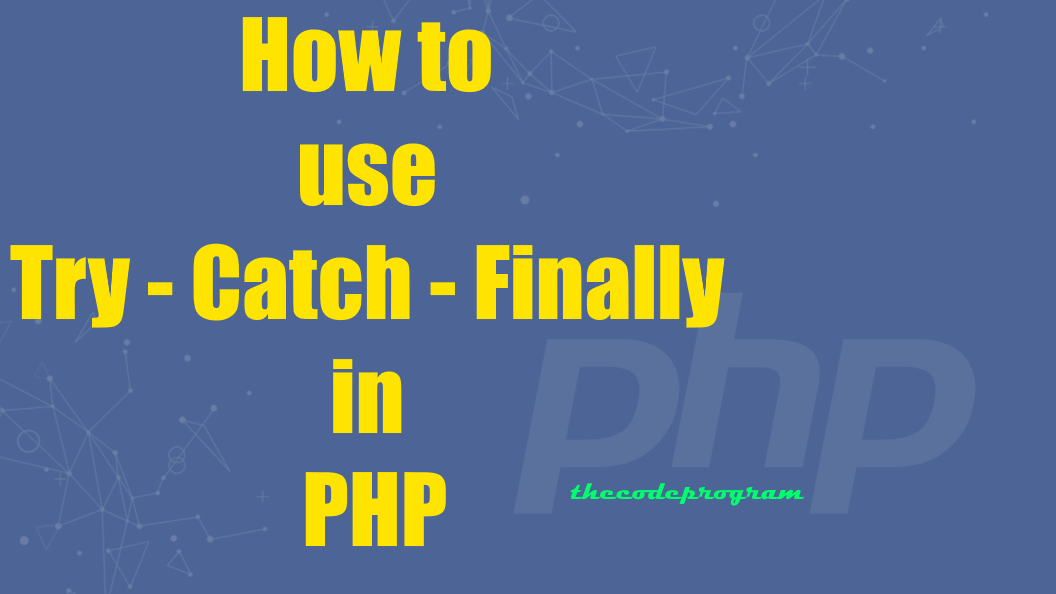
How to use Try - Catch - Finally in PHP
Hello everyone, in this article we are going to talk about Exceptions in PHP. We will talk about Try - Catch and Finally. We will explain it first and then make an example on it with PHP.Let's begin.
In PHP there is an Exception model like any other programming kanguages. You can Catch the specified exception and throw it to show developer and the user if you want.
To accomplish this you must write code inside a try{ .... } block. After this try block, you have to locate a catch block or a finally block to make somethings if an exception occured.
When an exception occured in the try block, the code does not continue and it goes to catch block. If there is no exception, catch block does not run. You can do whatever you want in the catch block: you can save the problem to the database or show to user to warn for something.
If the catch class has a variable it must be Exception class or a class which derived from Exception class. I mean you can use differant type of Exception classes in your try catch blocks. All you have to be carefull, these classes must be Exception class or Exception-Derived classes.
You can also write more than one catch blocks after the try block for different type Exceptions. With this specification you can catch multitype class Exceptions. After PHP 7.1 you can make it with ( | ) operator at same catch block.
Also you can use finally block after try. Finally block runs even if there is no exception after the try block. Catch block runs if there is an exception, and finally block runs at both situtation theres an exception or no exception. This is the differance than catch block.
Now let's write some to get this understand.
try {
// The code block you will run and check for exception
}
catch (Exception $ex) {
// This area will run if an exception occured
echo $ex->getMessage();
}
finally {
//This code block will run at last. If there is an exception occured or not.
}
Below code block you will see the example and output.
try {
echo "Try code block.";
throw new Exception();
} catch (Exception $e) {
echo "This is the catch block.";
} finally {
echo "This is the finally block.";
}
Output:
Try code block.
This is the catch block.
This is the finally block
Below code block you will see the how can we use miltiple Exception derived class with multiple catch blocks.
//These classes derived from exception classes
class ExceptionClass_1 extends Exception {};
class ExceptionClass_2 extends Exception {};
function build_exception($errorType)
{
try
{
if ($errorType == '1') {
throw new ExceptionClass_1();
}
else if ($errorType == '2') {
throw new ExceptionClass_2();
}
else{
throw new Exception();
}
}
catch (ExceptionClass_1 $ex)
{
echo "Exception Class 1\n";
}
catch (ExceptionClass_2 $ex)
{
echo "Exception Class 2\n";
}
catch (Exception $e)
{
echo "General Type Exception\n";
}
}
At above code block, if we call the
build_exception('1');
build_exception('2');
build_exception('another');
Exception Class 1
Exception Class 2
General Type Exception
Now we are ready to handle Exceptions in PHP.
That is all in this article.
Have good exception handling.
Burak Hamdi TUFAN.
Comments