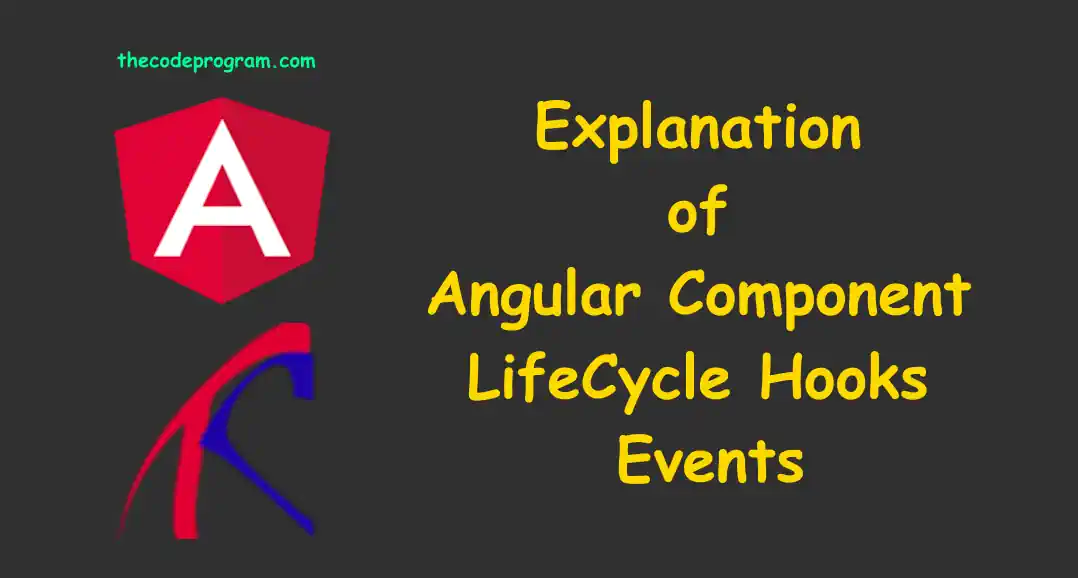
Explanation of Angular Component LifeCycle Hooks and Events
Hello everyone, in this article we are going to talk about Life Cycle of an Angular component which is one of most essential topic in Angular development.Let's get started.
Angular is a very famous framework for building dynamic web applications. Components are one of base built pieces of an angular application. With components we can create reusable components to improve the maintainability of Angular web applications.
Introduction of Angular Component Lifecycle
Angular components has their of life cycle from constructing to destructing. These lifecycle stages have events and hooks which are invoking at these stages of components. A developer can execute some code on different stages of component. We can improve the efficiency of the application with using these life cycle events.
Now let's dive in the implementations of Life Cycle hooks and events
Angular Component and the Constructor
NOTE
Firstly we need to understand what is an Angular component in Angular.
A component is a TypeScipt file in Angular which defined with @Component decorator. Class is having the required metadats like template and class location, CHangeDetection strategy and selector specifier to manage the appearence and behaviour with the Component Decorator.
import { Component } from '@angular/core';
@Component({
selector: 'app-example',
template: '<p>Hello, Thecodeprogram</p>',
})
export class ExampleComponent {
constructor() {
console.log('Constructor of Angular component is called');
}
}
Above you can see an Angular component definition and its constructor method for performing initial operations.
OnChanges and OnCheck Hooks
OnChanges and DoCheck hook is being called depending on the defined change detection strategy in Component Decorator. In default change detaction strategy it is invoking contuniously. If we use OnPush strategy, it will be called when an input property is changed or a detect changes operation is invoked.
Difference between OnChanges and DoCheck we are able to check the input properties with ngOnChanges to perform some additional logics on data changing.
We have to be carefull when using OnCHanges and DoCheck. We should not implement complex and unnecessary logic with these hooks. Because with default strategy they are being called continuously and with complex operations there will be possible performance issues in your web application.
Below you can see an Angular component with ngOnChanges and ngDoCheck
import { Component, OnChanges, DoCheck } from '@angular/core';
@Component({
selector: 'lifecycle-example',
template: '<p>Hello, Thecodeprogram</p>',
})
export class ExampleComponent implements OnChanges, DoCheck {
ngOnChanges(changes: SimpleChanges) {
// Perform operations with ngOnChanges
}
ngDoCheck() {
// Implement custom logic to perform every default change detection cycle
}
}
OnInit Hook
OnInit hook is calling for one time on the initiialisation stage of a component. It is a good event to prepare the component for its functionality like calling API's and some intial checks.
Below you can see an Angular component with ngOnInit
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'lifecycle-example',
template: '<p>Hello, Thecodeprogram</p>',
})
export class ExampleComponent implements OnInit {
ngOnInit() {
// Perform initialisation logic with ngOnInit
}
}
AfterContentInit and AfterContentChecked Hooks
AfterContentInit and AfterContentChecked Hooks are invoking after directives are loaded and their first change detection cycle is happened in the component. These hooks are helping us to manage the elements which are loaded by directives in the main component.
Below you can see an Angular component with ngAfterContentInit and ngAfterContentChecked
import { Component, AfterContentChecked, AfterContentInit } from '@angular/core';
@Component({
selector: 'lifecycle-example',
template: '<p>Hello, Thecodeprogram</p>',
})
export class ExampleComponent implements AfterContentInit, AfterContentChecked {
ngAfterContentInit(): void {
// Perform related operations after directives loaded
}
ngAfterContentChecked(): void {
// Perform related operations after directives first change detection cycles are done
}
}
AfterViewInit and AfterViewChecked Hooks
AfterViewInit and AfterViewChecked Hooks are calling after the view of the component is loaded and first change detaction cycle is completed.
Below you can see an Angular component with ngAfterViewInit and ngAfterViewChecked
import { Component, AfterViewChecked, AfterViewInit } from '@angular/core';
@Component({
selector: 'lifecycle-example',
template: '<p>Hello, Thecodeprogram</p>',
})
export class ExampleComponent implements AfterViewInit, AfterViewChecked {
ngAfterViewInit(): void {
// Invoking after the view initialisation is completed
}
ngAfterViewChecked(): void {
// Perform the operations after first change detection cycle is done of the view
}
}
OnDestroy Hook
OnDestroy is performing when component is removing Mostly all cleanups and unsubscribings are happening in OnDestroy event in the component.
Below you can see an Angular component with ngOnDestroy
import { Component, OnDestroy } from '@angular/core';
@Component({
selector: 'lifecycle-example',
template: '<p>Hello, Thecodeprogram</p>',
})
export class ExampleComponent implements OnDestroy {
ngOnDestroy() {
// Perform logics on destroying the component, mostly cleanups happening here
}
}
Conclusion
Understanding and implementing the Angular lifecycle hooks is very important to increase the efficiency of the application. We can manage our web application in correct stages like calling API's in initialisations, unsubscribing from async calls at destructor, or manage the component depending the the input datas when changed on running and other most of things. We can increase the efficiency with the correct using of lifecycle hooks.
That is all
Burak Hamdi TUFAN
Comments