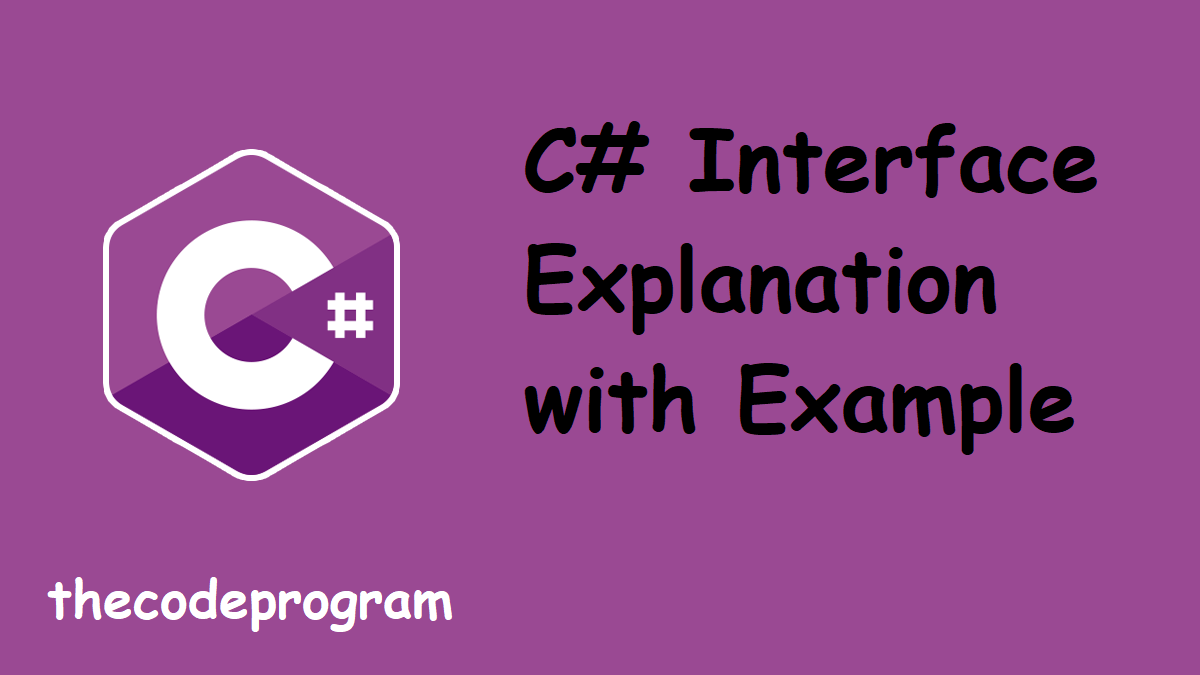
C# Interface Explanation with Example
Hello everyone, in this article we are going to talk about Interface. Purpose of interfaces, what is interface and why should we use interface. I will make an example in C# to explain interface and show the use of interface.Lets begin then.
What is Interface?
Interface is a class which has no function bodies. It has just function, property, indexer and event declerations. Mostly programming languages we can derive the class from only one class. We can implement more than one interfaces to a class. This is the reason of the usage of interfaces. You can not declare variables as public, private or something like them.
- We use interface keyword when we declared an interface.
- We can declare only methods, properties, indexes and events in the interfaces. We can not define the body of the method.
- We can not use modifiers inside this interface. All classes can reach the interface methods and properties. So we can not use public private or something like them. All of members of an interface is public.
- Most programming languages do not allow the multiple inheritance. So you can derive a class only one class. We can implement multiple interfaces into the classes. But remembe implementing is not inheritance.
- We can derive an interface from another interface.
- The classes that derived from interface must implement all methods at interface.
- If a class derived from multiple interfaces we will seperate the inerfaces with comma (,) .
- All of the members of an interface must declare as public at class which derived from related interface.
- We can create methods or properties at the class that do not exist in the interface. Also we can use any modifier that we want.
Now We have information what interface is. Let's make example about interface and get our information better.
