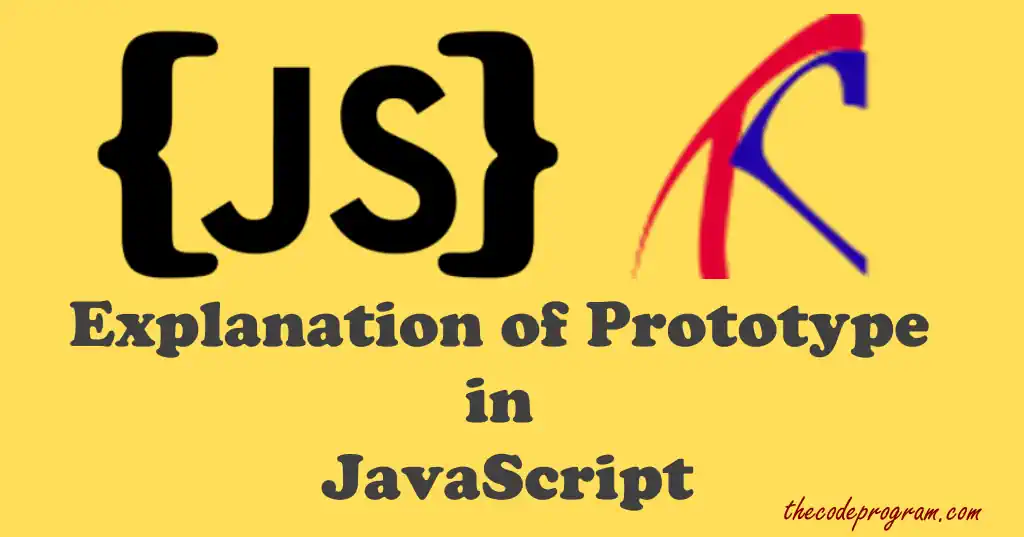
Explanation of Prototype in JavaScript
Hello everyone, in this article we are going to talk about Javascript prototypes. In this article we are also talk about Javascript objects and constructors with examples.Let's get started.
What is prototype
All objects in Javascript have their own prototypes. When an object initialized, instance took all properties and methods from object prototype. We can access the prototype via ObjectName.prototype.
function User() {
this.name = "Burak Hamdi" ;
this.surname = "Tufan";
}
var u = new User();
console.log(u.name);
console.log(u.surname);
User.prototype.web = "thecodeprogram.com";
console.log(u.web);
User.prototype.showFullname = function() {
return this.name + " " + this.surname;
}
console.log(u.showFullname());
Example output will be like below:
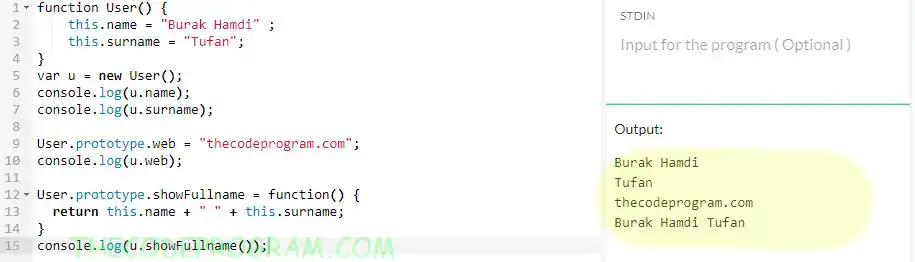
All built-in classes in Javascript took their own properties and methods from their own prototypes.
