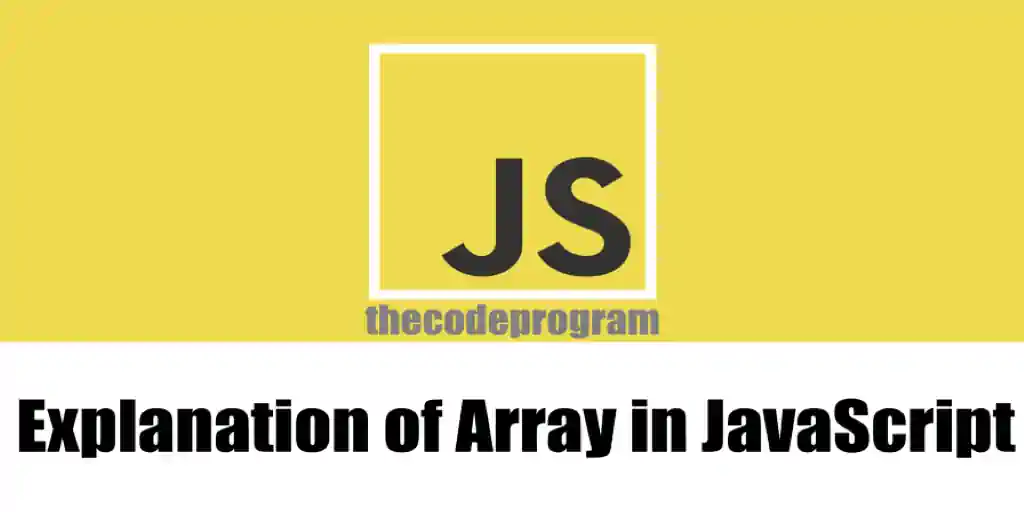
Explanation of Array in JavaScript
Hello everyone, in this article we are going to talk about arrays in JavaScript. We will see the methods of arrays and then we will make some examples to understand what they are.Let's get started.
Firstly, What is an array ?
Array is a variable type which store the multiple values. Normally, we define variables for each value to store. But we can use only one array to store all of these variables.
Firstly let's create and initialize an array with its values in JavaScript
var me = ["Burak", "Hamdi", "TUFAN"];
var web = ["The", "Code", "Program", 1, 2, 3];
var num = [1, 2, 3, 4, 5];
var inf = new Array("Array", "with", "new", "keyword");
Now let's access and change the element which inside of array.
var aircrafts = ["Airbus", "Boeing", "Embraer"];
console.log(aircrafts);
aircrafts[0] = "Airbus Belluga XL";
aircrafts[1] = "Boeing 777-300ER";
aircrafts[2] = "Embraer 190";
console.log("Updated aircrafts : " + aircrafts);
There are some special properties and methods for arrays in Javascript. Let's look at them now:
var array = ["The", "Code", "Program"];
document.getElementById("divOutput").innerHTML += "length : " + array.length + "<br />";
document.getElementById("divOutput").innerHTML += "indexOf: " + array.indexOf("Program") + "<br />";
var sortedArray = array.sort();
document.getElementById("divOutput").innerHTML += "sort() : " + sortedArray + "<br />";
array.push("pushedElement");
document.getElementById("divOutput").innerHTML += "push() : " + array+ "<br />";
array[array.length] = "pushedWithIndex";
document.getElementById("divOutput").innerHTML += "push() : " + array+ "<br />";
document.getElementById("divOutput").innerHTML += "forEach() : <br />";
array.forEach( foreachFunc );
function foreachFunc(value) {
document.getElementById("divOutput").innerHTML += "--" + value + "<br />";
}
document.getElementById("divOutput").innerHTML += "Array.isArray() :" + Array.isArray(array); + " <br />";
So what if we want to use names as index keys instead of numbers. In this case we use Java Script objects.
var me = { "name":"Burak Hamdi" ,
"surname" : "TUFAN" ,
"web" : "Thecodeprogram" ,
"language" : "JavaScript" };
document.getElementById("divObjectOutput").innerHTML = JSON.stringify(me) + "<br />";
document.getElementById("divObjectOutput").innerHTML += "Name : " + me["name"] + "<br />";
document.getElementById("divObjectOutput").innerHTML += "Surname : " + me["surname"] + "<br />";
document.getElementById("divObjectOutput").innerHTML += "Web : " + me["web"] + "<br />";
document.getElementById("divObjectOutput").innerHTML += "Language : " + me["language"] + "<br />";
JSON.stringify() is a method which convert the json array object into string. So we can print the object as text.
That is all in this article.
I wish you healthy days.
Burak Hamdi TUFAN.
Comments