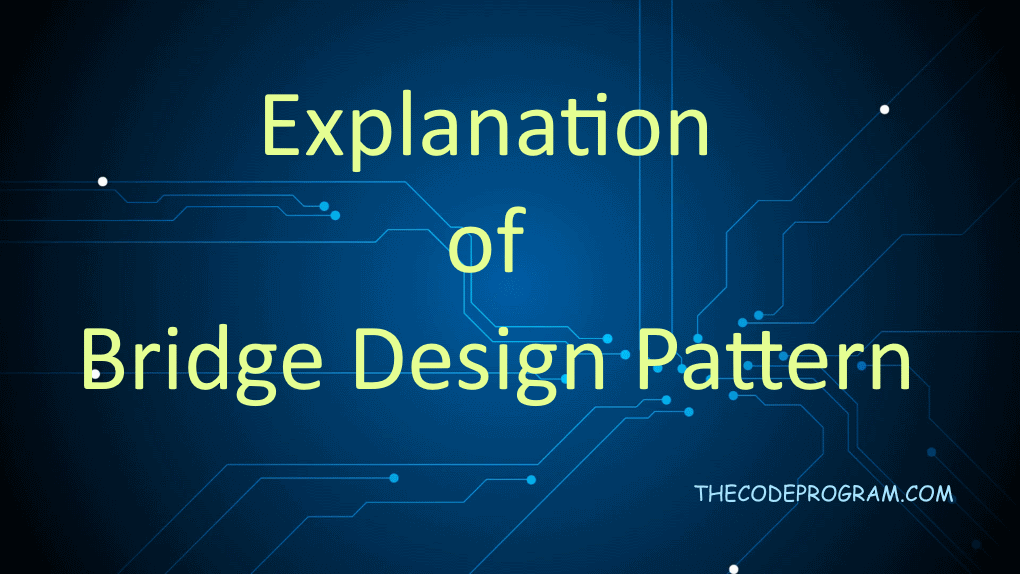
Explanation of Bridge Design Pattern
Hello everyone in this article we are going to talk about Bridge Design Pattern which is a part of Structural Design pattern. In this article I will make a simple explenation and I will give an example about Bridge Design Patterns.Now let's begin.
Bridge Design Pattern is the design patterns which seperates the abstract classes and interfaces. We will built bridge classes and we will seperate the vehicles and starting methods. I will start the car with button and truck with vehicle. I will manage it from one abstract class. This class will act as our Bridge class.
Below code blocks you can see the example:
Vehicle.cs
namespace BridgePattern_Example
{
public abstract class Vehicle
{
public IBridge _bridge;
public abstract void startVehicle();
}
}
Car.cs
using System;
namespace BridgePattern_Example
{
class Car : Vehicle
{
public override void startVehicle()
{
Console.WriteLine("Car started...");
}
}
}
Truck.cs
using System;
namespace BridgePattern_Example
{
class Truck : Vehicle
{
public override void startVehicle()
{
Console.WriteLine("Truck Started");
}
}
}
withButton.cs
using System;
namespace BridgePattern_Example
{
class withButton : IBridge
{
public void startBridge()
{
Console.WriteLine("started with Button");
}
}
}
withKey.cs
using System;
namespace BridgePattern_Example
{
class withKey : IBridge
{
public void startBridge()
{
Console.WriteLine("started with Key");
}
}
}
Program.cs
using System;
namespace BridgePattern_Example
{
class Program
{
static void Main(string[] args)
{
Vehicle vec = new Car();
vec._bridge = new withButton();
vec.startVehicle();
vec._bridge.startBridge();
vec = new Truck();
vec._bridge = new withKey();
vec.startVehicle();
vec._bridge.startBridge();
Console.ReadLine();
}
}
}
That is all in this article.
Have a good bridging.
Burak Hamdi TUFAN
Comments