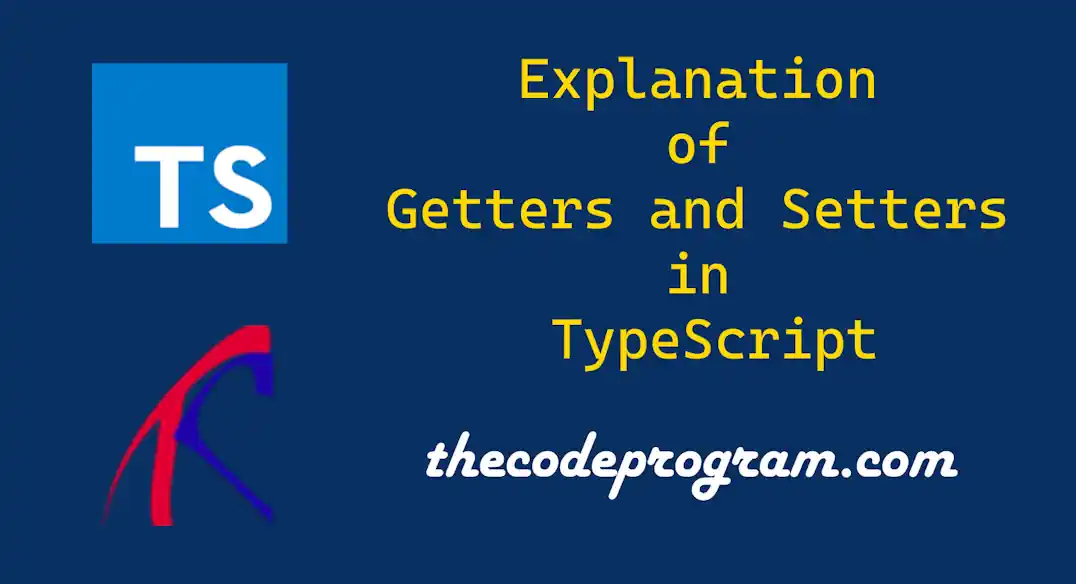
Explanation of Getters and Setters in TypeScript
Hello everyone, in this article we are going to talk about Getter and Setter functions of members in TypeScript programming languages with help of examples.Let's begin
What is getter and setter in Typescript?
Getters and Setters are very efficient features to control the accesses to the properties of a class in TypeScript. We can control the assignee value to the variable and also we can perform some other functionalities on reading or assiging to a variable.
Getter
Getter is a dedicated function in TypeScript to get the value of an internal variable of a class. A getter is defining with get keyword and then the name of function without any parameter.
Below you can see an example of Getter function
class Person {
private _name: string;
private _surname: string;
constructor(name: string, surname: string) {
this._name = name;
this._surname = surname;
}
get name(): string {
return this._name;
}
get surname(): string {
return this._surname;
}
}
const user = new Person("Burak", "Hamdi");
console.log(user.name); // Burak
console.log(user.surname); // Hamdi
As you can see above, We are storing the values within internal private variables and we are getting the values with getter functions. As you can see above we called the get function name without paranthesis and we used only the name of name of get function.
Setter
Setter function is changing the value of a function. In here We can make some validations before setting and also allowing the logging and overriding the information before set, or preventing the setting.
Below you can see an example of Setter function
class Employee {
readonly email_regex: RegExp = /^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,}$/i;
public errorLogs = "";
private _email: string;
constructor(email: string) {
this._email = email;
}
set email(value: string) {
if ( this.email_regex.test(value) ) {
this._email = value;
}
else{
this.errorLogs += "Email setting failed\n";
throw new Error("Email format should be valid.");
}
}
}
const employee = new Employee("abcde@gmail.com");
employee.email = "thecodeprogram"; // Error will be thrown here
Program output will throw error
[ERR]: "Executed JavaScript Failed:"
[ERR]: Email format should be valid.
As you can see above with setter method we have checked the input value with a regular expression and if it is not valid we prevented to assign and throw an Error. Also we have logged the failure.
That is all for getter and setter in Typescript.
Burak Hamdi TUFAN
Comments