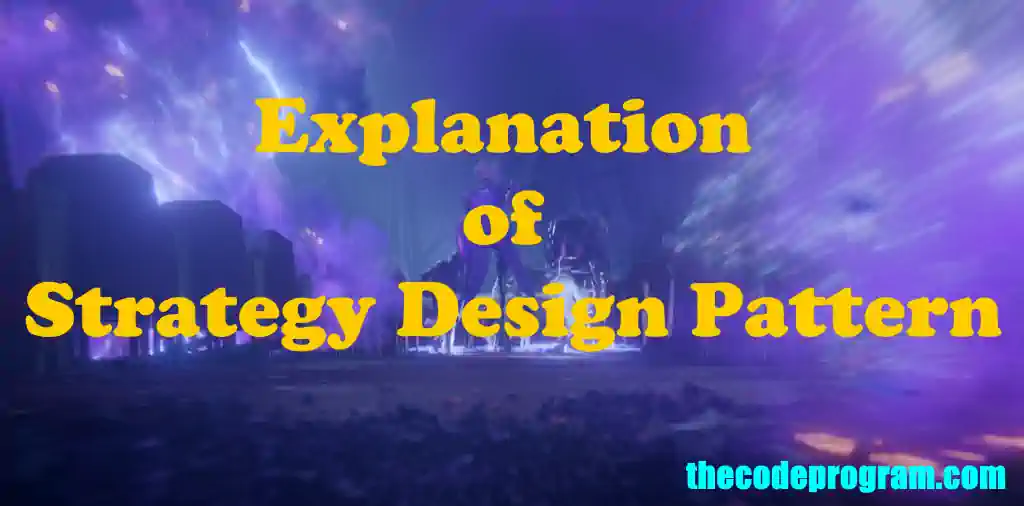
Explanation of Strategy Design Pattern
Hello everyone, in this article we are going to talk about Strategy Design patterns within Behavioral Design Patterns. We will make a simple description and an example in C# language.Let's get started.
Sometimes we may have more than one ways to perform a task in our projects. To accomodate these requirements we may have multiple classes and we call them seperatelly.
Let's write some code now...
First we are going to create an interface which contains the required methods ( behaviours ) for our project.
IEngineStart.cs
/*
Engine start interface will contain the required method for all behaviours
The derived classes will have these methods.
*/
namespace StrategyPattern_Example
{
public interface IEngineStart
{
void StartEngine();
}
}
And now we are going to create our classes. These classes will refer to the behaviours for engine start methods. I will have two different ways to start an engine. First method is the automatic start and the other method is manualStart method. And I will derive these behaviour from above interface.
AutomaticStart.cs
using System;
namespace StrategyPattern_Example
{
class AutomaticStart : IEngineStart
{
public void StartEngine()
{
Console.WriteLine("Engine has started as AUTOMATICALLY");
}
}
}
ManualStart.cs
using System;
namespace StrategyPattern_Example
{
class ManualStart : IEngineStart
{
public void StartEngine()
{
Console.WriteLine("Engine has started as MANUALLY");
}
}
}
Now our methods are ready. In here you can create another class to manage the engine starting process. I will call them from directly Program.cs main function. It depends on your project.
Program.cs
using System;
namespace StrategyPattern_Example
{
class Program
{
static void Main(string[] args)
{
Console.Title = "trategy Design Pattern Example - Thecodeprogram";
//First I will create interface component
IEngineStart engineStart = null;
//And I will get the method selection
Console.WriteLine("Please enter start strategy : ");
string start_procedure = Console.ReadLine();
//Then I will start the engine according to user selection
if (start_procedure == "auto")
{
engineStart = new AutomaticStart();
}
else if (start_procedure == "manual")
{
engineStart = new ManualStart();
}
engineStart.StartEngine();
Console.ReadLine();
}
}
}
That is all in this article.
You can also find the example source code on Github : https://github.com/thecodeprogram/StrategyPattern_Example
Have a good strategies.
Burak Hamdi TUFAN
Comments