We can also create objects with initial values. We are going to use Constructor to initialize an object with initial values.
function User(_name, _surname, _web) {
this.name = _name;
this.surname = _surname;
this.web = _web;
}
var u = new User("Burak Hamdi", "TUFAN", "thecodeprogram.com");
console.log(u.name);
console.log(u.surname);
console.log(u.web);
User.prototype.showFullname = function() {
return this.name + " " + this.surname;
}
console.log(u.showFullname());
User.prototype.showFullData = function() {
return "\nFull Data of object : \n" + this.name + " \n" + this.surname + " \n" + this.web;
}
console.log(u.showFullData());
Below image you can see the example output:
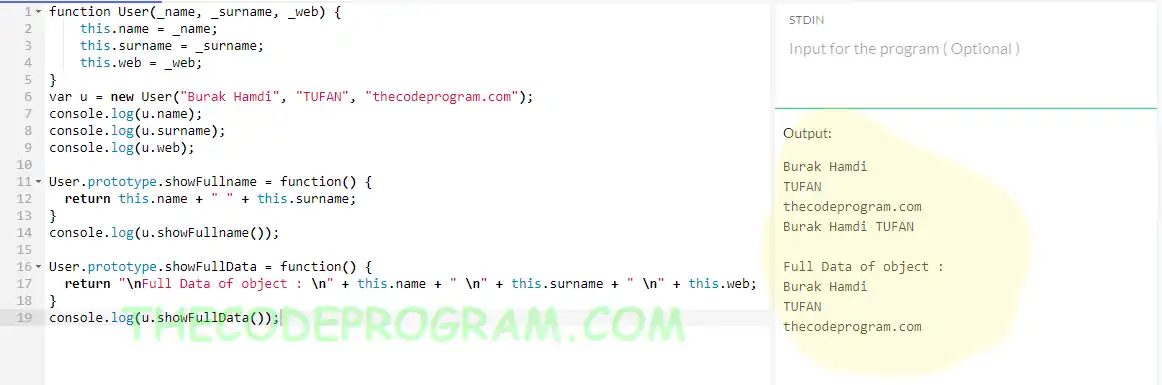
There is an important detail that you need to remember that adding the members to instance of classes will not change the original class and other initialized instances. Only adding members to classes via prototypes will affect all created instances.
function User(_name, _surname) {
this.name = _name;
this.surname = _surname;
}
var u1 = new User("Burak Hamdi", "TUFAN");
var u2 = new User("John", "DOE");
u1.web = "thecodeprogram.com";
u2.language = "Javascript";
//first whow data that exist in u1 and not exist in u2 and viseverse
console.log(u1.web)
console.log(u2.web)
console.log(u1.language)
console.log(u2.language)
User.prototype.showFullname = function() {
return this.name + " " + this.surname;
}
//If you call showFullname method before prototype, it will throw error on function not exist
console.log(u1.showFullname());
console.log(u2.showFullname());
Below image you can see the example output.
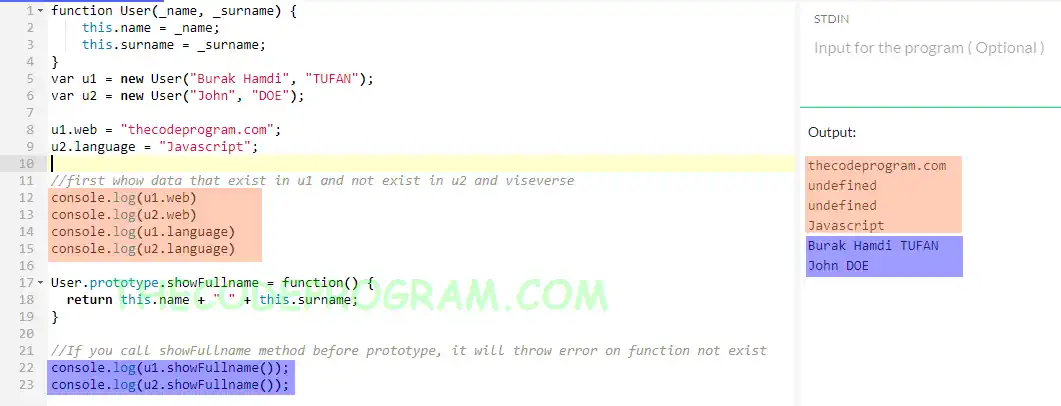
That is all in this article.
Have a good prototyping.
Burak Hamdi TUFAN
Comments