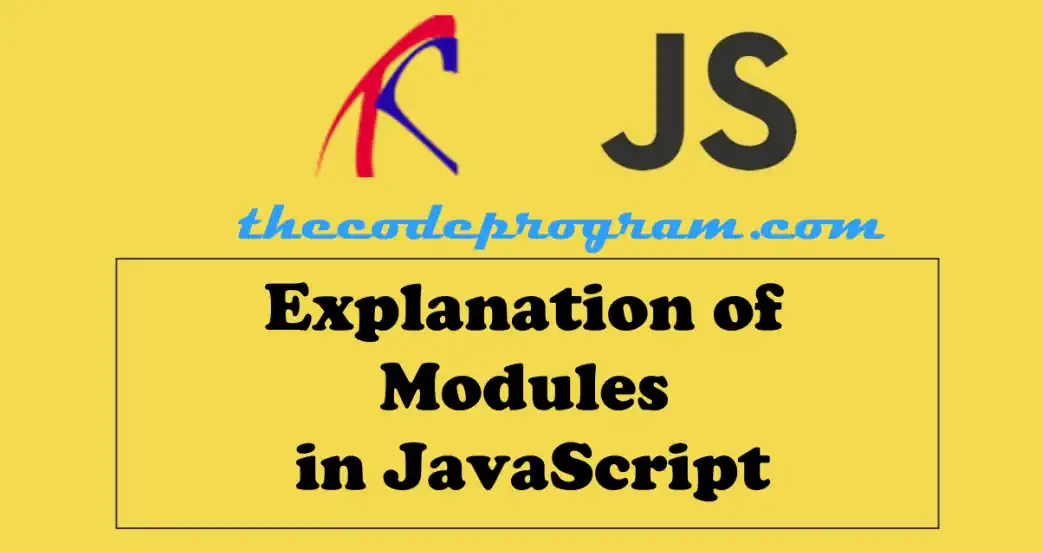
Explanation of Modules in JavaScript
Hello everyone, in this article we are going to talk about modules ın JavaScript. Modules wıll help us when we are working on big projects to tidy up the entire project.Let's get started.
Modules are the fıles to keep the codes for a specıfıc task. With the help of modules we can seperate the dedicated code blocks within specific javascript files. We can organize our java script structure easily with the help of the modules.
We can keep every required member (functions, variables...) for related task.
The logic is, we can export the specific members from related module file and these members can be imported within the other javascript files. With this way we can also create our own libraries.
export function multiply(num1, num2){
return num1 * num2;
}
export function myPrint(text){
console.log(text);
}
import { myPrint, multiply } from './utils.js';
var userObj = { "fullname": "Burak Hamdi TUFAN", "salary": "5000", "totalMonth": "117" };
function showUserData(){
var totalPaid = multiply(userObj.salary, userObj.totalMonth);
var data = userObj.fullname + "\nMonthly Salary: " + userObj.salary + "\nTotal Paid: " + userObj.totalMonth
myPrint(data );
}
showUserData();
Program output will be like below
Burak Hamdi TUFAN
Monthly Salary: 5000
Total Paid: 585000
There is another way to export the members from a module file. We can export them within a list also we can assign a different name for the functions to prevent the confusion with the other libraries. Let's make an example to understand this type of module decleration
function multiply(num1, num2){
return num1 * num2;
}
function print(text){
console.log(text);
}
//Method 1: Named Export
export { print as myPrint, multiply as myMultiply }
//Method 2: ListedExport
export { print, multiply }
import { myPrint, myMultiply } from './utils.js';
var userObj = { "fullname": "Burak Hamdi TUFAN", "salary": "5000", "totalMonth": "117" };
function showUserData(){
var totalPaid = myMultiply(userObj.salary, userObj.totalMonth);
var data = userObj.fullname + "\nMonthly Salary: " + userObj.salary + "\nTotal Paid: " + userObj.totalMonth
myPrint(data );
}
showUserData();
Program output will remain same
That is all in this simple article.
Have a good modularizing.
Burak Hamdi TUFAN
Comments