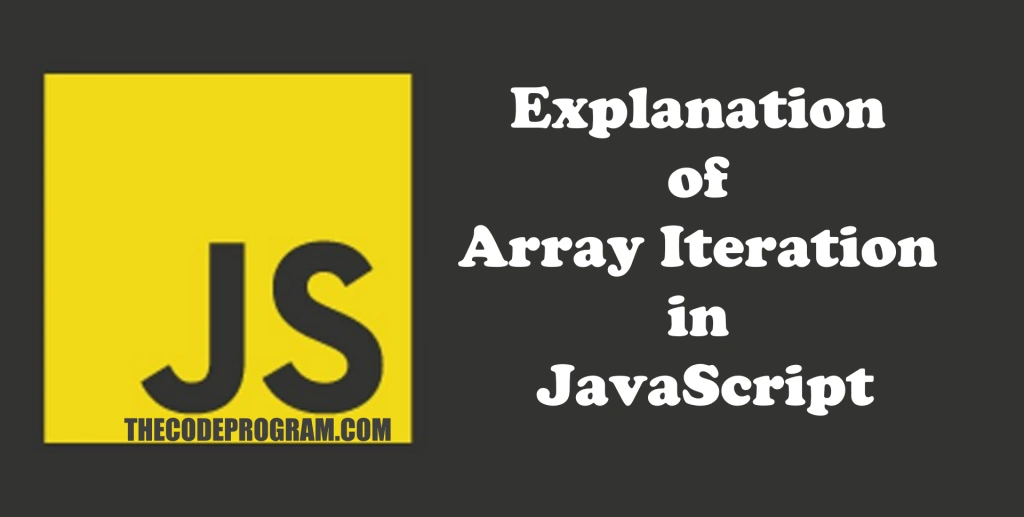
Explanation of Array Iteration in JavaScript
Hello everyone, in this article we are going to talk about how can we iterate arrays in JavaScript. We are going to talk about different types of arrays in JavaScript.Let's begin
Now let's start to make example about them one-by-one.
forEach()
We use forEach function to execute a task for every element in given array.
function printValues(value) {
console.log(value);
}
var numbers = [1, 2, 3, 4, 5, 10];
numbers.forEach(printValues);
Output will be:
1
2
3
4
5
10
map()
We can create a new array from given array. Map function does not change the original array and it allows us to process the values of given array with in use callback function.
If there is null value in given array, these values will be 0 in new created array.
function calculateVal(value) {
return value++;
}
var numbers = [1, 2, 3, null, 4, 5, 10, null];
const newNumbers= numbers.map(calculateVal);
console.log(newNumbers.toString());
Output will be
1,2,3,0,4,5,10,0
filter()
filter function creates another array with using a boolean type function. It checks the elements with this function. If it returns true, it means the element passed the condition and it creates new array with passed elements.
function isPositive(value) {
return value >= 0;
}
var numbers = [1, -2, 3, -4, -5, 6, -7, 8];
var positives= numbers.filter(isPositive);
console.log(positives.toString());
Output will be
1,3,6,8
reduce()
We use reduce function to create one element from all element insde given array. We can also use reduceRight function to calculate elements from right-to-left.
function sum(total, value) {
return total + value;
}
var numbers = [7, 77, 55, 3, 4, 61, 33];
var average= numbers.reduce(sum) / numbers.length;
console.log(average);
Output will be:
34.285714285714285
We can also give an initial value to start calculation from one number.
function sum(total, value) {
return total + value;
}
var numbers1 = [7, 77, 55, 3, 4, 61, 33];
var numbers2 = [1, 17, 37, 9, 6, 39, 99];
var sumOfArrays = numbers2.reduce(sum, numbers1.reduce(sum));
console.log(sumOfArrays);
Output will be:
448
every()
We can check every element in the array for obeying the specified condition. We use a callback functions to check every element in array. If all of elements return true, function will return true.
function isPositive(value) {
return value >= 0;
}
const numbers1 = [1, -2, 3, -4, -5, 6, -7, 8];
var isAllPositive1= numbers1.every(isPositive);
const numbers2 = [1, 2, 3, 4, 5, 6, 7, 8];
var isAllPositive2= numbers2.every(isPositive);
console.log(isAllPositive1);
console.log(isAllPositive2);
Output will be :
false
true
some()
We can check every element in the array for obeying the specified condition. We use a callback functions to check every element in array. If at least one element returns true, the function will return true.
function isNegative(value) {
return value < 0;
}
const numbers1 = [1, -2, 3, 4, 5, 6, -7, 8];
var hasNegative1 = numbers1.some(isNegative);
const numbers2 = [1, 2, 3, 4, 5, 6, 7, 8];
var hasNegative2 = numbers2.every(isNegative);
console.log(hasNegative1);
console.log(hasNegative2);
Output will be :
true
false
IndexOf()
We can find the first index of given value in the given array. If not exist in the array it returns -1.
var strings = ["Burak", "Hamdi", "TUFAN", "The", "Code", "Program"];
console.log(strings.indexOf("TUFAN"));
console.log(strings.indexOf("TheCode"));
Output will be
2
-1
lastIndexOf()
We can find the last index of given value in the given array. If not exist in the array it returns -1.
var strings = ["Burak", "Hamdi", "TUFAN", "The", "Code", "Program", "TUFAN"];
console.log("First index : " + strings.indexOf("TUFAN"));
console.log("Last Index : " + strings.lastIndexOf("TUFAN"));
console.log(strings.indexOf("TheCode"));
Output will be
First index : 2
Last Index : 6
-1
includes()
We can check th given element value is exist in the given array. It returns boolean result.
var strings = ["Burak", "Hamdi", "TUFAN", "The", "Code", "Program"];
console.log(strings.includes("TUFAN"));
console.log(strings.includes("TheCode"));
Output will be
true
false
find()
Uses a boolean type function to return first element value which passes the given condition
function check(value) {
return value > 33;
}
var numbers = [7, 77, 55, 3, 4, 61, 33];
var firstFound = numbers.find(check);
console.log(firstFound);
Output will be
77
findIndex()
Uses a boolean type function to return first element index which passes the given condition
function check(value) {
return value > 33;
}
var numbers = [7, 77, 55, 3, 4, 61, 33];
var firstIndex = numbers.findIndex(check);
console.log(firstIndex);
Output will be
1
from
From function creates an array from a given array-like object or an iterable object like strings
var newArray = Array.from("THECODEPROGRAM");
console.log(newArray.toString());
Output will be:
T,H,E,C,O,D,E,P,R,O,G,R,A,M
That is all in this article.
Burak Hamdi TUFAN
Comments