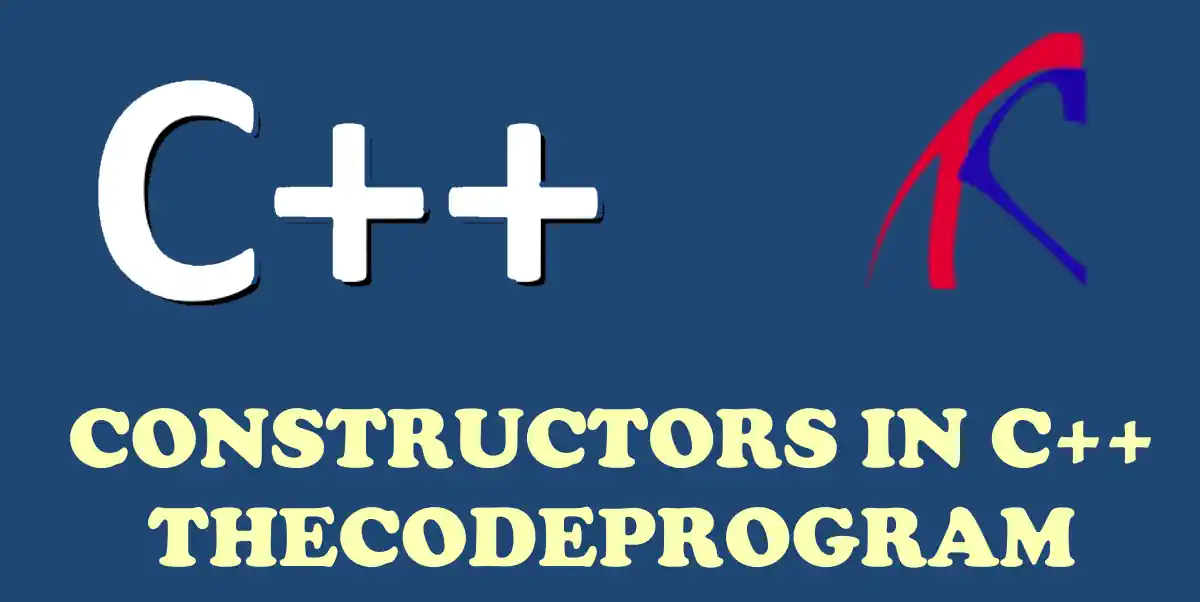
Constructors in C++
Hello everyone, in this article we are going to talk about constructors and destructors which are special members of a class in C++ with help of examples.Lets get started.
Constructor is a special member of a class that is calling automatically when class is initialised. Constructors have no return type and they are very important for object oriented programming. Constructors are so helpful for initialising an object properly. We can use the constructor to configure the all datas of an object when it is started.
Constructors do not have return types. SO they do not return any values. They have been directly called after object is created.
class class_name {
public:
class_name() {
// Object initialisation
}
};
Now lets start to take a look at the types of constructors one by one.
Default Constructor
Default constructor is a type of a constructor which does not take any parameter. Default constructors are also automatically creating by the C++ compiler if you don't define. They directly getting called when an object initialized.
#include <iostream>
using namespace std;
class Vehicle {
public:
Vehicle () {
//Initialize the interval variables when object created
value = 5;
cout << "Vehicle object is initialized." << endl;
cout << "Internal value is: " << value << endl;
}
private:
int value;
};
int main() {
Vehicle vec;
return 0;
}
Output of above code block:
Vehicle object is initialized.
Internal value is: 5
As you can see after the object is created, default constructor will be invoked directly.
Parameterized Constructor
Parameterized constructor is a type of a constructor which takes parameters. With parameterized constructor, we can send the initial values to class and object uses these initial values for initialization.
#include <iostream>
using namespace std;
class Vehicle {
private:
std::string brand;
int year;
public:
// parameterized constructor
Vehicle (std::string _brand, int _year) {
brand = _brand;
year = _year;
}
void printDetails() {
cout << "Brand is: " << brand << " and model year is " << year << endl;
}
};
int main() {
// Initialize the object with initial values
Vehicle veh("Renault", 1999);
veh.printDetails();
return 0;
}
Output
Brand is: Renault and model year is 1999
When we have initialized this object it will set up the internal variables and when we call the function to print, it will directly print the values.
Copy Constructor
Copy constructor is a type of constructor which allow us to create an object as a copy of an existing one. It takes itself as a parameter and initialize the object with the values in parameter.
#include <iostream>
using namespace std;
class Vehicle {
private:
std::string brand;
int year;
public:
// initialize variables throguhconstructor
Vehicle (std::string _brand, int _year) {
brand = _brand;
year = _year;
}
// copy values from existing object with address ob object
Vehicle (Vehicle &obj) {
brand = obj.brand;
year = obj.year;
}
void printDetails() {
cout << "Brand is: " << brand << " and model year is " << year << endl;
}
};
int main() {
// Initialize the object with initial values
Vehicle veh("Renault", 1999);
veh.printDetails();
return 0;
}
Output
Brand is: Renault and model year is 1999
As you can see above we have initialized new object from existing object.
That is all for now.
Burak Hamdi TUFAN
Comments