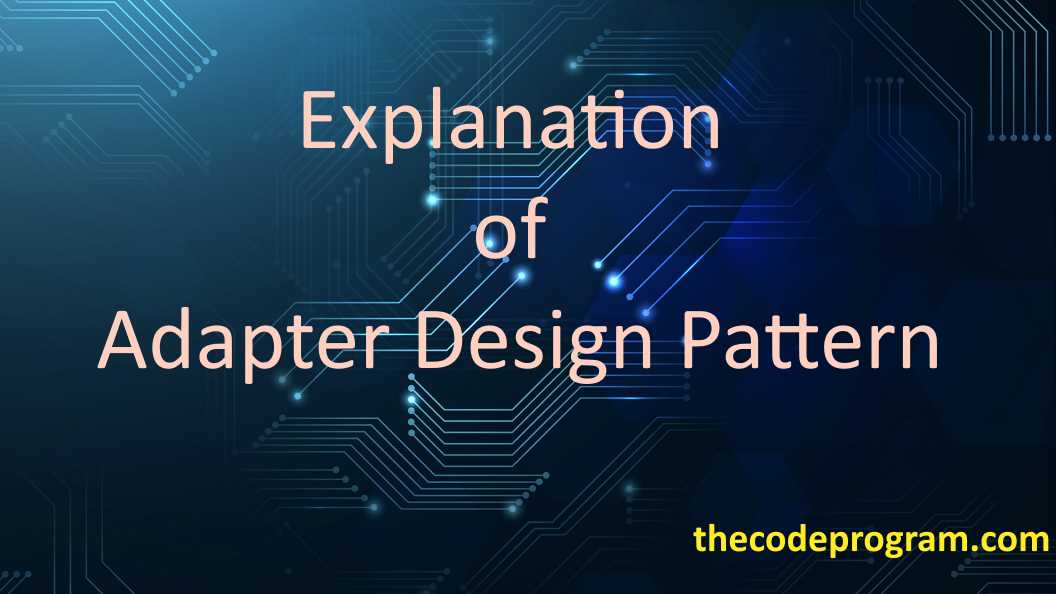
Explanation of Adapter Design Pattern
Hello everyone, in this article we are going to talk about Adapter Design Pattern. We will give some code blocks to make some explanations and examples. How can we use these design patterns.Let's get started.
Adapter Design Pattern
We are using the adapter design patter for adapting something to our project. Our used models or structures probably will not be same as the our used external plugin. So we need to adapt that plugin with our project. For this we use Adapter Design Patterns and adapter classes.
I will do it with an interface class. I will have a class for external serialport device. The provider of that device provided a class to use it on my project. I will make a connection via this adapter class from my application to that device.
Now let's look at an example about Adapter Design Pattern.
I built a class named SerialDeviceManager and I derived it from SerialDeviceInterface. This interface required for managing the external device. And I used this class from our main function of the program.
Program.cs
using System;
namespace AdapterPattern_Example
{
class Program
{
static void Main(string[] args)
{
SerialDeviceManager manager = new SerialDeviceManager();
manager.connectDevice();
Console.WriteLine("Make here some operations...");
manager.disconnectDevice();
Console.ReadLine();
}
}
}
SerialDeviceManager.cs
using System;
namespace AdapterPattern_Example
{
class SerialDeviceManager : SerialDeviceInterface
{
public void connectDevice()
{
Console.WriteLine("Device Connected...");
}
public void disconnectDevice()
{
Console.WriteLine("Device Disconnected...");
}
private void anotherMethod()
{
//This method not required at main program
}
}
}
SerialDeviceInterface.cs
namespace AdapterPattern_Example
{
interface SerialDeviceInterface
{
void connectDevice();
void disconnectDevice();
}
}
That is all in this article.
Have a great Adapting classes.
Burak Hamdi TUFAN
Comments