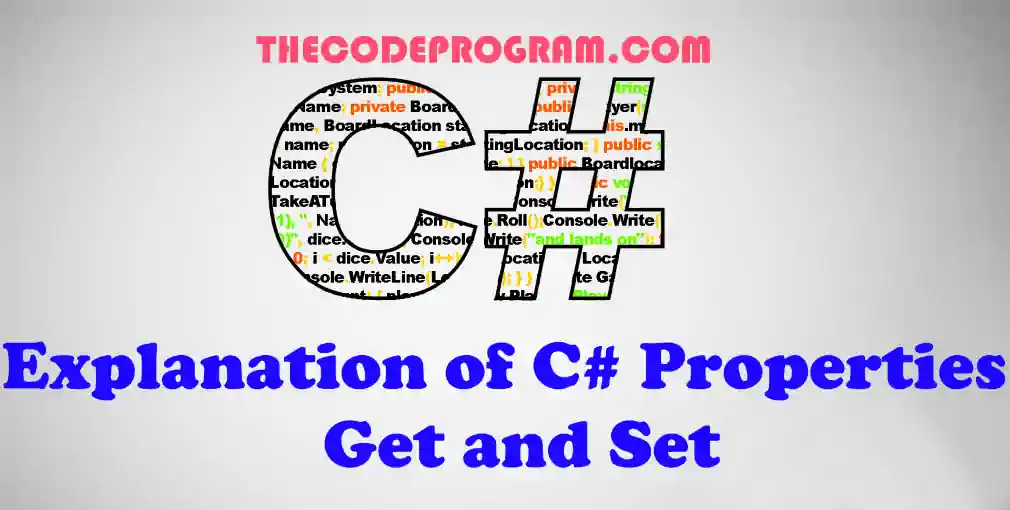
Explanation of C# Properties - Get and Set
Hello everyone, in this article we are going to talk about Properties specification of C# programming languages. I am sure you all hear get and set keywords. We will see what they are with simple examples.Let's get started.
When we create a class to derive some classes, we may declare some variables as private. And sometimes we may need to use these private variables at derived classes. In these cases we are going to use getters and setters as public properties. We can update a private variable through public property structure.
We generally need this specification while we use the Encapsulation. In our projects we generally create an upper class to derive some subclasses to write less code. At this point we may need to increase our code security so declare some variables as private. But also we may need to reach these classes in some subclasses. In here we can use Properties and GET/SET.
Below you can see a class with private variable and its property:
Aircraft.cs
namespace CSharp_Properties_Example
{
class Aircraft
{
//private variable
private string brand;
//public property of private variable
public string Brand
{
get { return this.brand; }
set { this.brand = value; }
}
}
}
In Here:
You can also create the property automatically. This method is a shortcut method in C#. All you need to write get; set; inside the property. You can see it at below code block.
Aircraft.cs
namespace CSharp_Properties_Example
{
class Aircraft
{
//private variable
private string brand;
//public property of private variable
public string Brand
{
get; set ;
}
}
}
Some important informations:
This specification lets you to improve code security and disabling to reach to unexpected variable and decreasing the confussion.
Also the intellisense will not let you to use the private variable. It will let you to use its property variable. Below image you can see it.
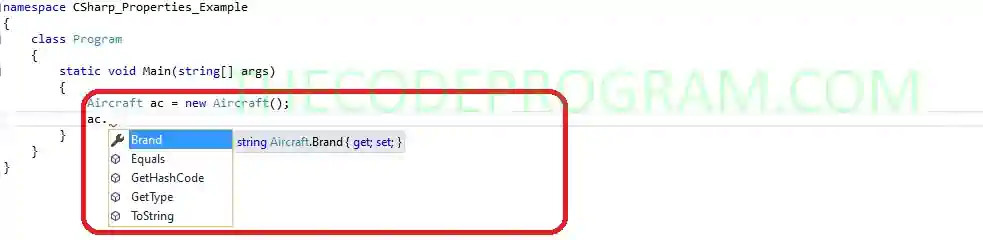
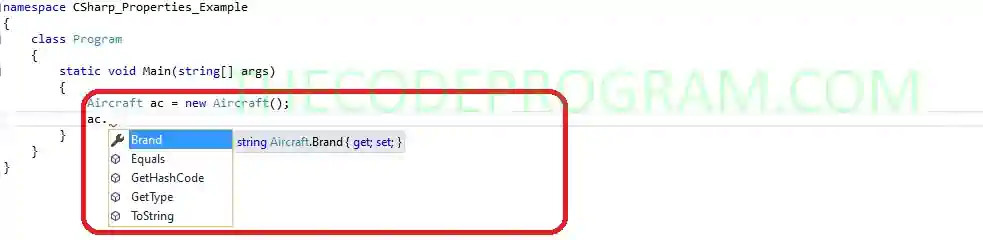
Let's give it a try :)
As you can see below code block will set a value first then read it via property.Program.cs
using System;
namespace CSharp_Properties_Example
{
class Program
{
static void Main(string[] args)
{
Aircraft ac = new Aircraft();
ac.Brand = "Boeing";
Console.WriteLine(ac.Brand);
Console.ReadLine();
}
}
}
That is all in this article.
Have a good gettings and settings.
Burak Hamdi TUFAN.
Tags
Share this Post
02/11/2021
QT C++ ile JSON Veri İşleme
18/02/2022
Comments