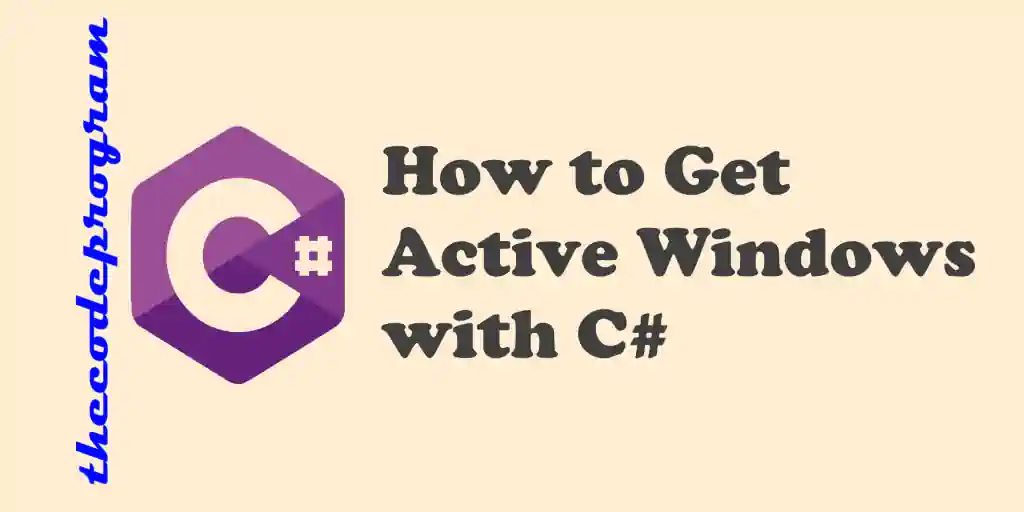
How to Get Active Windows with C#
Hello everyone, in this article we are going to make an example application in C# that will get the title of the active windows at that time and record it time by time.Let's get started.
Firstly we need to add below library in our namespace:
using System.Runtime.InteropServices;
Now define GetForeGroundWindow() and GetWindowsText() from user32.dll inside the class which will be called.
[DllImport("user32.dll")]
static extern IntPtr GetForegroundWindow();
[DllImport("user32.dll")]
static extern int GetWindowText(IntPtr hwnd, StringBuilder ss, int count);
Below code block will get the title of active window. Below method will first get active window and assign it to a pointer variable. Then via this pointer it will get the title text of active window. Then it will return the title as string.
private string ActiveWindowTitle()
{
//Create the variable
const int nChar = 256;
StringBuilder ss = new StringBuilder(nChar);
//Run GetForeGroundWindows and get active window informations
//assign them into handle pointer variable
IntPtr handle = IntPtr.Zero;
handle = GetForegroundWindow();
if (GetWindowText(handle, ss, nChar) > 0) return ss.ToString();
else return "";
}
Now I will see the active windows time by time. To perform this I have created a Timer and in Tick event of this timer, At tick event we have recorded the active titles inside a Listbox with their time values.
public Form1()
{
InitializeComponent();
this.TopMost = true;
Timer tmr = new Timer();
tmr.Interval = 1000;
tmr.Tick += Tmr_Tick;
tmr.Start();
}
private void Tmr_Tick(object sender, EventArgs e)
{
//get title of active window
string title = ActiveWindowTitle();
//check if it is null and add it to list if correct
if (title != "")
{
lbActiveWindows.Items.Add( DateTime.Now.ToString("hh:mm:ss") + " - " + title);
}
}
Run the program and switch between the screens. Then you will see the switched screens inside listbox.
That is all in this article.
You can reach the example project on Github via below link:
https://github.com/thecodeprogram/GetActiveWindow
Burak Hamdi TUFAN
Comments
Hi sir, I want to get all user actions in window such as opening folder, clicking on an application ... How do it ?
2021/06/20 11:08:35This idea is similar to keylogger and I think you can perform it with windows API. But probably, an antivirus program will prevent your application to read the user activities.
2021/06/28 08:28:22