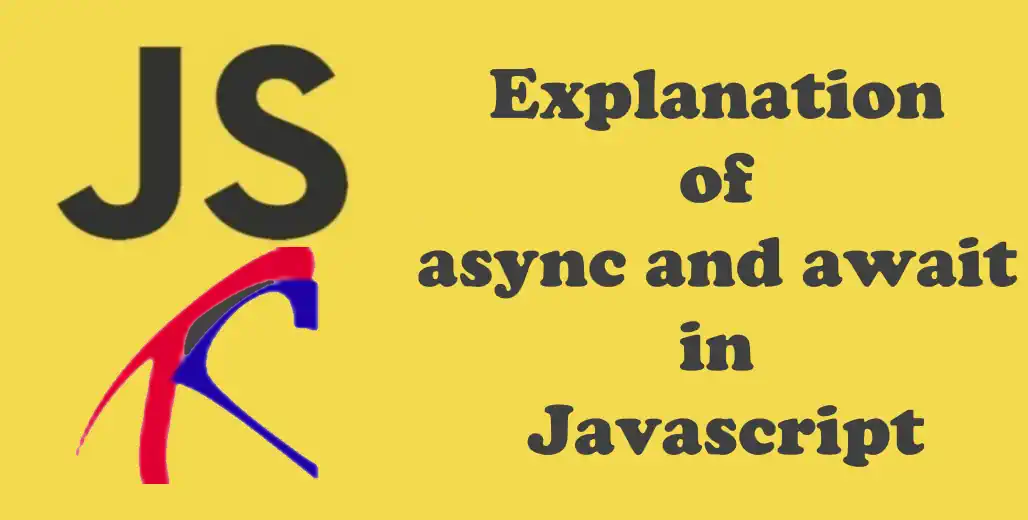
Explanation of async and await in Javascript
Hello everyone, in this article we are going to talk about async and await keywords within javascript. We will understand async and await with simple but effective examples.Let's begin.
ASYNC
Firstly what is async
async is a keyword which specify the function is an asynchronous function. Async functions return promise in javascript.
async function theFunction() {
//statements
}
An async function always returns a promise. We can make the function return a rejected or resolved promise. If we do not return a Promise, that function will return implicitly resolved promise.
//Below two functions are returning same result.
async function theFunction() {
return "Thecodeprogram";
}
function theFunction() {
return Promise.resolve("Thecodeprogram");
}
As we know promise methods allow chaining with then function. You can reach more details on Explanation of Promise in JavaScript article. Below code block you can see the example.
async function theFunction () {
console.log("TheCodeProgram ASYNC function.");
}
theFunction().then(function(res) {
console.log(res)
});
Output will be below

AWAIT
Firstly what is await
awaitis a keyword allow us to wait a promise function execution is completed. When we need to wait a value from a promise function await is usefull for waiting.
function control() {
return new Promise(function(resolve,reject) {
setTimeout(function() {
resolve("promise resolved ")
},2000);
});
}
// async function
async function asyncFunction() {
console.log("Before initialization." );
let res = await control().then(function(res) {
console.log("promise handled Result is : " + res);
});
console.log("promise completed. " );
}
//Call the async function
asyncFunction();
Below image you can see the output.

In above code block execution will wait promise untill being finished. Normally promise function will be executed asynchronously. When we use await it is executing synchronize.
In our programs we may use multiple promises for same task and they may need their values. Syncronize execution is useful for these types of executions.
That is all in this article.
Burak Hamdi TUFAN
Comments