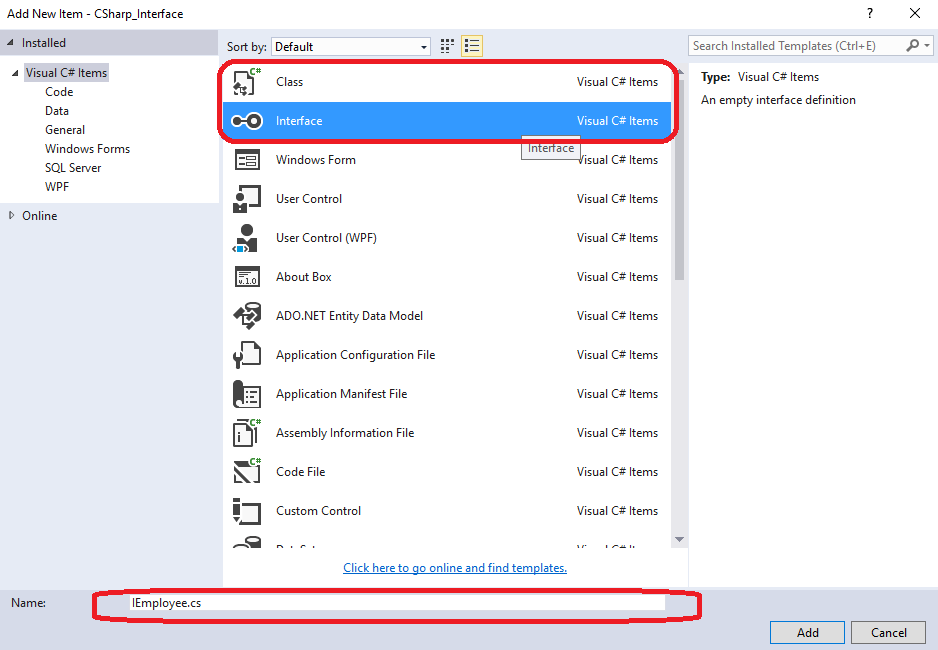
As you can see the above image I give the name starting with I. This is not required but strongly recommend to do this to decrease confusing between the names of classes.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharp_Interface
{
interface IEmployee
{
}
}
And I will declare some properties and methods which belong to the Employee.
NOTE: I will not the write the entire interface class. I will write here only interface content to prevent to write same things again and again.
interface IEmployee
{
//Properties of Employee
string NAME_SURNAME { get; set; }
string ROLE { get; set; }
string DEPARTMENT { get; set; }
int SALARY { get; set; }
DateTime STARTING_DATE { get; set; }
//Methods of Employee
void SET_NEWROLE(string _ROLE);
void SET_NEWDEPARTMENT(string _DEPARTMENT);
void SET_SALARY(int _SALARY);
}
class Employee : IEmployee
{
public string DEPARTMENT
{
get
{
throw new NotImplementedException();
}
set
{
throw new NotImplementedException();
}
}
public string NAME_SURNAME
{
get
{
throw new NotImplementedException();
}
set
{
throw new NotImplementedException();
}
}
public string ROLE
{
get
{
throw new NotImplementedException();
}
set
{
throw new NotImplementedException();
}
}
public int SALARY
{
get
{
throw new NotImplementedException();
}
set
{
throw new NotImplementedException();
}
}
public DateTime STARTING_DATE
{
get
{
throw new NotImplementedException();
}
set
{
throw new NotImplementedException();
}
}
public void SET_NEWDEPARTMENT(string _DEPARTMENT)
{
throw new NotImplementedException();
}
public void SET_NEWROLE(string _ROLE)
{
throw new NotImplementedException();
}
public void SET_SALARY(int _SALARY)
{
throw new NotImplementedException();
}
}
I have created another class that derived from same Interfaces and implement all objects in that interface. Now below code block I will assign interface variables and I will use them seperatelly. Below code block you will see how can we use these interface and class:
IEmployee emp1 = new Employee();
IEmployee emp2 = new Employee2();
emp1.DEPARTMENT = "IT";
emp1.NAME_SURNAME = "Burak ";
emp1.ROLE = "Manager";
emp1.SALARY = 10000;
emp1.SET_NEWDEPARTMENT("Technic");
emp2.DEPARTMENT = "Sales";
emp2.NAME_SURNAME = "hamdi ";
emp2.ROLE = "Intern";
emp2.SALARY = 10000;
emp2.SET_NEWDEPARTMENT("Technic");
IEmployee emp = new Employee();
emp = new Employee2();
That is all in this article.
Have a nice interfacing.
Burak Hamdi TUFAN
Comments