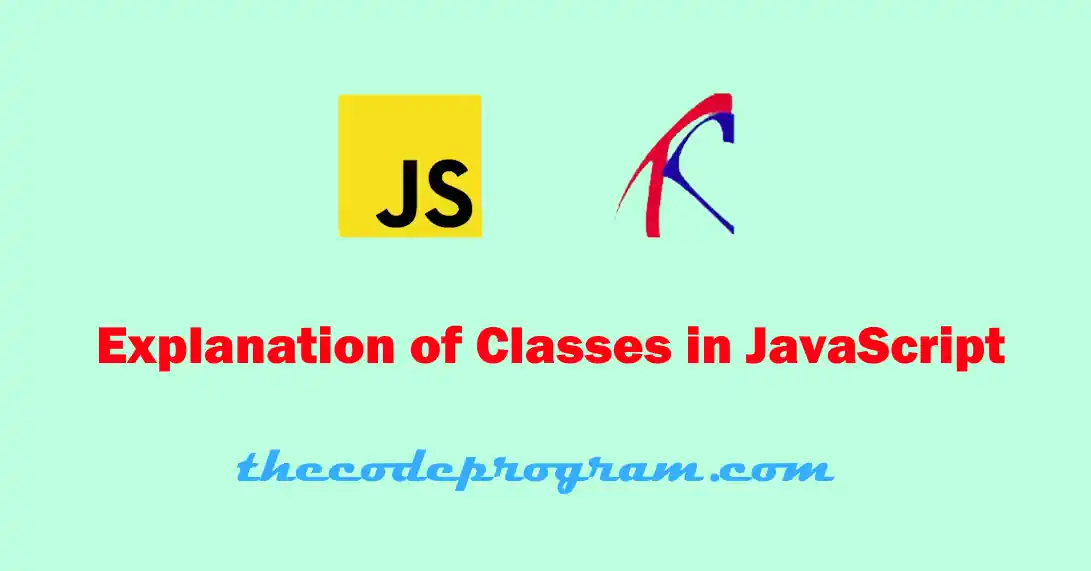
Explanation of Classes in JavaScript
Hello everyone, in this article we are going to talk about usages of classes which is one of very important feature in JavaScript with help of examples.Let's get started.
One of very important feature of Javascript is working with objects and classes and handle the data in an efficient way. Javascript provides an efficient way to create and use classes.
What is a Class?
A class is a template of objects which has same behaviours and properties. We can define the behaviours of objects and we can make them to use same properties.
Classes are introduced in JavaScript with ES6 and after ES6 JavaScript is started to be ObJect Oriented Programming language and more organised.
// Define a new class
class Vehicle {
constructor(brand, year) {
this.brand = brand;
this.year = year;
}
start() {
console.log(`Vehicle brand is ${this.brand} and year is ${this.year}.`);
}
}
//Initialise and use the class
const vehicle = new Vehicle('Tofas', 2000);
vehicle.start(); // Vehicle brand is Tofas and year is 2000.
As you can see above it is a classic class definition. In here brand and year are properties of class and start() is a method of a class. Also as you can see in constructor method we are initialising the values with parameters with we have sent.
// Define a new class
class Vehicle {
constructor(brand, year) {
this.brand = brand;
this.year = year;
}
start() {
console.log(`Vehicle brand is ${this.brand} and year is ${this.year}.`);
}
}
class Truck extends Vehicle {
constructor(brand, year, weight) {
super(brand, year); // Initialise the parent
this.weight = weight;
}
// Override the function of Parent
start() {
console.log(`Weight of the truck is ${this.weight}.`);
}
}
// Parent class
const vehicle = new Vehicle('Tofas', 2000);
vehicle.start(); // Vehicle brand is Tofas and year is 2000.
// Derived class
const truck = new Truck('BMC', 2020, 3000);
truck.start(); // Weight of the truck is 3000.
As you can see above we have two classes. We created the Vehicle class as a parent and we derived Truck class from this parent class. So now child class is allowed to use the properties of parent class. Also both classes have same method, In child class we defined exactly same method to override the parent method. When we call the method from child class, methods from child will be called.
class MathUtil {
static sum(a, b) {
return a + b;
}
static multiply(a, b) {
return a * b;
}
static subtract(a, b) {
return a - b;
}
static divide(a, b) {
return a / b;
}
}
console.log(MathUtil.sum(10, 20)); // 30
console.log(MathUtil.multiply(10, 20)); // 200
console.log(MathUtil.subtract(50, 30)); // 20
console.log(MathUtil.divide(40, 20)); // 2
As you can see above we have directly called the static methods without initialising the class since they are static functions.
Conclusion
Javascript provides very efficient way to organise the data with object-oriented way. With classes, we can implement reusable and more readable code. Also organising the data with object-oriented approach is easier with JavaScript.
That is all.
Burak Hamdi TUFAN
Comments