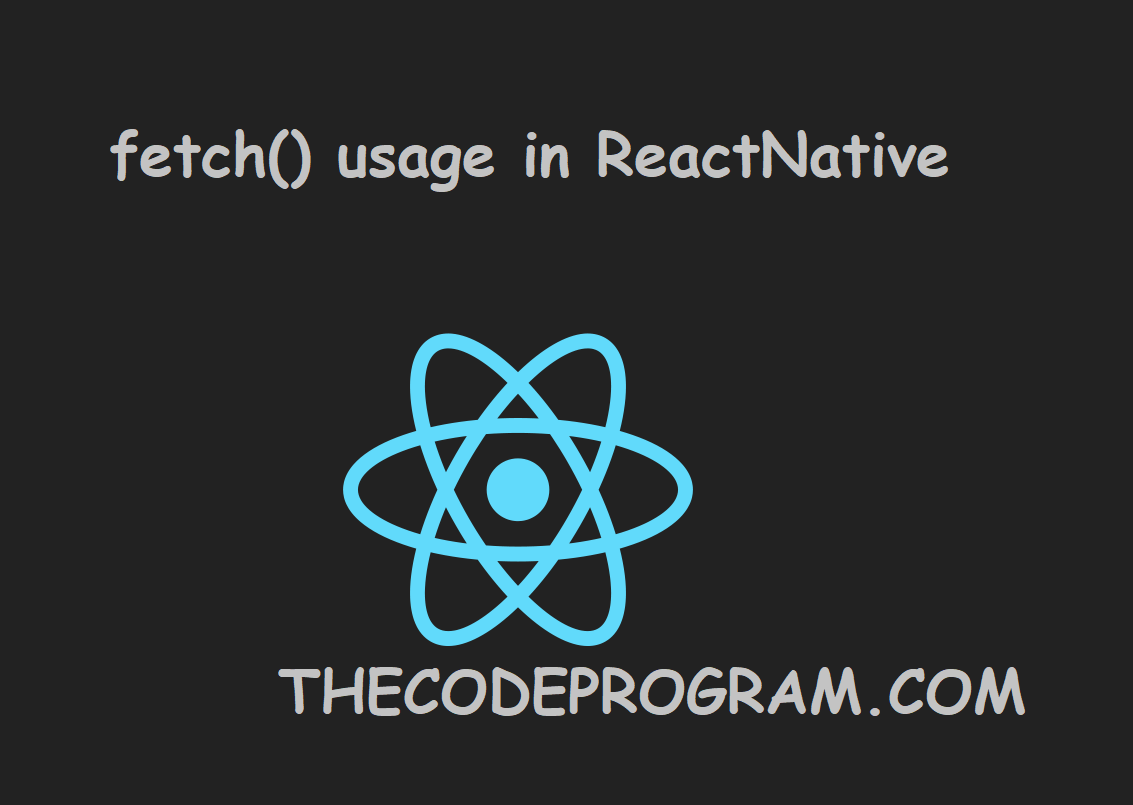
React Native How to use fetch() function
Hello everyone, In this article we are going to talk about how can we use fetch function with react native and we will make some examples on it make it understandable.First I need to explain what does fetch() function does ?
Mobile applications may need to upload resources from a remote URL. We may want to make a POST request to a web API, or you may need to fetch some static content from another server. React Native provides the fetch API for your network needs. If you have previously used XMLHttpRequest or other network APIs, the fetch will be familiar. With this api, you can take JSON or XML type data from your remote server and perform operations in your application.
Pay Attention : Do not forget to Add the INTERNET PERMISSION to your Android Manifest file for Android Devices
Now we are ready to code something about fetch:
fetch('http://thecodeprogram.com/my_service.php');
Above code will only run the URL. That is all. With this code block. But What if want to do more things? I generally use POST method to get something from the urls and send the datas as JSON string. Here take a look at how can we do this ?
fetch('http://thecodeprogram.com/my_service.php', {
method: 'POST',
headers: {
Accept: 'application/json',
'Content-Type': 'application/json',
},
body: JSON.stringify({
parameter1: 'value1',
parameter2: 'value2',
}),
});
The example above have a general usage of fetch function. With method keyword we are specifing the data sending method. Here we used the POST method. We can also use the GET method here. I generally use the POST method. With the headers array we are specifing the header datas of the sending data. Here we are telling the service: our data is a JSON type. This is an optional array. If we do not to send this information we can just send our data and our service will know the incoming data will be a JSON typed data. At last we have body arrayhere. With this array we are sending the content of the sending data. We need to specify what values will we send.
Now What if we need to use the response data from our web service. Now what to do ?
Below code section has an example about how to use fatch function and how to get response data from the web servis. Here we are tking the data as JSON and then equalize them into variables that we will use in our program.
let url = "http://thecodeprogram.com/my_service.php";
let data = {
method: 'POST',
credentials: 'same-origin',
mode: 'same-origin',
body: JSON.stringify({
process: "get",
id: "1",
}),
headers: {
'Accept': 'application/json',
'Content-Type': 'application/json',
}
}
fetch( url, data).then( res => res.json() )
.then( incomingData => {
this.setState( { loading: false } );
//Custom result data for error exception
//I will use this for myself
if( incomingData.result != '0')
{
incomingData= incomingData.result.map( item => {
return {
image: item.image,
text: item.name,
key: item.id,
sub_id: item.id,
id: item.id,
master_id: item.master_cat_id,
height: 50 ,
width: 80,
}
}
);
}
} );
Above code section has an example on how to fetch and process data. First I have declared the url and data sending configuration. I have variables named url and data for this. You can see this at top of the code section. After fetching the data from the service we are using the then function. With this function we are specifing the process what will we do and sending the incoming data inside this function as named incomingData. After a little checking of the incoming data is what I want, I am making my equalizing to use these datas to show on screen.
Now we are ready to use this fetch function in our React Native projects.
That is all in this article.
Have a nice and clean coding.
Burak hamdi TUFAN
Comments