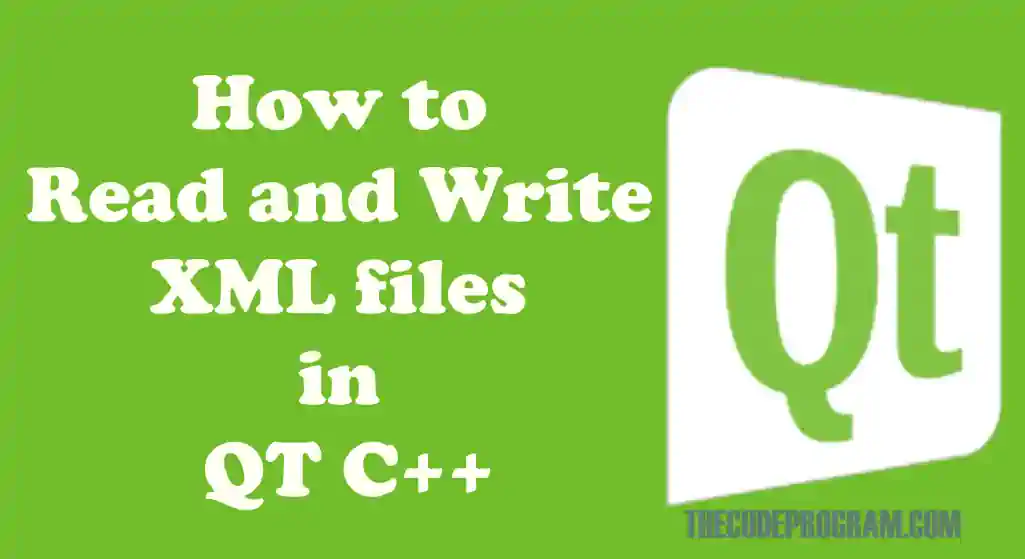
How to Read and Write XML files in QT C++
Hello everyone, in this article we are going to read and write XML files with using QT C++. We will make an example about management of XML datas in QT C++.Let's begin.
We can write and read XML files to store some datas in an XML file to use later. In qt we can perform this operations with QtXML library.
Firstly we need to add below row into .pro file of the project.
QT += xml
Now we need to include below two libraries in our header.
#include <QtXml>
#include <QTextStream>
Now we let's create XML elements and load datas in it. Then write them into file.
Below code block will create an XML file and write XML data in created file.
void XmlExample::writeXMLFile(){
QFile xmlFile("xmlSample.xml");
if (!xmlFile.open(QFile::WriteOnly | QFile::Text ))
{
qDebug() << "Already opened or there is another issue";
xmlFile.close();
}
QTextStream xmlContent(&xmlFile);
QDomDocument document;
//make the root element
QDomElement root = document.createElement("StudentList");
//add it to document
document.appendChild(root);
QDomElement student = document.createElement("student");
student.setAttribute("id", "1");
student.setAttribute("name", "Burak");
student.setAttribute("number", "1111");
root.appendChild(student);
student = document.createElement("student");
student.setAttribute("id", "2");
student.setAttribute("name", "Hamdi");
student.setAttribute("number", "2222");
root.appendChild(student);
student = document.createElement("student");
student.setAttribute("id", "3");
student.setAttribute("name", "TUFAN");
student.setAttribute("number", "33333");
root.appendChild(student);
student = document.createElement("student");
student.setAttribute("id", "4");
student.setAttribute("name", "Thecodeprogram");
student.setAttribute("number", "4444");
root.appendChild(student);
xmlContent << document.toString();
}
What have we done in writing method :
Now let's read the XML file which we have written above.
Below code you will see the reading XML file:
void XmlExample::loadXMLFile(){
QDomDocument studentsXML;
QFile xmlFile("xmlSample.xml");
if (!xmlFile.open(QIODevice::ReadOnly ))
{
// Error while loading file
}
studentsXML.setContent(&xmlFile);
xmlFile.close();
QDomElement root = studentsXML.documentElement();
QDomElement node = root.firstChild().toElement();
QString datas = "";
while(node.isNull() == false)
{
qDebug() << node.tagName();
if(node.tagName() == "student"){
while(!node.isNull()){
QString id = node.attribute("id","0");
QString name = node.attribute("name","name");
QString number = node.attribute("number","number");
datas.append(id).append(" - ").append(name).append(" - ").append(number).append("\n");
node = node.nextSibling().toElement();
}
}
node = node.nextSibling().toElement();
}
ui->txtLoadedXmlContent->setPlainText(datas);
}
What have we done in reading method :
That is all in this article.
Have a good XML management.
Burak Hamdi TUFAN
Tags
Share this Post
31/10/2020
Explanation of LinkedList in Java
23/03/2021
Comments